Class TLcdGXYBoundsPainter
- All Implemented Interfaces:
ILcdCloneable
,ILcdPropertyChangeSource
,ILcdGXYEditor
,ILcdGXYEditorProvider
,ILcdGXYPainter
,ILcdGXYPainterProvider
,ILcdGXYPathPainter
,Serializable
,Cloneable
ILcdBounds
objects and enables visual editing of
ILcd2DEditableBounds
objects in an ILcdGXYView
.
Painting an ILcdBounds
Body
This painter provides three paint modes to paint the body of an ILcdBounds
object. The mode
property can be set using the method setMode
and allows the bounds to be painted as:
|
||||
![]() |
|
![]() |
|
![]() |
An outlined bounds (OUTLINED ) |
|
A filled bounds (FILLED ) |
|
A filled and outlined bounds (OUTLINED_FILLED ) |
Handles
This painter defines the four corner points of the ILcdBounds
object as a handle of the
bounds. The following image clarifies the handle location, the corner points are represented by red
points.
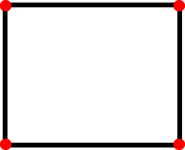
Snap targets
One of the four corner points of the ILcdBounds
object can be returned on the condition
that it is touched. The point returned as a snap target is highlighted with the snapIcon
.
Styling options
The visualization of the ILcdBounds
object is governed by the line style and fill style
set to this painter. Depending on the paint mode chosen one or both of these will be taken into account.
Locating an ILcdBounds in a view
Anchor point of an ILcdBounds
The anchor point of the ILcdBounds
object is located at the center point of the bounds,
calculated by boundsSFCT
.
When is an ILcdBounds touched
Depending on the paint mode, a bounds is touched when either the contour, one of the corner points, or the internal area (when filled) of the bounds is touched.
Visually editing an ILcd2DEditableBounds
Modifying an ILcd2DEditableBounds
This editor provides the following edit functionality for the different render modes:
RESHAPED
: a contour edge or a corner point is reshaped, depending on whether they are touched. The images below illustrate the editing behavior. The small black arrow indicates the path of the mouse cursor while reshaping the bounds.
Moving a corner point.
Moving a contour edge. TRANSLATED
: a corner point or the whole bounds is translated, depending on whether a corner point, the contour or the interior is touched. The images below illustrate the editing behavior. The small black arrow indicates the path of the mouse cursor while translating the bounds.
Moving a corner point.
Moving the contour.
Moving the interior.
Initializing an ILcd2DEditableBounds
When initializing a bounds via interaction through the view, 2
user interactions are required
to complete the initialization. The first will define the first corner point of the bounds and the second
will define the opposite corner point.
Accepted snap targets
All points are accepted as snap target as long as:
- the point can be transformed to the reference of the
ILcdBounds
object with themodel to model transformation
, - the point is not one of the corner points of the
ILcdBounds
object set to this painter.
Additional properties
Caching
This painter implementation supports caching for objects implementing ILcdCache
.
Caching can be turned on/off with the setPaintCache
method.
