Class TLcdGXYRoundedPointListPainter
- All Implemented Interfaces:
ILcdCloneable
,ILcdPropertyChangeSource
,ILcdGXYEditor
,ILcdGXYEditorProvider
,ILcdGXYPainter
,ILcdGXYPainterProvider
,ILcdGXYPathPainter
,Serializable
,Cloneable
Supported shapes
This painter supportsILcdPointList
, TLcdRoundedPolyline
, and TLcdRoundedPolygon
objects.
Rounding
A spline algorithm based on the construction of Bezier curves is used to calculate the rounded corners. The resulting spline does not pass through the points of the point list, except for the begin and end point whenTLcdGXYPointListPainter.POLYLINE
mode is used. The amount of roundness used for the
corners can be controlled through the method setRoundness(double)
.
Note that the spline is calculated in world coordinates, so the rendering can vary for different
geographic projections.
Painting an ILcdPointList
Body
The same five paint modes as in TLcdGXYPointListPainter are provided by this painter, which allow the point list to be painted as:
![]() |
|
![]() |
||
A set of points (TLcdGXYPointListPainter.POINT ) |
|
A polyline (TLcdGXYPointListPainter.POLYLINE ) |
||
|
||||
![]() |
|
![]() |
|
![]() |
An outlined polygon (TLcdGXYPointListPainter.POLYGON ) |
|
A filled polygon (TLcdGXYPointListPainter.FILLED ) |
|
A filled and outlined polygon (TLcdGXYPointListPainter.OUTLINED_FILLED ) |
Objects that are selected or that are being edited, are painted as defined by the selectionMode
and editMode properties, respectively. The same values as above can be used for both mode
properties. These properties are not taken into account when an object needs to
be painted in the paint mode POINT
or POLYLINE
.
Handles
Every point of the source point list is regarded as a handle of the point list. The following image clarifies the handle location, the points are represented by red points.
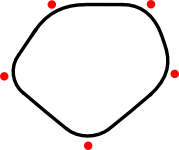
Note that, when the paint mode is set to
POINT
, the points are more than only a
handle. They are also the only visible part of the shape and should therefore always be painted
independent of the render mode.
Snap targets
Every point of the source point list can be returned on the condition that it is touched (see
getTouchedPoint
) and that
the roundness set on this painter (see getRoundness()
) equals 0. The point returned as
a snap target is highlighted with the snapIcon
.
Styling options
The visualization of the ILcdPointList
object is governed by the line style and fill
style set to this painter. Depending on the paint mode chosen one or both of these will be taken
into account.
Locating an ILcdPointList in a view
Anchor point of an ILcdPointList
When the ILcdPointList
is also an ILcdShape
, the anchor point is located at
the focus point of the shape. Otherwise the anchor point is located at the center point of the source
point list.
When is an ILcdPointList touched
Depending on the paint mode, the object is touched when either
the painted point list, or the internal area of the painted point list is touched.
The methods
getTouchedPoint
,
getTouchedSegment(java.awt.Graphics, int, com.luciad.view.gxy.ILcdGXYContext)
getTouchedSegment}
and
insidePolygon
provide information
on which part of the object is being touched.
