This guide explains how you can interactively edit features on a Map, and integrate this capability in an
IController
IController
IController
.
Overview
The CPillar Editor
Editor
Editor
translates input events
input events
input events
to edit actions that are applied to a configured
configured
configured
selection
of features. For these features, the editor retrieves
retrieves
retrieves
and
visualizes
visualizes
visualizes
a set of handles
handles
handles
.
The handles are linked to edit actions that perform the actual feature modification
feature modification
feature modification
.
Programmatically creating geometries
link: If you want to edit geometries through the API instead of editing them interactively on the map, see the related article on geometries. |
Editing Features and Geometries
Base concepts
To understand how features or geometries are edited, a few concepts need to be explained.
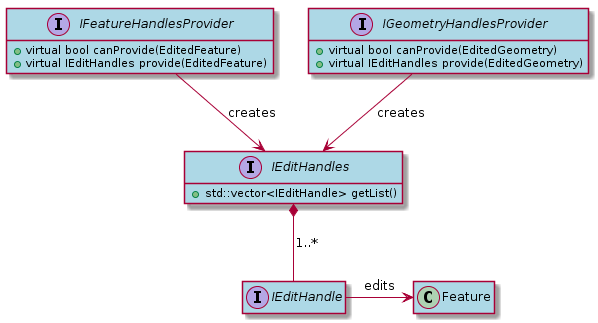
Observable feature and geometries
To make it easier to observe the changes that are happening to an edited feature or geometry, the
Observable<T>
Observable<T>
Observable<T>
class is used in the edit API:
This class stores a Feature or Geometry that can change during editing. It allows you to get the current state, and observe changes to the Feature or Geometry. This for example allows you to update the handles of an object that is being edited.
IEditHandle
IEditHandle
IEditHandle
IEditHandle
is a visual
visual
visual
component on the map that triggers concrete edit actions based on input events
input events
input events
.
An example is a point handle that is visualized as an icon, and that you can drag around, click on, or touch press.
An edit handle triggers a change to the feature or geometry by notifying that it has changed. A point handle will for example
send a notification that it has moved. Based on that notification, the IEditHandles
IEditHandles
IEditHandles
can modify a feature or geometry.
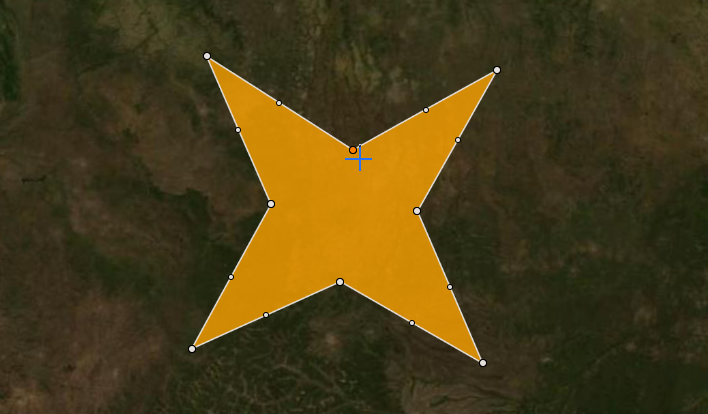
Example IEditHandle
IEditHandle
IEditHandle
implementations are:
-
PointEditHandle
PointEditHandle
PointEditHandle
: This handle is visualized by a point icon. It allows you to drag it around, click, or touch press it. It usesIPointEditAction
IPointEditAction
IPointEditAction
to signal that an action is performed. -
TranslateEditHandle
TranslateEditHandle
TranslateEditHandle
: This handle is either not visualized, or visualized by ashadow geometry
shadow geometry
shadow geometry
. It allows you to drag it around, and translate an entire geometry. It usesITranslateEditAction
ITranslateEditAction
ITranslateEditAction
to signal that an action is performed.
IEditHandles
IEditHandles
IEditHandles
IEditHandles
is a collection of IEditHandle
IEditHandle
IEditHandle
instances that is created
for a specific feature or geometry.
This collection must update its own handles whenever the edited object changes. The edited object can change in two cases:
-
By an edit action performed by one of its handles.
-
By an
external change
external change
external change
to the feature or geometry, for example originating from anIFeatureModel
IFeatureModel
IFeatureModel
event
In both cases, the observable
observable
observable
feature
feature
feature
or geometry
geometry
geometry
will notify the IEditHandles
IEditHandles
IEditHandles
that it has changed.
Triggered by this notification, IEditHandles
IEditHandles
IEditHandles
can update its list of handles.
IEditHandles provider
IFeatureHandlesProvider
IFeatureHandlesProvider
IFeatureHandlesProvider
and
IGeometryHandlesProvider
IGeometryHandlesProvider
IGeometryHandlesProvider
can create an IEditHandles
IEditHandles
IEditHandles
instance
for a given feature or geometry. These classes are typically stateless, and can be reused for more than one feature or
geometry.
