Package com.luciad.maps.effects
Class EyeDomeLightingEffect
java.lang.Object
com.luciad.maps.effects.EyeDomeLightingEffect
- All Implemented Interfaces:
AutoCloseable
This class implements the Eye-dome lighting (EDL) effect, a non-photorealistic lighting model which helps accentuate the shapes of different objects to improve depth perception, by shading their outlines.
Left: eye-dome lighting disabled, Right: eye-dome lighting enabled
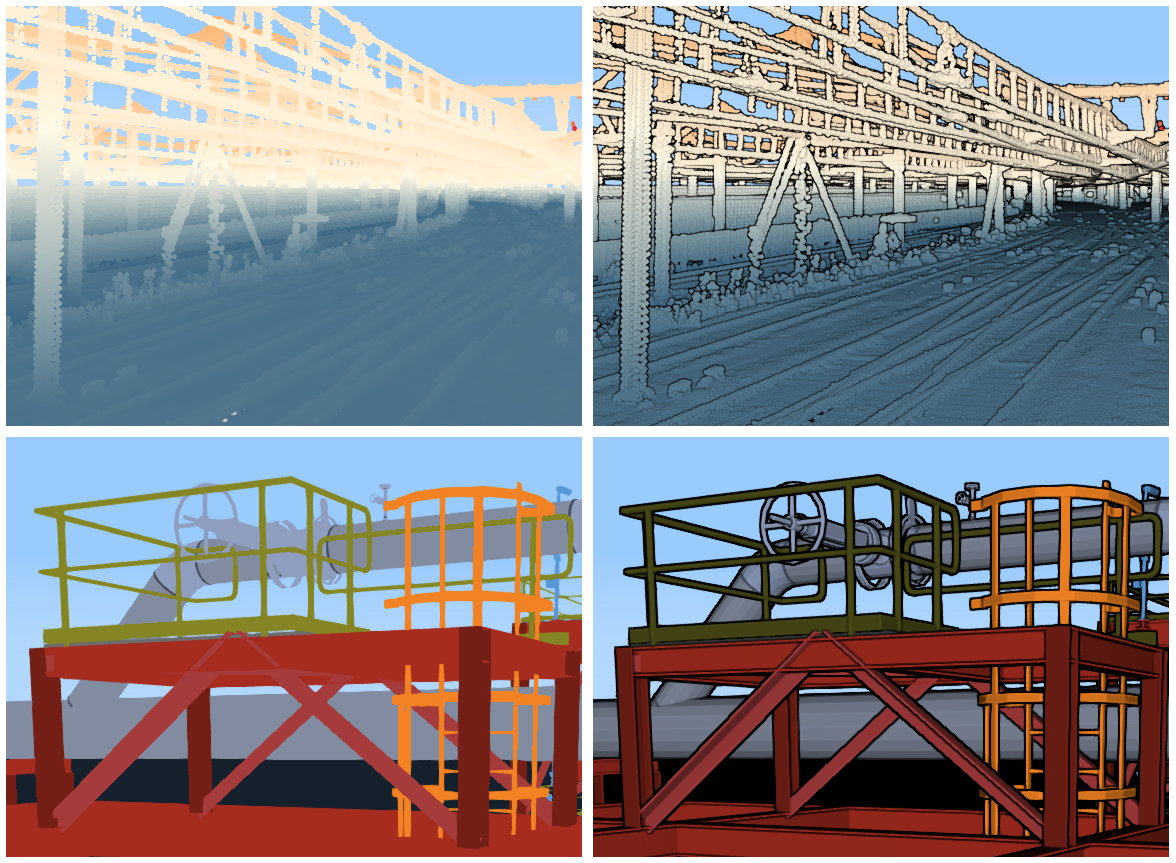
The lighting model applies a shade to each pixel, based on the depth difference between that pixel and its surrounding pixels. The EDL technique uses 3 properties:
- the window property defines how many surrounding pixels must be taken into account.
- the strength property is a factor to multiply with the shading that is applied to the image.
- the color property defines what color the shade will have.
Note that Eye-dome lighting is only applied in 3D views.
You cannot create an EyeDomeLightingEffect
. Instead, you can retrieve it from GraphicsEffects#getEyeDomeLighting
.
-
Method Summary
Modifier and TypeMethodDescriptionvoid
close()
protected void
finalize()
android.graphics.Color
getColor()
Returns the color of the shade.double
Returns the shading multiplication factor.int
Returns the number of surrounding pixels taken into account by EDL model.boolean
Returns whether the Eye-dome lighting effect is enabled.void
set
(int window, double strength, android.graphics.Color color) Sets multiple properties in one go.void
setColor
(android.graphics.Color color) Sets the color of the shade.void
setEnabled
(boolean enabled) Sets whether the Eye-dome lighting effect is enabled.void
setStrength
(double strength) Sets the shading multiplication factor.void
setWindow
(int window) Sets the number of surrounding pixels taken into account by EDL model.
-
Method Details
-
finalize
protected void finalize() -
close
public void close()- Specified by:
close
in interfaceAutoCloseable
-
isEnabled
public boolean isEnabled()Returns whether the Eye-dome lighting effect is enabled.- Returns:
- whether the Eye-dome lighting effect is enabled.
-
setEnabled
public void setEnabled(boolean enabled) Sets whether the Eye-dome lighting effect is enabled.The default is false.
- Parameters:
enabled
- true to enable the Eye-dome lighting effect or false to disable it.
-
getWindow
public int getWindow()Returns the number of surrounding pixels taken into account by EDL model.- Returns:
- the number of surrounding pixels taken into account by EDL model.
-
setWindow
public void setWindow(int window) Sets the number of surrounding pixels taken into account by EDL model.The default window is 2.
- Parameters:
window
- number of surrounding pixels taken into account by EDL model.
-
getColor
@NotNull public android.graphics.Color getColor()Returns the color of the shade.- Returns:
- the color of the shade.
-
setColor
public void setColor(@NotNull android.graphics.Color color) Sets the color of the shade.Note that the alpha component of the color is ignored so the color is fully opaque.
The default color is black.
- Parameters:
color
- color of the shade.
-
getStrength
public double getStrength()Returns the shading multiplication factor.- Returns:
- the shading multiplication factor.
-
setStrength
public void setStrength(double strength) Sets the shading multiplication factor.A value between 0 and 1 softens the shade, a value higher than 1 hardens the shade.
The default strength is 1.
- Parameters:
strength
- shading multiplication factor.
-
set
public void set(int window, double strength, @NotNull android.graphics.Color color) Sets multiple properties in one go.- Parameters:
window
- number of surrounding pixels taken into account by EDL model.strength
- color of the shade.color
- shading multiplication factor.- See Also:
-