Class AmbientOcclusionEffect
- All Implemented Interfaces:
AutoCloseable
This mimics a behavior of the real world where light gets trapped in tight areas. Typical examples are the corners of a room, which are slightly darker than the rest of the room as the light is trapped in the corners.
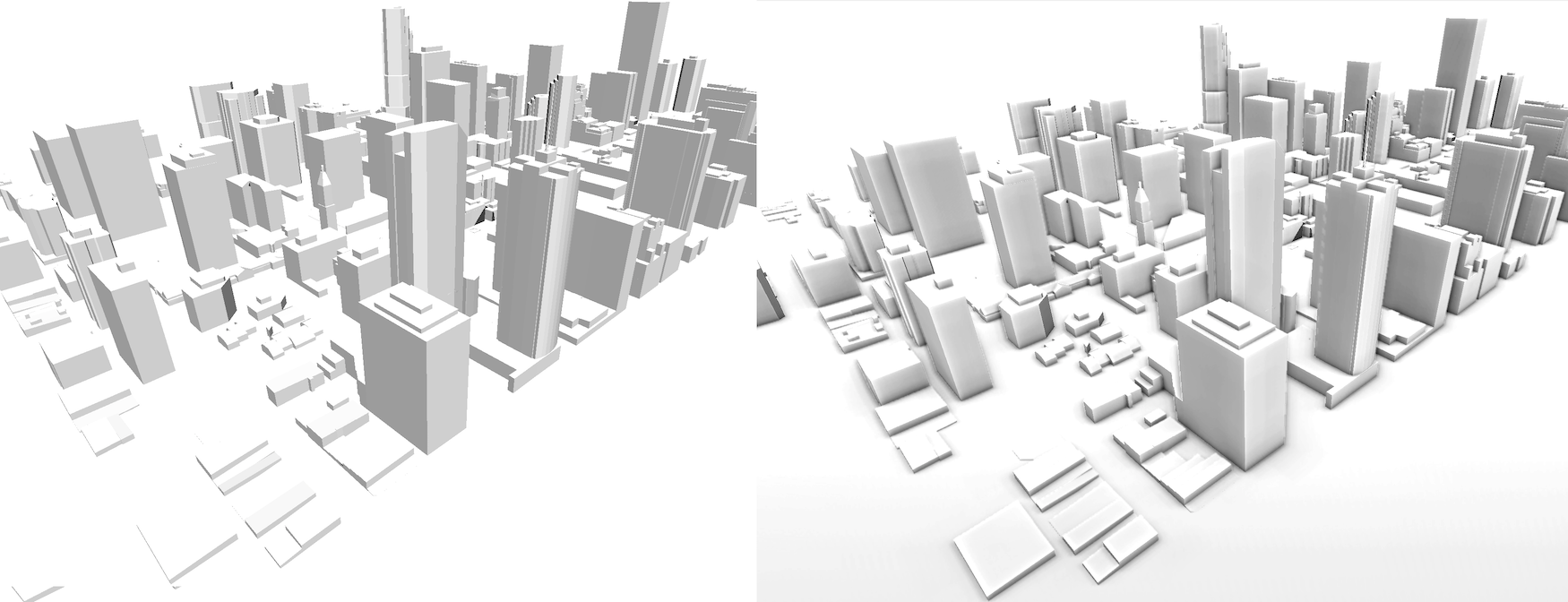
Ambient Occlusion is recommended for cases in which 3D datasets with simple colors, such as 3D CAD & BIM models, are visualized. For these types of datasets, Ambient Occlusion can help give the dataset more depth and make it easier to understand how geometry relates to each other. On top of that, it also improves the visual quality of such datasets, and gives an overall better impression.
Note that Ambient Occlusion is only applied in 3D views.
You cannot create an AmbientOcclusionEffect
. Instead, you can retrieve it from GraphicsEffects#getAmbientOcclusion
.
-
Method Summary
Modifier and TypeMethodDescriptionvoid
close()
protected void
finalize()
double
getPower()
Returns the power factor which determines how strongly the effect is applied to the view.double
Returns the radius in meters at which the effect is sampled.boolean
Returns whether the Ambient Occlusion effect is enabled.void
set
(double radius, double power) Sets the radius and power values for the ambient occlusion effect.void
setEnabled
(boolean enabled) Sets whether the Ambient Occlusion effect is enabled.void
setPower
(double power) Sets the power factor which determines how strongly the effect is applied to the view.void
setRadius
(double radius) Sets the radius in meters at which the effect is sampled.
-
Method Details
-
finalize
protected void finalize() -
close
public void close()- Specified by:
close
in interfaceAutoCloseable
-
isEnabled
public boolean isEnabled()Returns whether the Ambient Occlusion effect is enabled.- Returns:
- whether the Ambient Occlusion effect is enabled.
-
setEnabled
public void setEnabled(boolean enabled) Sets whether the Ambient Occlusion effect is enabled.The default is false.
- Parameters:
enabled
- true to enable the Ambient Occlusion effect or false to disable it.
-
getRadius
public double getRadius()Returns the radius in meters at which the effect is sampled.- Returns:
- the radius in meters at which the effect is sampled.
-
setRadius
public void setRadius(double radius) Sets the radius in meters at which the effect is sampled.A lower value produces sharper results, while higher values produce softer results.
The default radius is 30m.
- Parameters:
radius
- radius in meters at which the effect should be sampled.
-
getPower
public double getPower()Returns the power factor which determines how strongly the effect is applied to the view.- Returns:
- the power factor which determines how strongly the effect is applied to the view.
-
setPower
public void setPower(double power) Sets the power factor which determines how strongly the effect is applied to the view.The power factor determines how much to brighten or darken the effect.
Values should be a positive floating point number, generally between 0 and 5. Higher values are accepted, but not recommended.
The default power value is 1.
- Parameters:
power
- factor to brighten or darken the effect.
-
set
public void set(double radius, double power) Sets the radius and power values for the ambient occlusion effect.- Parameters:
radius
- radius in meters at which the effect should be sampled.power
- a factor to brighten or darken the effect.- See Also:
-