In the TileSet3DLayer
, you can perform selection operations on the layer.
For a TileSet3DLayer
to have selectable parts, you must:
-
Set the
TileSet3DLayer.selectable
property to true. -
Set the
TileSet3DLayer.idProperty
at construction time.
With the idProperty
of a TileSet3DLayer, you refer to a property name in the metadata of the tileset.
For OGC 3D Tiles, the metadata is part of the batch table.
The idProperty
is often specific to the domain and the data, so you can’t generalize it for all use cases.
The figure Figure 1, “An example of a simple dataset with metadata.” shows a simple example of 4 cubes and their metadata property names and values.
Note that in this example, all cubes are part of the same TileSet3DLayer
dataset:

If you used the GUID
property in this dataset, and applied a selection to all data with GUID
equal to 1, you’d get the following result:
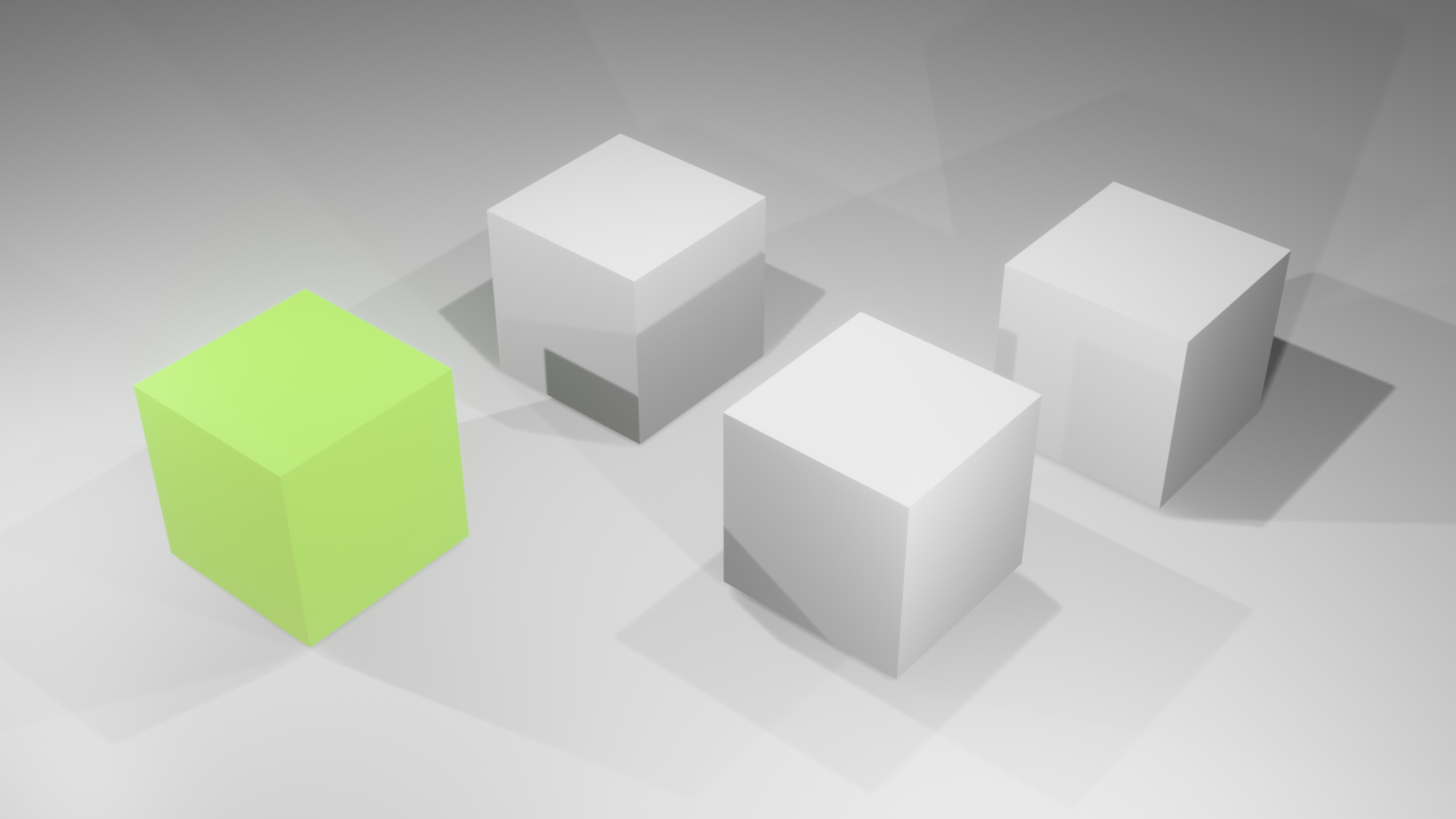
GUID
set as the idProperty
. The cube with GUID
equal to 1 is selected.
