A Map
Map
Map
has a GraphicsEffects
GraphicsEffects
GraphicsEffects
object dedicated to map-wide graphics effects.
This article gives you an overview of the available effects and how you can use them in your application.
Ambient occlusion effect
Ambient occlusion
Ambient occlusion
Ambient occlusion
is an effect that mimics the natural phenomenon of shadows appearing on surfaces where objects are close to each other.
The difference between shadows and ambient occlusion is that ambient occlusion isn’t dependent on light sources.
It operates solely on the geometry of your dataset.
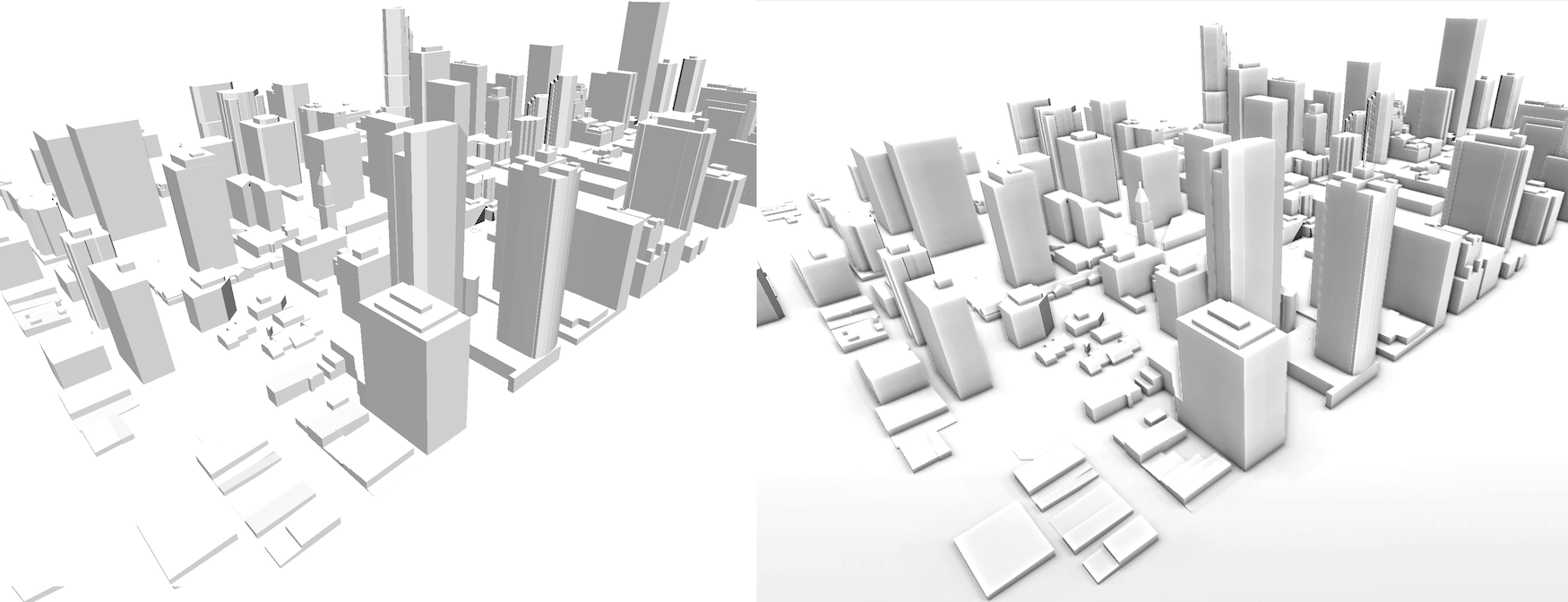
// get ambient occlusion effect from the map.
AmbientOcclusionEffect& ambientOcclusion = map->getEffects().getAmbientOcclusion();
// enable ambient occlusion effect.
ambientOcclusion.setEnabled(true);
// set ambient occlusion parameters individually.
ambientOcclusion.setRadius(28.0);
ambientOcclusion.setPower(0.8);
// set ambient occlusion parameters all at once.
ambientOcclusion.set(28.0, 0.8);
// get ambient occlusion effect from the map.
