Class TLcdLonLatHeightBuffer
- All Implemented Interfaces:
ILcdBounded
,ILcdPointList
,ILcdShape
,ILcd2DEditablePointList
,ILcd2DEditableShape
,ILcd3DEditablePointList
,ILcd3DEditableShape
,ILcdCache
,ILcdCloneable
,Serializable
,Cloneable
- The buffer's
width
is from the axis to the edge of the buffer on both sides, so the buffer is 2 * width wide. - The buffer's
height
is from the bottom to the top of the buffer, half of the height above the axis, half beneath.
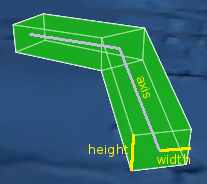
All longitude/latitude coordinates are expressed in degrees. Lengths are expressed in meters.
For efficiency reasons the current implementation works
with a spherical approximation where the radius of the sphere
is the major axis of the ellipsoid. If no ellipsoid is specified
at the construction of the TLcdLonLatHeightBuffer
object
the default ellipsoid is used.
This class is thread-safe for concurrent read-only access of its contents. For read-write access, external locking must be used. Such locking is typically done at the model level.
For a more flexible 3D buffer, see TLcdLonLatHeightVariableGeoBuffer
.
- See Also:
-
Constructor Summary
ConstructorsConstructorDescriptionCreates a newTLcdLonLatHeightBuffer
, without points, with a default width of 300km and height of 100mTLcdLonLatHeightBuffer
(ILcd3DEditablePoint[] aAxisPointArray, double aWidth, double aHeight) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.TLcdLonLatHeightBuffer
(ILcd3DEditablePoint[] aAxisPointArray, double aWidth, double aHeight, ILcdEllipsoid aEllipsoid) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.TLcdLonLatHeightBuffer
(ILcd3DEditablePointList aAxisPointList, double aWidth, double aHeight) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.TLcdLonLatHeightBuffer
(ILcd3DEditablePointList aAxisPointList, double aWidth, double aHeight, ILcdEllipsoid aEllipsoid) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.TLcdLonLatHeightBuffer
(TLcdLonLatHeightBuffer aLonLatHeightBuffer) Copy constructor.TLcdLonLatHeightBuffer
(TLcdLonLatHeightPolyline aAxisPolyline, double aWidth, double aHeight) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.TLcdLonLatHeightBuffer
(TLcdLonLatHeightPolyline aAxisPolyline, double aWidth, double aHeight, ILcdEllipsoid aEllipsoid) Creates a newTLcdLonLatHeightBuffer
with the specified parameters. -
Method Summary
Modifier and TypeMethodDescriptionvoid
Clears the cache.clone()
Creates and returns a copy of this object.boolean
contains2D
(double x, double y) Checks whether thisILcdShape
contains the given point in the 2D space.boolean
contains2D
(ILcdPoint aPoint) Checks whether thisALcdShape
contains the given point in the 2D cartesian plane.boolean
contains3D
(double aX, double aY, double aZ) Provides an approximate default implementation of the 3D containment test based on the 2D containment test.boolean
contains3D
(ILcdPoint aPoint) Checks whether thisALcdShape
contains the given point in the 3D space.boolean
Returns whether the given object has the same class and the same coordinates and parameters.final ILcdPolyline
Returns theILcdBounds
by which the geometry of thisILcdBounded
object is bounded.getCachedObject
(Object aKey) Looks up and returns the cached Object corresponding to the given key.Returns the focus point of thisILcdShape
.double
Returns the height of the buffer.getPoint
(int aIndex) Returns theILcdPoint
at a given index.int
Returns the number ofILcdPoint
objects in the list.double
getWidth()
Returns the distance from the axis to the buffer edge.int
hashCode()
The hash code of this shape is the hash code of its class, in order to be consistent with theALcdShape.equals(Object)
method.void
insert2DPoint
(int aIndex, double aX, double aY) Inserts a point at the given index into thisILcd2DEditablePointList
.void
insert3DPoint
(int aIndex, double aX, double aY, double aZ) Inserts a point at the given index into thisILcd3DEditablePointList
.void
insertIntoCache
(Object aKey, Object aObject) Inserts a cache Object corresponding to the given key Object.void
move2D
(double aX, double aY) Translates this shape so that its focus point ends up at the specified position.void
move2DPoint
(int aIndex, double aX, double aY) Moves the specified point of thisILcd2DEditablePointList
to the given point in the 2D space.void
move3DPoint
(int aIndex, double aX, double aY, double aZ) Moves the specified point of thisILcd3DEditablePointList
to the given point in the 3D space.removeCachedObject
(Object aKey) Looks up and removes the cached Object corresponding to the given key.void
removePointAt
(int aIndex) Removes the point at the given index from thisILcd3DEditablePointList
.void
setHeight
(double aHeight) Set the height of this buffer.void
setWidth
(double aWidth) Set the distance is from the axis to the buffer edge.toString()
void
translate2D
(double aX, double aY) Translates all the points of thisILcd2DEditablePointList
from their current positions over the given translation vector in the 2D space.void
translate2DPoint
(int aIndex, double aX, double aY) Translates the specified point of thisILcd2DEditablePointList
from its current position over the given translation vector in the 2D space.void
translate3D
(double aX, double aY, double aZ) Translates all the points of thisILcd3DEditablePointList
from their current positions over the given translation vector in the 3D space.void
translate3DPoint
(int aIndex, double aX, double aY, double aZ) Translates the specified point of thisILcd3DEditablePointList