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final int
Constant value indicating the lower left corner of theILcdBounds
.static final int
Constant value indicating the lower right corner of theILcdBounds
.static final int
Constant value indicating the upper left corner of theILcdBounds
.static final int
Constant value indicating the upper right corner of theILcdBounds
.static final int
Draw theILcdBounds
object as a filled bounds, only the area of the bounds defined by theILcdBounds
is painted.static final int
Draw theILcdBounds
object as an outlined bounds, only the contour of the bounds defined by theILcdBounds
is painted.static final int
Draw theILcdBounds
object as an outlined and filled bounds, both the area and the contour of the bounds defined by theILcdBounds
are painted.Fields inherited from class com.luciad.view.gxy.ALcdGXYPainter
defaultCreationFillStyle, defaultCreationLineStyle, defaultFillStyle, defaultLineStyle, fWorkBounds
Fields inherited from interface com.luciad.view.gxy.ILcdGXYEditor
CREATING, END_CREATION, RESHAPED, START_CREATION, TRANSLATED
Fields inherited from interface com.luciad.view.gxy.ILcdGXYPainter
BODY, CREATING, DEFAULT, HANDLES, RESHAPING, SELECTED, SNAPS, TRANSLATING
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs a defaultTLcdGXYBoundsPainter
in the default modeOUTLINED
and sets the display name to "Bounds". -
Method Summary
Modifier and TypeMethodDescriptionboolean
acceptSnapTarget
(Graphics aGraphics, ILcdGXYContext aGXYContext) Determines whether a snap target should be accepted or not.boolean
appendAWTPath
(ILcdGXYContext aGXYContext, int aRenderMode, ILcdAWTPath aAWTPathSFCT) Appends a discretized representation in view coordinates to the given path.boolean
appendGeneralPath
(ILcdGXYContext aGXYContext, int aRenderMode, ILcdGeneralPath aGeneralPathSFCT) Appends a discretized representation in world coordinates to the given path.void
boundsSFCT
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) Computes the bounds of the representation of anILcdBounds
in AWT coordinates.clone()
MakesObject.clone()
public.boolean
edit
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) This implementation edits theILcdBounds
object set to the painter.int
Returns2
as the number of required user interactions.getCursor
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Returns aCursor
that clarifies the render mode and context this painter is operating in.boolean
Deprecated.getGXYEditor
(Object aObject) Returns this instance as editor for editing the specifiedObject
.int
Returns the minimum pixel distance the input device (a mouse, for example) must move before editing the shape.final Object
Returns the object that can currently be painted or edited by this painter/editor.boolean
Returns whether caching is used when painting this painter's object.Returns the icon that is used to paint snap target points of the object set to this painter.boolean
isTouched
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Depending on the rendering mode, returns whether the contour, one of the corner points, or the interior of the bounds is touched.boolean
Deprecated.This method has been deprecated.protected boolean
linkToSnapTarget
(Graphics aGraphics, ILcd2DEditableBounds a2DEditableBoundsSFCT, int aCornerIndex, int aRenderMode, ILcdGXYContext aGXYContext) Sets the specified corner point at the given snap target, if the snap target is accepted.void
paint
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Displays the representation of the object in the given mode on the Graphics passed, taking into account the context passed.void
setFillSelection
(boolean aFillSelection) Deprecated.useALcdGXYAreaPainter.setSelectionMode(int)
instead.void
setMinimumEditDelta
(int aDelta) Sets the minimum pixel distance the input device (a mouse, for example) must move before editing the shape.void
setModelModelTransformationClass
(Class aModel2ModelTransformationClass) Sets the transformation class that should be used when snapping to points that are defined in a different reference than the reference of this painter's object.void
Sets the object to paint and edit.void
setPaintCache
(boolean aPaintCache) Turns caching of the representation of the object on or off.void
setSnapIcon
(ILcdIcon aSnapIcon) Sets the icon that marks snap targets of the object currently set to this painter.void
setTraceOn
(boolean aTraceOn) Deprecated.This method has been deprecated.protected void
setupGraphicsForFill
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Called just before filling bounds on the specifiedGraphics
object.protected void