AmbientOcclusionEffect ambientOcclusion = map.Effects.AmbientOcclusion;
// enable ambient occlusion effect.
ambientOcclusion.IsEnabled = true;
// set ambient occlusion parameters individually.
ambientOcclusion.Radius = 28.0;
ambientOcclusion.Power = 0.8;
// set ambient occlusion parameters all at once.
ambientOcclusion.Set(28.0, 0.8);
// get ambient occlusion effect from the map.
AmbientOcclusionEffect ambientOcclusion = _map.getEffects().getAmbientOcclusion();
// enable ambient occlusion effect.
ambientOcclusion.setEnabled(true);
// set ambient occlusion parameters individually.
ambientOcclusion.setRadius(28.0);
ambientOcclusion.setPower(0.8);
// set ambient occlusion parameters all at once.
ambientOcclusion.set(28.0, 0.8);
You can use ambient occlusion to enhance your map with a greater sense of depth. It defines objects close to the ground more clearly. It’s highly recommended for data sets that were computer-generated, such as 3D CAD and BIM models.
You can control the radius and the power of the ambient occlusion effect.
LuciadCPillar supports the version of ambient occlusion called Screen Space Ambient Occlusion (SSAO). This implementation uses the GPU to calculate the effects of ambient occlusion on-the-fly based on what’s visible in your view. You can toggle it on and off at any time without extra overhead.
Ambient occlusion is recommended for cases in which 3D datasets with simple colors are visualized. For these types of datasets, ambient occlusion can help give the dataset more depth and make it easier to understand how geometry relates to each other. It also improves the visual quality of such datasets, and gives a better impression in general.
Ambient occlusion isn’t recommended for 3D datasets that were captured using real-life photography, such as reality meshes. Ambient occlusion already occurs naturally in the imagery of those datasets.
Ambient occlusion has a fixed performance overhead that depends on two factors:
-
The pixel resolution of your map
-
The GPU of the client
The size of your dataset doesn’t affect performance.
Ambient occlusion works on 3D maps only. It has no effect on 2D maps.
Eye-dome lighting effect
Eye-dome lighting
Eye-dome lighting
Eye-dome lighting
(EDL) is a non-photorealistic lighting model that accentuates the shapes of objects by shading their outlines.
Eye-dome lighting is similar to ambient occlusion.
It can give the dataset more depth and make it easier to understand how geometries relate to each other.
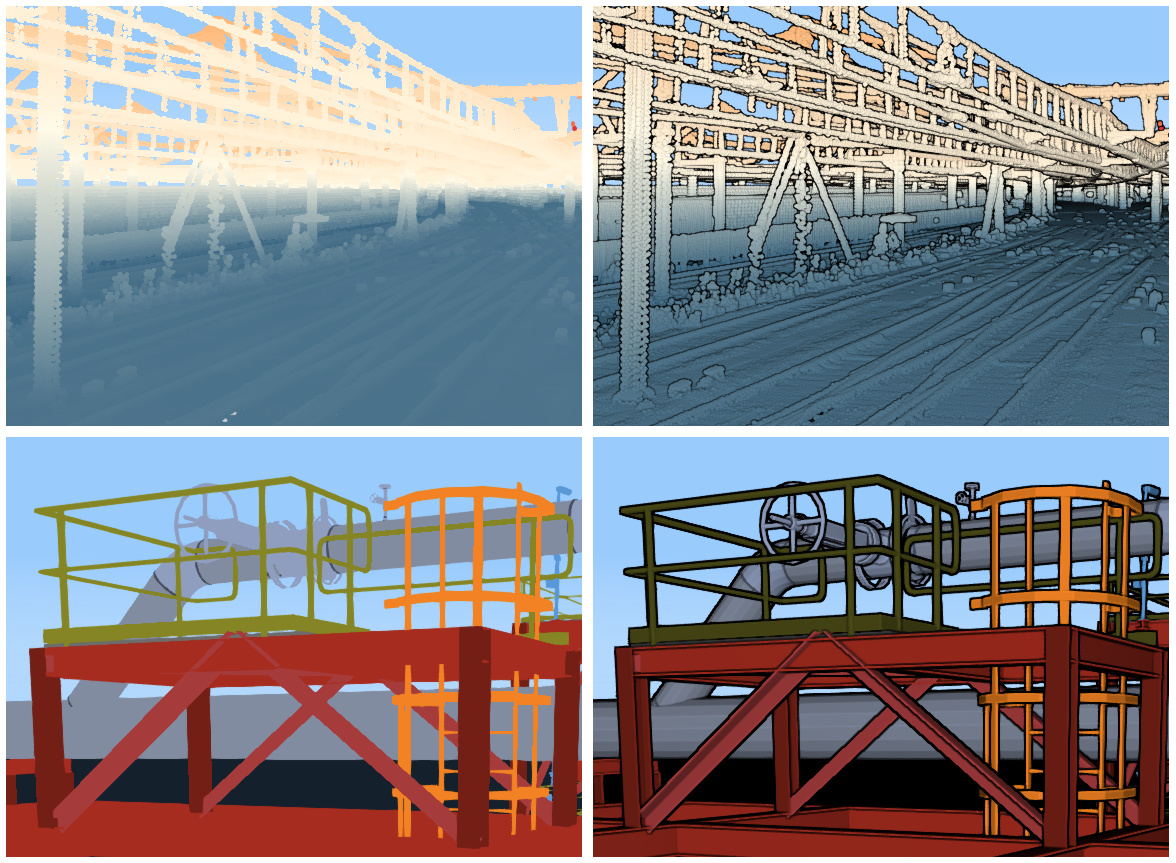