The idProperty
doesn’t have to be unique in the dataset.
You can choose any existing metadata field name in your dataset for your idProperty
.
If you set the idProperty
to the MaterialID
property in the example, and clicked on a cube with MaterialID=1, you would select all cubes with MaterialID=1:
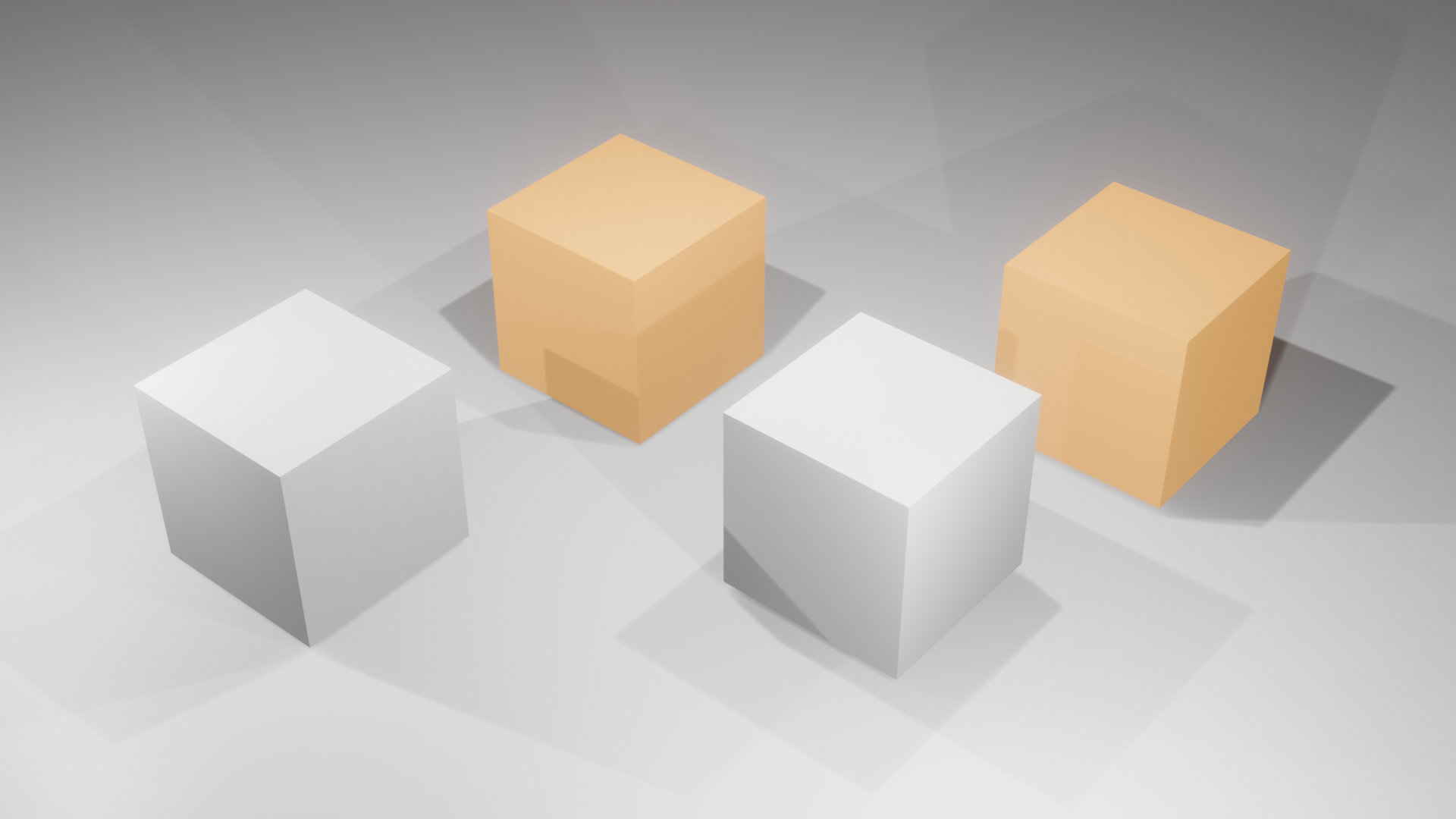
MaterialID
selected as the idProperty
. The user clicked on one of the cubes with MaterialID=1.