setupGraphicsForLine
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Called just before painting a line on the specifiedGraphics
object.snapTarget
(Graphics aGraphics, ILcdGXYContext aGXYContext) Returns one of the bounds points of theILcdBounds
object if it is touched.boolean
supportSnap
(Graphics aGraphics, ILcdGXYContext aGXYContext) This implementation supports snapping, always returnstrue
.Methods inherited from class com.luciad.view.gxy.painter.ALcdGXYAreaPainter
getEditMode, getFillStyle, getLineStyle, getMode, getSelectionMode, setEditMode, setFillStyle, setLineStyle, setMode, setSelectionMode
Methods inherited from class com.luciad.view.gxy.ALcdGXYPainter
addPropertyChangeListener, anchorPointSFCT, firePropertyChangeEvent, firePropertyChangeEvent, getDisplayName, getGXYPainter, removePropertyChangeListener, setClassTraceOn, setDisplayName
Methods inherited from class java.lang.Object
equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface com.luciad.view.gxy.ILcdGXYEditor
getDisplayName
Methods inherited from interface com.luciad.view.gxy.ILcdGXYPainter
anchorPointSFCT, getDisplayName
Methods inherited from interface com.luciad.util.ILcdPropertyChangeSource
addPropertyChangeListener, removePropertyChangeListener
-
Field Details
-
CORNER_LOWER_LEFT
public static final int CORNER_LOWER_LEFTConstant value indicating the lower left corner of theILcdBounds
.- See Also:
-
CORNER_UPPER_LEFT
public static final int CORNER_UPPER_LEFTConstant value indicating the upper left corner of theILcdBounds
.- See Also:
-
CORNER_UPPER_RIGHT
public static final int CORNER_UPPER_RIGHTConstant value indicating the upper right corner of theILcdBounds
.- See Also:
-
CORNER_LOWER_RIGHT
public static final int CORNER_LOWER_RIGHTConstant value indicating the lower right corner of theILcdBounds
.- See Also:
-
OUTLINED
public static final int OUTLINEDDraw theILcdBounds
object as an outlined bounds, only the contour of the bounds defined by theILcdBounds
is painted.- See Also:
-
FILLED
public static final int FILLEDDraw theILcdBounds
object as a filled bounds, only the area of the bounds defined by theILcdBounds
is painted.- See Also:
-
OUTLINED_FILLED
public static final int OUTLINED_FILLEDDraw theILcdBounds
object as an outlined and filled bounds, both the area and the contour of the bounds defined by theILcdBounds
are painted.- See Also:
-
-
Constructor Details
-
TLcdGXYBoundsPainter
public TLcdGXYBoundsPainter()Constructs a defaultTLcdGXYBoundsPainter
in the default modeOUTLINED
and sets the display name to "Bounds".
-
-
Method Details
-
setTraceOn
public void setTraceOn(boolean aTraceOn) Deprecated.This method has been deprecated. It is recommended to use the standard Java logging framework directly.Enables tracing for this class instance. Calling this method with eithertrue
orfalse
as argument automatically turns off tracing for all other class instances for whichsetTraceOn
has not been called. If the argument isfalse
then only the informative, warning and error log messages are recorded.- Overrides:
setTraceOn
in classALcdGXYPainter
- Parameters:
aTraceOn
- if true then all log messages are recorded for this instance. If false, then only the informative, warning and error log messages are recorded.
-
isTraceOn
public boolean isTraceOn()Deprecated.This method has been deprecated. It is recommended to use the standard Java logging framework directly.Returnstrue
if tracing is enabled for this class.- Overrides:
isTraceOn
in classALcdGXYPainter
- Returns:
- true if tracing is enabled for this class, false otherwise.
-
setObject
Sets the object to paint and edit. The object should be an instance of
ILcdBounds
for painting and an instance ofILcd2DEditableBounds
for editing.This painter/editor can be used to paint the object without using the edit functionality. This method shall therefore only check if the object implements
ILcdBounds
and shall throw aClassCastException
if it does not.When this painter is used as editor with an object that does not implement the interface
ILcd2DEditableBounds
, theedit
method shall throw the necessary exception.- Specified by:
setObject
in interfaceILcdGXYEditor
- Specified by:
setObject
in interfaceILcdGXYPainter
- Parameters:
aObject
- The object to paint and edit.- Throws:
ClassCastException
- when the object does not implementILcdBounds
.- See Also:
-
getObject
Returns the object that can currently be painted or edited by this painter/editor.- Specified by:
getObject
in interfaceILcdGXYEditor
- Specified by:
getObject
in interfaceILcdGXYPainter
- Returns:
- the object that can currently be painted or edited by this painter/editor.