EDL can help to interpret datasets that have little or no visual variance. It’s useful for 3D datasets in particular, such as CAD and BIM models, or point clouds. Eye-dome lighting isn’t recommended for 3D datasets that were captured using real-life photography, such as reality meshes.
// get eye-dome lighting effect from the map.
EyeDomeLightingEffect& eyeDomeLighting = map->getEffects().getEyeDomeLighting();
// enable eye-dome lighting effect.
eyeDomeLighting.setEnabled(true);
// set eye-dome lighting parameters individually.
eyeDomeLighting.setWindow(1);
eyeDomeLighting.setStrength(0.5);
eyeDomeLighting.setColor(Color::blue());
// set eye-dome lighting parameters all at once.
eyeDomeLighting.set(1, 0.5, Color::blue());
// get eye-dome lighting effect from the map.
EyeDomeLightingEffect eyeDomeLighting = map.Effects.EyeDomeLighting;
// enable eye-dome lighting effect.
eyeDomeLighting.IsEnabled = true;
// set eye-dome lighting parameters individually.
eyeDomeLighting.Window = 1;
eyeDomeLighting.Strength = 0.5;
eyeDomeLighting.Color = Color.Blue;
// set eye-dome lighting parameters all at once.
eyeDomeLighting.Set(1, 0.5, Color.Blue);
// get eye-dome lighting effect from the map.
EyeDomeLightingEffect eyeDomeLighting = _map.getEffects().getEyeDomeLighting();
// enable eye-dome lighting effect.
eyeDomeLighting.setEnabled(true);
// set eye-dome lighting parameters individually.
eyeDomeLighting.setWindow(1);
eyeDomeLighting.setStrength(0.5);
eyeDomeLighting.setColor(Color.valueOf(Color.BLUE));
// set eye-dome lighting parameters all at once.
eyeDomeLighting.set(1, 0.5, Color.valueOf(Color.BLUE));
You can control the:
-
window: to increase the thickness of the applied EDL shade.
-
strength: to soften or harden the applied EDL shade.
-
color: to change the color of the applied EDL shade.
Technically, the lighting model applies a shade to each pixel, based on the depth difference between that pixel and its surrounding pixels. This implementation uses the GPU to calculate the effects on-the-fly based on what’s visible in your view. You can toggle it on and off at any time without extra overhead.
Eye-dome lighting has a fixed performance overhead that depends on two factors:
-
The pixel resolution of your map
-
The GPU of the client
The size of your dataset doesn’t affect performance.
Eye-dome lighting works on 3D maps only. It has no effect on 2D maps.
Atmosphere effect
You can use the atmosphere effect
atmosphere effect
atmosphere effect
to control how the sky looks like
on a 3D map. You can choose to disable the effect or change the sky appearance by changing the sky and horizon color.