Visually editing and creating an ILcd2DEditablePointList
Additional properties
- Since:
- 8.0
- See Also:
-
Field Summary
Fields inherited from class com.luciad.view.gxy.painter.TLcdGXYPointListPainter
AREA, COORDINATES_AVERAGE, END_OF_CURVE, FILLED, FIRST_POSSIBLE_POINT, FOCUS_POINT, INSIDE_POLYGON, MEDIAN_POINT, MIDDLE_OF_BOUNDS, MIDDLE_OF_CURVE, OUTLINE_AREA, OUTLINED_FILLED, POINT, POLYGON, POLYLINE, START_OF_CURVE
Fields inherited from class com.luciad.view.gxy.painter.ALcdGXYAreaPainter
OUTLINED
Fields inherited from class com.luciad.view.gxy.ALcdGXYPainter
defaultCreationFillStyle, defaultCreationLineStyle, defaultFillStyle, defaultLineStyle, fWorkBounds
Fields inherited from interface com.luciad.view.gxy.ILcdGXYEditor
CREATING, END_CREATION, RESHAPED, START_CREATION, TRANSLATED
Fields inherited from interface com.luciad.view.gxy.ILcdGXYPainter
BODY, CREATING, DEFAULT, HANDLES, RESHAPING, SELECTED, SNAPS, TRANSLATING
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs a defaultTLcdGXYRoundedPointListPainter
in a POLYLINE mode and with display name "Polypoint".TLcdGXYRoundedPointListPainter
(int aPointListMode) Constructs aTLcdGXYRoundedPointListPainter
in the given mode and with display name "Polypoint".TLcdGXYRoundedPointListPainter
(String aDisplayName) Constructs a defaultTLcdGXYRoundedPointListPainter
in a POLYLINE mode and with display nameaDisplayName
.TLcdGXYRoundedPointListPainter
(String aDisplayName, int aPointListMode) Constructs aTLcdGXYRoundedPointListPainter
in the given mode and with display nameaDisplayName
. -
Method Summary
Modifier and TypeMethodDescriptionvoid
boundsSFCT
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) Sets the supplied bounds (in view coordinates, pixels) so that it encompasses the representation of the object and its handles (the source point list) in the given mode taking into account the given context.clone()
MakesObject.clone()
public.Returns the object that can currently be painted or edited by this painter/editor.double
Returns the roundness factor that is used to render corners of polygons or polylines.int
getTouchedSegment
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Returns the index of the segment in theILcdPointList
object that has been touched according to the coordinates in the context, or-1
if none of the segments were touched.boolean
isTouched
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Depending on the render mode and paint mode, returns whether one of the handles (the source point list), the painted point list or the interior of the point list is touched.protected ILcdPointList
retrievePointList
(Object aObject) Extracts anILcdPointList
from the given object.void
Sets the object to paint or edit.void
setRoundness
(double aRoundness) Sets the roundness factor that should be used to render corners of polygons or polylines.boolean
supportSnap
(Graphics aGraphics, ILcdGXYContext aGXYContext) Supports snapping when theILcdPointList
object set to this painter contains at least one point andgetRoundness()
equals 0.Methods inherited from class com.luciad.view.gxy.painter.TLcdGXYPointListPainter
acceptSnapTarget, anchorPointSFCT, append2DPoint, appendAWTPath, appendGeneralPath, edit, getAnchorPointLocation, getCreationClickCount, getCursor, getFillSelection, getGXYEditor, getMinimumEditDelta, getMode, getModeName, getPaintCache, getSnapIcon, getTouchedPoint, getTouchedSegment, getWindingRule, insert2DPoint, insidePolygon, isMoveOnlyOnEdit, isTraceOn, linkToSnapTarget, move2DPoint, paint, removePointAt, retrieveMinimalPointcount, setAnchorPointLocation, setFillSelection, setMinimumEditDelta, setMode, setModelModelTransformationClass, setMoveOnlyOnEdit, setPaintCache, setSnapIcon, setTraceOn, setupGraphicsForFill, setupGraphicsForLine, setupGraphicsForPoint, setWindingRule, snapTarget, translate2D, translate2DPoint
Methods inherited from class com.luciad.view.gxy.painter.ALcdGXYAreaPainter
getEditMode, getFillStyle, getLineStyle, getSelectionMode, setEditMode, setFillStyle, setLineStyle, setSelectionMode
Methods inherited from class com.luciad.view.gxy.ALcdGXYPainter
addPropertyChangeListener, firePropertyChangeEvent, firePropertyChangeEvent, getDisplayName, getGXYPainter, removePropertyChangeListener, setClassTraceOn, setDisplayName
Methods inherited from class java.lang.Object
equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface com.luciad.view.gxy.ILcdGXYEditor
getDisplayName
Methods inherited from interface com.luciad.view.gxy.ILcdGXYPainter
getDisplayName
Methods inherited from interface com.luciad.util.ILcdPropertyChangeSource
addPropertyChangeListener, removePropertyChangeListener
-
Constructor Details
-
TLcdGXYRoundedPointListPainter
public TLcdGXYRoundedPointListPainter()Constructs a defaultTLcdGXYRoundedPointListPainter
in a POLYLINE mode and with display name "Polypoint". The default roundness factor is 0.5. -
TLcdGXYRoundedPointListPainter
Constructs a defaultTLcdGXYRoundedPointListPainter
in a POLYLINE mode and with display nameaDisplayName
. The default roundness factor is 0.5.- Parameters:
aDisplayName
- the display name for this painter, used for textual representation of this painter.