- See Also:
-
setPaintCache
public void setPaintCache(boolean aPaintCache) Turns caching of the representation of the object on or off. Caching greatly reduces the time to paint an object but requires more memory. The representation of an object can only be cached for objects which implementILcdCache
. By default, caching is turned on.- Parameters:
aPaintCache
- A flag indicating whether to use caching when painting an object.- See Also:
-
getPaintCache
public boolean getPaintCache()Returns whether caching is used when painting this painter's object.- Returns:
true
if caching is used to paint this painter's object,false
otherwise.- See Also:
-
setFillSelection
public void setFillSelection(boolean aFillSelection) Deprecated.useALcdGXYAreaPainter.setSelectionMode(int)
instead.Determines whether the painter will fill the bounds when it is selected. This property is only valid when the default paint mode property is eitherOUTLINED
,FILLED
orOUTLINED_FILLED
. The default isfalse
.- Parameters:
aFillSelection
- A flag indicating whether to paint the object filled when selected.- See Also:
-
getFillSelection
public boolean getFillSelection()Deprecated.useALcdGXYAreaPainter.getSelectionMode()
instead.Returns whether the object is painted filled in the render modeILcdGXYPainter.SELECTED
.- Returns:
- whether the object is painted filled in the render mode
ILcdGXYPainter.SELECTED
. - See Also:
-
setSnapIcon
Sets the icon that marks snap targets of the object currently set to this painter. This icon is painted when thepaint
method is called with the render modeILcdGXYPainter.SNAPS
.- Parameters:
aSnapIcon
- The icon that should be used to paint snap target points.- See Also:
-
getSnapIcon
Returns the icon that is used to paint snap target points of the object set to this painter.- Returns:
- the icon that is used to paint snap target points of the object set to this painter.
- See Also:
-
setMinimumEditDelta
public void setMinimumEditDelta(int aDelta) Sets the minimum pixel distance the input device (a mouse, for example) must move before editing the shape. A larger value ensures that the object easily "snaps" back in place, a small value allows fine editing.- Parameters:
aDelta
- the minimum pixel distance the input device should move in either the X or Y direction
-
getMinimumEditDelta
public int getMinimumEditDelta()Returns the minimum pixel distance the input device (a mouse, for example) must move before editing the shape. A larger value ensures that the object easily "snaps" back in place, a small value allows fine editing. The default value is 3 pixels.- Returns:
- the minimum pixel distance the input device should move in either the X or Y direction
-
getCursor
Returns a
Cursor
that clarifies the render mode and context this painter is operating in. When no specificCursor
is required,null
is returned.If one of the corner points is touched, the cursor reshaping is returned. In reshaping mode and if none of the corner points is touched but one of the sides is touched a resizing cursor is returned. Otherwise the default cursor is returned (translating).
- Specified by:
getCursor
in interfaceILcdGXYPainter
- Overrides:
getCursor
in classALcdGXYPainter
- Parameters:
aGraphics
- The graphics on which the object is painted.aRenderMode
- The mode to render the object in.aGXYContext
- The context in which the object is rendered.- Returns:
- a cursor to indicate the type of operating mode and context. Returns
null
if no particular cursor is required.
-
getGXYEditor
Returns this instance as editor for editing the specifiedObject
. If thisObject
is not the same as theObject
set to this painter, thesetObject(Object)
method is called to update the object set to the painter.- Specified by:
getGXYEditor
in interfaceILcdGXYEditorProvider
- Parameters:
aObject
- the object to be edited.- Returns:
- this instance as editor for editing the specified
Object
.
-
getCreationClickCount
public int getCreationClickCount()Returns2
as the number of required user interactions.- Specified by:
getCreationClickCount
in interfaceILcdGXYEditor
- Returns:
2
.
-
isTouched
Depending on the rendering mode, returns whether the contour, one of the corner points, or the interior of the bounds is touched.This implementation will use the specified
paint mode
to determine how the object is represented. Depending on the representation of theILcdBounds
object, the object is touched if the contour, one of the corner points, or its interior space (when it is painted filled) is touched. The coordinate for which the check is done is derived from the context passed as argument.- Specified by:
isTouched
in interfaceILcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context in which the object is rendered.- Returns:
- whether the contour, one of the corner points, or the interior of the bounds is touched.