Example IGeometryHandlesProvider
IGeometryHandlesProvider
IGeometryHandlesProvider
implementations:
-
PointHandlesProvider
PointHandlesProvider
PointHandlesProvider
: provides handles to edit Point geometries -
PolylineHandlesProvider
PolylineHandlesProvider
PolylineHandlesProvider
: provides handles to edit Polyline geometries -
PolylineRingHandlesProvider
PolylineRingHandlesProvider
PolylineRingHandlesProvider
: provides handles to edit PolylineRing geometries -
PatchHandlesProvider
PatchHandlesProvider
PatchHandlesProvider
: provides handles to edit patches. This class doesn’t provide any handles itself, but it asks anotherIGeometryHandlesProvider
IGeometryHandlesProvider
IGeometryHandlesProvider
to provide handles for its base geometry, for example aPolylineRingHandlesProvider
PolylineRingHandlesProvider
PolylineRingHandlesProvider
. -
PolygonHandlesProvider
PolygonHandlesProvider
PolygonHandlesProvider
: provides handles to edit polygons. This class doesn’t provide any handles itself, but it asks anotherIGeometryHandlesProvider
IGeometryHandlesProvider
IGeometryHandlesProvider
to provide handles for its exterior and interior rings, for example aPolylineRingHandlesProvider
PolylineRingHandlesProvider
PolylineRingHandlesProvider
.
Example IFeatureHandlesProvider
IFeatureHandlesProvider
IFeatureHandlesProvider
implementations:
-
FeatureHandlesProvider
FeatureHandlesProvider
FeatureHandlesProvider
: provides handles to edit a Feature byextracting
extracting
extracting
a geometry from the feature and using anIGeometryHandlesProvider
IGeometryHandlesProvider
IGeometryHandlesProvider
to provide handles for that geometry. After the geometry is modified, it is used to create modified Feature.
Editor
Editor
Editor
Editor
determines which features are edited, and delegates its
input events
input events
input events
to the edit handles
edit handles
edit handles
for that feature. It also provides a layer
provides a layer
provides a layer
that can
visualize the used handles on the Map.
Handle interactions
The following diagram shows the most common interactions between the different concepts:
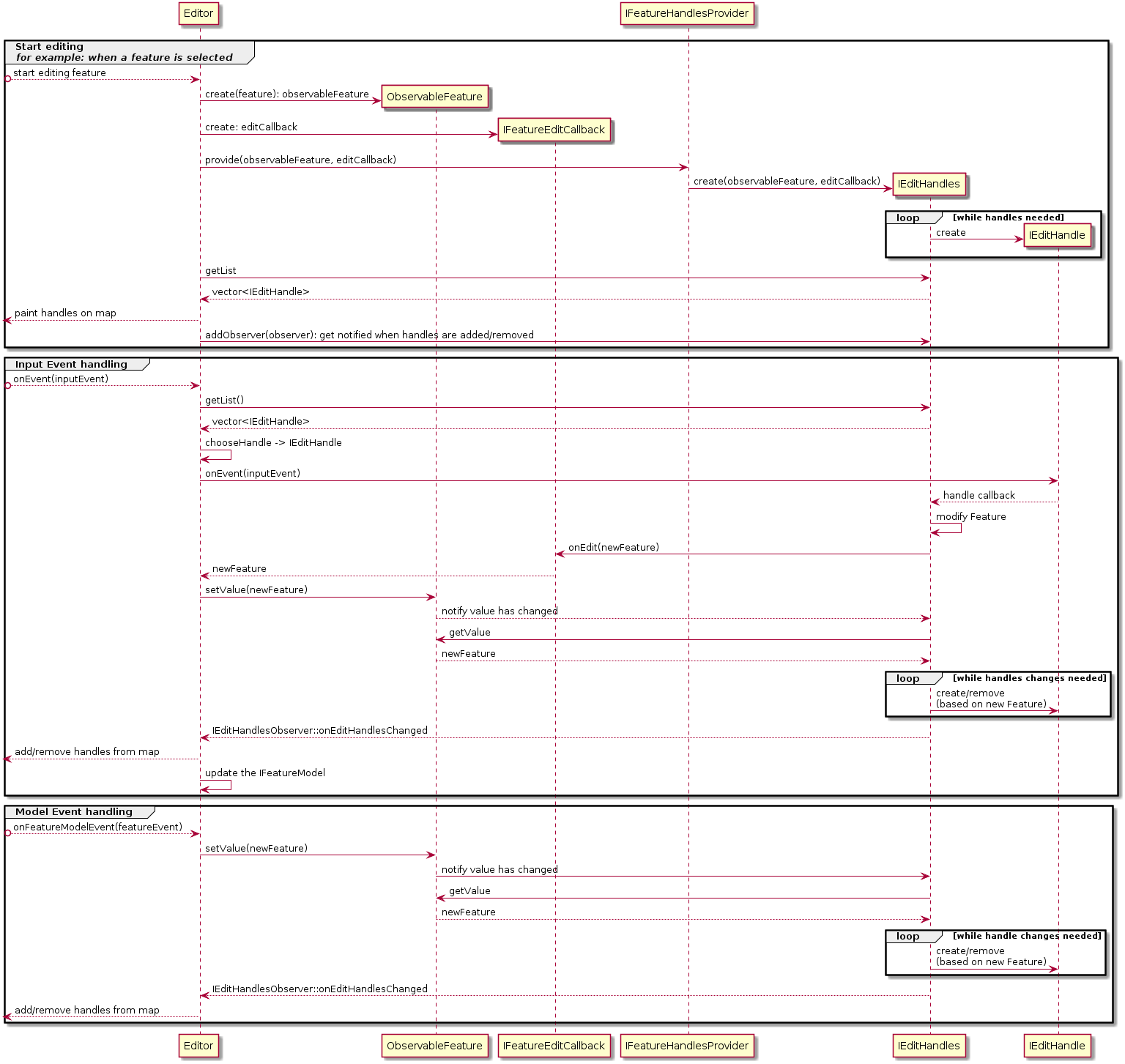
Handle interaction is wired together using callbacks and observers:
-
IEditHandle
IEditHandle
IEditHandle
implementations typically use an action to notifyIEditHandles
IEditHandles
IEditHandles
that they have been modified. Based on this callback, theIEditHandles
IEditHandles
IEditHandles
implementation can modify the feature or geometry. An example isPointEditHandle
PointEditHandle
PointEditHandle
which usesIPointEditAction
IPointEditAction
IPointEditAction
to communicate that the handle has been interacted with. -
IFeatureEditCallback
IFeatureEditCallback
IFeatureEditCallback
orIGeometryEditCallback
IGeometryEditCallback
IGeometryEditCallback
notifyEditor
Editor
Editor
or otherIEditHandles
IEditHandles
IEditHandles
that the feature or geometry has been modified. -
Observable