-
TLcdGXYRoundedPointListPainter
public TLcdGXYRoundedPointListPainter(int aPointListMode) Constructs aTLcdGXYRoundedPointListPainter
in the given mode and with display name "Polypoint". The default roundness factor is 0.5.- Parameters:
aPointListMode
- the mode for the default representation of the point lists. The value should be one of POINT, POLYLINE, POLYGON, FILLED, OUTLINED_FILLED.- See Also:
-
TLcdGXYRoundedPointListPainter
Constructs aTLcdGXYRoundedPointListPainter
in the given mode and with display nameaDisplayName
. The default roundness factor is 0.5.- Parameters:
aDisplayName
- the display name for this painter, used for textual representation of this painter.aPointListMode
- the mode for the default representation of the point lists. The value should be one of POINT, POLYLINE, POLYGON, FILLED, OUTLINED_FILLED.- See Also:
-
-
Method Details
-
setObject
Description copied from class:TLcdGXYPointListPainter
Sets the object to paint or edit. This painter needs anILcdPointList
for painting and (optionally) anILcd2DEditablePointList
for editing. You can change how theILcdPointList
is derived from the object by overridingTLcdGXYPointListPainter.retrievePointList(java.lang.Object)
.- Specified by:
setObject
in interfaceILcdGXYEditor
- Specified by:
setObject
in interfaceILcdGXYPainter
- Overrides:
setObject
in classTLcdGXYPointListPainter
- Parameters:
aObject
- The object to paint and edit.- See Also:
-
retrievePointList
Description copied from class:TLcdGXYPointListPainter
Extracts anILcdPointList
from the given object. The default implementation simply casts the object to anILcdPointList
. Re-define this method to obtain theILcdPointList
by other means.- Overrides:
retrievePointList
in classTLcdGXYPointListPainter
- Returns:
- the point list associated with the given object
- See Also:
-
getObject
Description copied from class:TLcdGXYPointListPainter
Returns the object that can currently be painted or edited by this painter/editor.- Specified by:
getObject
in interfaceILcdGXYEditor
- Specified by:
getObject
in interfaceILcdGXYPainter
- Overrides:
getObject
in classTLcdGXYPointListPainter
- Returns:
- the object that can currently be painted or edited by this painter/editor.
- See Also:
-
getRoundness
public double getRoundness()Returns the roundness factor that is used to render corners of polygons or polylines. This factor is a value in the interval [0.0, 1.0]. By default, 0.5 is used.- Returns:
- the roundness factor for polygon and polyline corners.
- See Also:
-
setRoundness
public void setRoundness(double aRoundness) Sets the roundness factor that should be used to render corners of polygons or polylines. This factor must be a value in the interval [0.0, 1.0], with 0.0 indicating no rounding and 1.0 indicating a maximum rounding, which results in a very smooth corner. This setting does not apply toTLcdRoundedPolyline
orTLcdRoundedPolygon
, because these shapes impose their own roundness.- Parameters:
aRoundness
- the roundness (or rounding) factor for polygon and polyline corners.- Throws:
IllegalArgumentException
- ifaRoundness < 0 || aRoundness > 1.0
.