- See Also:
-
paint
Displays the representation of the object in the given mode on the Graphics passed, taking into account the context passed.This implementation will use the specified render mode to determine how the object needs to be rendered. In
ILcdGXYPainter.CREATING
,ILcdGXYPainter.RESHAPING
andILcdGXYPainter.TRANSLATING
mode, the object is painted using the edit paint mode (ALcdGXYAreaPainter.getEditMode()
). The object is rendered as shown in the images of the class documentation.In the render mode
ILcdGXYPainter.SELECTED
, the object is painted using the selection paint mode (ALcdGXYAreaPainter.getSelectionMode()
). In all other render modes, the paint mode (ALcdGXYAreaPainter.getMode()
) is used.- Specified by:
paint
in interfaceILcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.
-
edit
This implementation edits the
ILcdBounds
object set to the painter. The object needs to implement the interfaceILcd2DEditableBounds
otherwise aClassCastException
will be thrown.In
ILcdGXYEditor.TRANSLATED
render mode, theILcdBounds
object is edited as follows:- While touching a corner point, the corner point gets translated.
- While touching the contour, the object gets translated as a whole.
- While touching the interior and the selected object is painted as filled, the object gets translated as a whole.
In
ILcdGXYEditor.RESHAPED
render mode, theILcdBounds
object is edited as follows:- While touching a corner point, the corner point will move while enlarging or reducing the width and height of the bounds.
- While touching the contour, the edge line will move while enlarging or reducing the width or height of the bounds.
In the creation render modes, two user interactions are required. The first will define the first corner point of the bounds and the second will define the opposite corner point.
If, in all render modes, a snap target is found for a moved or created point, the snap target location is used for the moved/created point.
- Specified by:
edit
in interfaceILcdGXYEditor
- Parameters:
aGraphics
- The graphics to edit the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.- Returns:
- true if the object has changed when this method returns, false otherwise.
- Throws:
ClassCastException
- if the painter's object does not implementILcd2DEditableBounds
.- See Also:
-
boundsSFCT
public void boundsSFCT(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) throws TLcdNoBoundsException Computes the bounds of the representation of anILcdBounds
in AWT coordinates. The bounds will include the corner handles depending on the painter mode passed.- Specified by:
boundsSFCT
in interfaceILcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.aBoundsSFCT
- The bounds to update.- Throws:
TLcdNoBoundsException
- if no bounds can be determined for the representation of the object. This can be when the object does not have a representation in the given context, for example when it is located in a part of the world which is not visible in the projection as set in the views world reference.- See Also:
-
supportSnap
This implementation supports snapping, always returnstrue
.- Specified by:
supportSnap
in interfaceILcdGXYPainter
- Overrides:
supportSnap
in classALcdGXYPainter
- Parameters:
aGraphics
- the graphics on which is worked.aGXYContext
- the context of the snapping.- Returns:
true
.
-
snapTarget
Returns one of the bounds points of the
ILcdBounds
object if it is touched. If no point was touched,null
will be returned.- Specified by:
snapTarget
in interfaceILcdGXYPainter
- Overrides:
snapTarget
in classALcdGXYPainter
- Parameters:
aGraphics
- the graphics on which is worked.aGXYContext
- the context of the snapping.- Returns:
- one of the bounds points of the
ILcdBounds
object if it is touched,null
otherwise. - See Also:
-
appendAWTPath
Description copied from interface:ILcdGXYPathPainter
Appends a discretized representation in view coordinates to the given path.- Specified by:
appendAWTPath
in interfaceILcdGXYPathPainter
- Parameters:
aGXYContext
- the graphics contextaRenderMode
- the rendering modeaAWTPathSFCT
- the path to append to- Returns:
- true if a discretization of the shape could be successfully appended to the path
-
appendGeneralPath
public boolean appendGeneralPath(ILcdGXYContext aGXYContext, int aRenderMode, ILcdGeneralPath aGeneralPathSFCT) Description copied from interface:ILcdGXYPathPainter
Appends a discretized representation in world coordinates to the given path.- Specified by:
appendGeneralPath
in interfaceILcdGXYPathPainter
- Parameters:
aGXYContext
- the graphics contextaRenderMode
- the rendering modeaGeneralPathSFCT
- the path to append to- Returns:
- true if a discretization of the shape could be successfully appended to the path
-
setModelModelTransformationClass
Sets the transformation class that should be used when snapping to points that are defined in a different reference than the reference of this painter's object. The transformation will be instantiated and setup to transform points from the model of the snap layer to points in the model which contains the current object of this painter. The default value is the
TLcdGeoReference2GeoReference
class.This property allows the painter to snap to points defined in a different model reference.