Observable
Observable
of Feature and Geometry send notifications when a feature or geometry has been modified. This allows theIEditHandles
IEditHandles
IEditHandles
to update its handles. The change can be:-
external change: for example based on an event originating from
IFeatureModel
IFeatureModel
IFeatureModel
-
editing changes: triggered by
Editor
Editor
Editor
when receiving a callback fromIFeatureEditCallback
IFeatureEditCallback
IFeatureEditCallback
orIGeometryEditCallback
IGeometryEditCallback
IGeometryEditCallback
-
Configuring feature editing
To configure feature editing, you can use these classes:
-
Editor::Builder
: toconfigure the edit candidates
configure the edit candidates
configure the edit candidates
used. -
FeatureLayer::Builder
: toenable or disable
enable or disable
enable or disable
feature editing for a layer and to define anedit configuration
edit configuration
edit configuration
. With this edit configuration, you can:-
Configure the handles
Configure the handles
Configure the handles
used to edit a Feature. -
Configure
Configure
Configure
when theIFeatureModel
IFeatureModel
IFeatureModel
is updated by the Editor. -
Provide the geometry
Provide the geometry
Provide the geometry
used for editing a a Feature, and specify how to apply edited geometries to the Feature. This provider is mainly used by the defaultfeature handles provider
feature handles provider
feature handles provider
.
-
Constraining geometries during editing
The editing API has out of the box support for geometry constraints. Most
IGeometryHandlesProvider
IGeometryHandlesProvider
IGeometryHandlesProvider
implementations allow you to configure
a constraint that is applied during editing. See the related article
for more information.
Integrating an editor with a controller
You can use the Editor
Editor
Editor
class as a part of an IController
IController
IController
implementation. To integrate the feature
editor:
-
Delegate
input events
input events
input events
. The editor may or may not consume those events. Based on that information, the controller can decide to ignore the event, or use it for something else. For example, an editor can ignore mouse or touch events if there is no Feature at the position of those events. The controller can still use these events to navigate the map, though. -
Add the
handle layer
handle layer
handle layer
to the controller’slayer list
layer list
layer list
. You need to do that so that the map can visualize the edit handles. -
Change the
mouse cursor
mouse cursor
mouse cursor
at theappropriate moment
appropriate moment
appropriate moment
.
Examples of customizations
The following articles and tutorials give examples of how you can customize the edit behavior:
-
This tutorial shows you how to customize existing handles provider.
-
This tutorial shows you how you can extra handles to an existing handles provider.
-
This tutorial shows how you can implement your own handles provider.