Class PerspectiveCamera
- All Implemented Interfaces:
AutoCloseable
Also known as a pinhole camera.
The Camera
API is a low-level API. For simple map navigation use cases, it's recommended to use the higher-level API. The MapNavigator
API works in both 2D and 3D, on all map references. If you use the low-level Camera
API, keep in mind that the same camera manipulation implementation might not work for other types of cameras (OrthographicCamera
vs PerspectiveCamera
) or other (types of) map references (projected vs. geocentric).
A camera transforms points from the map reference
to the view (device independent pixel coordinates).
Besides the position, orientation and size properties that it inherits from Camera
, the perspective camera defines its projection using a field of view
: the angle, in degrees, that determines the vertical viewing angle of the camera. The horizontal field-of-view angle can be derived from the vertical field-of-view and the camera's aspect ratio.
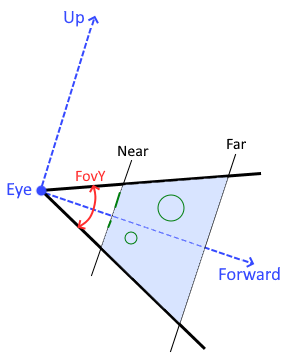
Cameras in LuciadCPillar are immutable. You can manipulate the map's camera by creating a modified copy using the builder
. The new camera can then be set
on the map.
Currently, the PerspectiveCamera
is only used in combination with geocentric (3D) map references (aka 3D maps). For now, you cannot use this camera if the map has a projected (grid) reference.
To manipulate a perspective camera in a geocentric reference, consider using lookAt
or lookFrom
. This allows you to reason about the camera in terms of yaw (angle from north direction), pitch (angle wrt. horizon) and roll. You could also just manipulate the camera's eye position and forward/up directions directly, if that's a better fit for your use case.
Map
navigation constraints configured on MapNavigator
are not enforced when this camera is updated on the map. Constraints are only respected when using MapNavigator
.
Example:
// low-level manipulation of perspective camera: put camera looking over Europe, faced towards the north var perspectiveCamera = (PerspectiveCamera) map.getCamera(); Camera.Look newCameraPosition = new Camera.Look( new Coordinate(6214861.581912037, 710226.7751339739, 4927634.769711619), new Coordinate(0.9588725076044676, -0.12713997961061635, -0.25376946180526144), new Coordinate(-0.2469197372006221, -0.06727846255744102, 0.9666976010400992) ); Camera newCamera = perspectiveCamera.asBuilder().look(newCameraPosition).build(); // ... // Set the camera on the map, for example by using Map#getAnimationManager map.setCamera(newCamera);
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic final class
Factory object that can create newPerspectiveCamera
instances.Nested classes/interfaces inherited from class com.luciad.cameras.Camera
Camera.Look, Camera.Look2D, Camera.LookAt, Camera.LookFrom
-
Method Summary
Modifier and TypeMethodDescriptionReturns a Builder that is initially configured to build cameras identical to this camera.asLook()
Returns the position and orientation of the camera.asLookAt
(double distance) Returns aLookAt
that matches the position and orientation of this camera.Returns aLookFrom
that matches the position and orientation of this camera.void
close()
protected void
finalize()
getFovY()
Returns the vertical field of view of the camera.
-
Method Details
-
finalize
protected void finalize() -
close
public void close()- Specified by:
close
in interfaceAutoCloseable
- Overrides:
close
in classCamera
-
asBuilder
Returns a Builder that is initially configured to build cameras identical to this camera.You can then change a subset of the camera properties using this builder to create a new camera.
- Returns:
- a Builder that is initially configured to build cameras identical to this camera.
-
getFovY
Returns the vertical field of view of the camera.- Returns:
- the vertical field of view of the camera.
- See Also:
-
asLook
Returns the position and orientation of the camera.- Returns:
- the position and orientation of the camera.
-
asLookAt
Returns aLookAt
that matches the position and orientation of this camera.- Returns:
- a
LookAt
that matches the position and orientation of this camera.
-
asLookFrom
Returns aLookFrom
that matches the position and orientation of this camera.- Returns:
- a
LookFrom
that matches the position and orientation of this camera.
-