Instances of this specified class should implement
ILcdModelModelTransformation
, otherwise the snapping functionality to different model references will not work.- Parameters:
aModel2ModelTransformationClass
- the transformation used as described above.
-
acceptSnapTarget
Determines whether a snap target should be accepted or not. A snap target is accepted if the following conditions are met:
- The current object is an
ILcd2DEditableBounds
. - The snap target is an
ILcdPoint
whose coordinates are expressed in the same coordinate system as the current objects coordinate system or the model to model transformation can transform the point to a point in the objects coordinate system. - The snap object is not one of the corner points of the current object. If this is wanted, override the function and return true if the snap object is one of the corner points.
- One of the corner points is touched or the width and the height of the bounds are
0
. This enables snapping when creating a bounds.
- Specified by:
acceptSnapTarget
in interfaceILcdGXYEditor
- Parameters:
aGraphics
- the graphics on which the snap target should be checked.aGXYContext
- the context in which the snap target should be checked.- Returns:
true
if the above conditions are met,false
otherwise.- See Also:
- The current object is an
-
setupGraphicsForLine
protected void setupGraphicsForLine(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Called just before painting a line on the specified
Graphics
object. It can be redefined in order to set specificGraphics
properties likeColor
, etc...This implementation calls
ILcdGXYPainterStyle.setupGraphics(java.awt.Graphics, java.lang.Object, int, com.luciad.view.gxy.ILcdGXYContext)
on the lineStyle property if a line style has been set to this instance.- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.
-
setupGraphicsForFill
protected void setupGraphicsForFill(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Called just before filling bounds on the specified
Graphics
object. It can be redefined in order to set specificGraphics
properties likeColor
, etc...This implementation calls
ILcdGXYPainterStyle.setupGraphics(java.awt.Graphics, java.lang.Object, int, com.luciad.view.gxy.ILcdGXYContext)
on the fillStyle property if a fill style has been set to this instance.- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.
-
linkToSnapTarget
protected boolean linkToSnapTarget(Graphics aGraphics, ILcd2DEditableBounds a2DEditableBoundsSFCT, int aCornerIndex, int aRenderMode, ILcdGXYContext aGXYContext) Sets the specified corner point at the given snap target, if the snap target is accepted. The corner index should be is one of the predefined constants (CORNER_LOWER_LEFT
,CORNER_LOWER_RIGHT
,CORNER_UPPER_LEFT
,CORNER_UPPER_RIGHT
).- Parameters:
aGraphics
- The graphics to paint the object on.a2DEditableBoundsSFCT
- The bounds objects to be edited.aCornerIndex
- The corner index to set at the snap target.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.- Returns:
- whether the corner was successfully linked to the snap target.
- See Also:
-
clone
Description copied from interface:ILcdCloneable
Makes
When for example extending fromObject.clone()
public.java.lang.Object
, it can be implemented like this:public Object clone() { try { return super.clone(); } catch ( CloneNotSupportedException e ) { // Cannot happen: extends from Object and implements Cloneable (see also Object.clone) throw new RuntimeException( e ); } }
- Specified by:
clone
in interfaceILcdCloneable
- Specified by:
clone
in interfaceILcdGXYEditorProvider
- Specified by:
clone
in interfaceILcdGXYPainterProvider
- Overrides:
clone
in classALcdGXYAreaPainter
- Returns:
- a clone of this painter provider.
- See Also:
-
ALcdGXYAreaPainter.getSelectionMode()
instead.