-
boundsSFCT
public void boundsSFCT(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) throws TLcdNoBoundsException Sets the supplied bounds (in view coordinates, pixels) so that it encompasses the representation of the object and its handles (the source point list) in the given mode taking into account the given context.If this method returns without exception the bounds argument must encompass the representation of the object. A point outside the bounds will not be contained within the painted object.
The bounds returned in this method can be seen as the equivalent in the view space of the bounds in the model space for
ILcdBounded
objects.- Specified by:
boundsSFCT
in interfaceILcdGXYPainter
- Overrides:
boundsSFCT
in classTLcdGXYPointListPainter
- Parameters:
aGraphics
- the Graphics on which the representation of the object is paintedaRenderMode
- the mode the object is represented in (see class documentation). For example, an object may be represented differently in SELECTED mode compared to DEFAULT mode. The returned bounds of the representation must take this different representation into account.aGXYContext
- the context for which the representation of the object is painted. It contains amongst others the transformations from model to world and world to view.aBoundsSFCT
- the bounds that must be adapted to encompass the representation of the object in the given mode and context. These bounds must not be taken into account when the method has thrown an exception.- Throws:
TLcdNoBoundsException
- if no bounds can be determined for the representation of the object. This can happen when the object does not have a representation in the given context, for example when it is located in a part of the world which is not visible in the current view.- See Also:
-
supportSnap
Supports snapping when the
ILcdPointList
object set to this painter contains at least one point andgetRoundness()
equals 0.- Specified by:
supportSnap
in interfaceILcdGXYPainter
- Overrides:
supportSnap
in classTLcdGXYPointListPainter
- Parameters:
aGraphics
- the graphics on which is worked.aGXYContext
- the context of the snapping.- Returns:
true
if theILcdPointList
object contains at least one point andgetRoundness()
equals 0,false
otherwise.
-
clone
Description copied from interface:ILcdCloneable
Makes
When for example extending fromObject.clone()
public.java.lang.Object
, it can be implemented like this:public Object clone() { try { return super.clone(); } catch ( CloneNotSupportedException e ) { // Cannot happen: extends from Object and implements Cloneable (see also Object.clone) throw new RuntimeException( e ); } }
- Specified by:
clone
in interfaceILcdCloneable
- Specified by:
clone
in interfaceILcdGXYEditorProvider
- Specified by:
clone
in interfaceILcdGXYPainterProvider
- Overrides:
clone
in classTLcdGXYPointListPainter
- Returns:
- a clone of this painter provider.
- See Also:
-
getTouchedSegment
Description copied from class:TLcdGXYPointListPainter
Returns the index of the segment in the
ILcdPointList
object that has been touched according to the coordinates in the context, or-1
if none of the segments were touched.If more than one segment of the
ILcdPointList
object is touched, the index of the one with the smaller index is returned.- Overrides:
getTouchedSegment
in classTLcdGXYPointListPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context in which the object is rendered.- Returns:
- the index of the segment of the point list painted that was touched, -1 if none was touched.
-
isTouched
Depending on the render mode and paint mode, returns whether one of the handles (the source point list), the painted point list or the interior of the point list is touched.This implementation will use the specified
paint mode
to determine how the object is represented. Depending on the representation of theILcdPointList
object, the object is touched when- the painted point list is touched,
- its interior space is touched when the paint mode is
TLcdGXYPointListPainter.FILLED
, - the source point list is touched when the render mode is
ILcdGXYPainter.SELECTED
.
The method
insidePolygon
determines whether the current mouse position is located inside theILcdPointList
object or not.It is not possible to derive from this method which part of the point list has been touched. To determine which part of the
ILcdPointList
object is touched, the methodsgetTouchedPoint
andgetTouchedSegment
will return the required information.- Specified by:
isTouched
in interfaceILcdGXYPainter
- Overrides:
isTouched
in classTLcdGXYPointListPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context in which the object is rendered.- Returns:
- whether one of the points, segments or the interior of the point list is touched.
- See Also:
-