To create a new TileSet3DLayer
with an idProperty
of GUID
, you can use:
const ogc3dTilesLayer = new TileSet3DLayer(model, {
selectable: true,
idProperty: "GUID" // Must be a property name that also exists in the metadata of the TileSet3DLayer
});
The list of metadata properties that you can use as |
Once you have your selectable TileSet3DLayer
up and running, you can start listening to SelectionChanged
events.
You listen to those events in the same way as you listen to selection changes in a FeatureLayer
.
See Selecting and deselecting objects on the map through the API.
Like in a FeatureLayer
, a SelectionChanged
event has a set of Feature
objects that are part of the current selection in your TileSet3DLayer
.
Although TileSet3DLayer
doesn’t explicitly Feature
objects, the interface is used to convey properties about the selected 3D mesh data.
The Feature
objects returned by the SelectionChanged
event contain this information:
-
The
id
is the value of the selectedidProperty
-
The
shape
is aPoint
with the map coordinates indicating where the mouse interacted with theTileSet3DLayer
-
The
properties
contain all metadata properties about the selected feature.
Handling selection without an idProperty
If your dataset doesn’t contain any metadata, you can’t perform selection operations, not even if you enable TileSet3DLayer.selectable
.
It’s possible, though, to use the Map.pickAt()
function to find out if a view- coordinate touches a TileSet3DLayer
.
In this scenario, the Map.pickAt()
function returns a reference to the TileSet3DLayer
, but it contains a Feature
with its id
set to the string "unknown"
and its properties
undefined.
This indicates that the mouse interacts with the view-coordinate at the specific pixel location, but that you can’t actually
select it.
For these cases, LuciadRIA doesn’t fire SelectionChanged
events, because the selection on the map didn’t actually change.
Changing the styling of the selection
By default, selecting a Feature
in a TileSet3DLayer
changes its color on the map.
The default selection color is orange.
To change the default color, you can override the MeshStyle.selectedColorExpression
.
LuciadRIA applies the expression to any part of your mesh where the idProperty
attribute has the selected property value as true
.
This expression is available for reasons of convenience.
To prevent selection from changing the color of your TileSet3DLayer
, set it to null
.
It’s also possible to write a more complex expression in MeshStyle.colorExpression
to further customize selection styling.
You typically won’t need this option, though.
Programmatic selection and its limits
To perform programmatic selection operations on the map, use the Map.selectObjects()
function.
See Selecting and deselecting objects on the map through the API for more details.
The interfaces for a TileSet3DLayer
and a FeatureLayer
are the same, with the exception that a TileSet3DLayer
doesn’t actually contain a set of Feature
objects.
It’s possible to create Feature
objects as needed.
The only condition is that you set their Feature.id
property to the desired property value.
Programmatic selection has one limitation. If you programmatically select a feature that you didn’t select before, the resulting
SelectionChanged
event won’t have any metadata in its feature properties.
You can select many objects at the same time in a TileSet3DLayer
, but keep in mind that the number of simultaneously selected objects is subject to hardware limitations. If you select thousands
of objects, you could trigger an overflow of the internal WebGL shader code.
It’s recommended to select only a few objects in one go.