from its current position over the given translation vector in the 3D space.Methods inherited from class com.luciad.shape.shape3D.ALcd3DEditableShape
move3D, move3D
Methods inherited from class com.luciad.shape.shape2D.ALcd2DEditableShape
move2D
Methods inherited from class com.luciad.shape.ALcdShape
fromDomainObject
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
Methods inherited from interface com.luciad.shape.shape2D.ILcd2DEditablePointList
append2DPoint
Methods inherited from interface com.luciad.shape.shape2D.ILcd2DEditableShape
move2D
Methods inherited from interface com.luciad.shape.ILcdPointList
getPointSFCT, getX, getY, getZ
-
Constructor Details
-
TLcdLonLatHeightBuffer
public TLcdLonLatHeightBuffer(TLcdLonLatHeightPolyline aAxisPolyline, double aWidth, double aHeight, ILcdEllipsoid aEllipsoid) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.- Parameters:
aAxisPolyline
- the polyline defining the axis of the corridor.aWidth
- the width in meters of the corridor (>= 0), from the axis to the edgeaHeight
- the full height in meters of the corridor (>= 0), around the axisaEllipsoid
- the ellipsoid used
-
TLcdLonLatHeightBuffer
public TLcdLonLatHeightBuffer(ILcd3DEditablePoint[] aAxisPointArray, double aWidth, double aHeight, ILcdEllipsoid aEllipsoid) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.- Parameters:
aAxisPointArray
- the points defining the axis of the corridor.aWidth
- the width in meters of the corridor (>= 0), from the axis to the edgeaHeight
- the full height in meters of the corridor (>= 0), around the axisaEllipsoid
- the ellipsoid used
-
TLcdLonLatHeightBuffer
public TLcdLonLatHeightBuffer(ILcd3DEditablePointList aAxisPointList, double aWidth, double aHeight, ILcdEllipsoid aEllipsoid) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.- Parameters:
aAxisPointList
- the points defining the axis of the corridor.aWidth
- the width in meters of the corridor (>= 0), from the axis to the edgeaHeight
- the full height in meters of the corridor (>= 0), around the axisaEllipsoid
- the ellipsoid used
-
TLcdLonLatHeightBuffer
public TLcdLonLatHeightBuffer(TLcdLonLatHeightPolyline aAxisPolyline, double aWidth, double aHeight) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.- Parameters:
aAxisPolyline
- the polyline defining the axis of the corridor.aWidth
- the width in meters of the corridor (>= 0), from the axis to the edgeaHeight
- the full height in meters of the corridor (>= 0), around the axis
-
TLcdLonLatHeightBuffer
Creates a newTLcdLonLatHeightBuffer
with the specified parameters.- Parameters:
aAxisPointArray
- the points defining the axis of the corridor.aWidth
- the width in meters of the corridor (>= 0), from the axis to the edgeaHeight
- the full height in meters of the corridor (>= 0), around the axis
-
TLcdLonLatHeightBuffer
public TLcdLonLatHeightBuffer(ILcd3DEditablePointList aAxisPointList, double aWidth, double aHeight) Creates a newTLcdLonLatHeightBuffer
with the specified parameters.- Parameters:
aAxisPointList
- the points defining the axis of the corridor.aWidth
- the width in meters of the corridor (>= 0), from the axis to the edgeaHeight
- the full height in meters of the corridor (>= 0), around the axis
-
TLcdLonLatHeightBuffer
public TLcdLonLatHeightBuffer()Creates a newTLcdLonLatHeightBuffer
, without points, with a default width of 300km and height of 100m -
TLcdLonLatHeightBuffer
Copy constructor.- Parameters:
aLonLatHeightBuffer
- TheTLcdLonLatHeightBuffer
to copy.
-
-
Method Details
-
getEllipsoid
-
getWidth
public double getWidth()Returns the distance from the axis to the buffer edge.The buffer is 2 * width wide in total.
- Returns:
- The width of the buffer, from axis to edge
-
setWidth
public void setWidth(double aWidth) Set the distance is from the axis to the buffer edge. The buffer is 2 * width wide in total.- Parameters:
aWidth
- the width in meters of the corridor (>= 0), from the axis to the edge
-
getHeight
public double getHeight()Returns the height of the buffer.The height is from bottom to top of the buffer, half of the height beneath and half of the height above the axis.
- Returns:
- The height
-
setHeight
public void setHeight(double aHeight) Set the height of this buffer.The height is from bottom to top of the buffer, half of the height beneath and half of the height above the axis.
- Parameters:
aHeight
- the full height in meters of the corridor (>= 0), around the axis
-
getPointCount
public int getPointCount()Description copied from interface:ILcdPointList
Returns the number ofILcdPoint
objects in the list.- Specified by:
getPointCount
in interfaceILcdPointList
- Returns:
- the number of
ILcdPoint
objects in the list.
-
getPoint
Description copied from interface:ILcdPointList
Returns theILcdPoint
at a given index.- Specified by:
getPoint
in interfaceILcdPointList
- Parameters:
aIndex
- a valid index in the list ofILcdPoint
objects.- Returns:
- the
ILcdPoint
at the given index.
-
removePointAt
public void removePointAt(int aIndex) Description copied from interface:ILcd3DEditablePointList
Removes the point at the given index from thisILcd3DEditablePointList
.- Specified by:
removePointAt
in interfaceILcd2DEditablePointList
- Specified by:
removePointAt
in interfaceILcd3DEditablePointList
- Parameters:
aIndex
- a valid index in the list ofILcdPoint
objects.
-
move2D
public void move2D(double aX, double aY) Description copied from class:ALcd2DEditableShape
Translates this shape so that its focus point ends up at the specified position.- Specified by:
move2D
in interfaceILcd2DEditableShape
- Overrides:
move2D
in classALcd2DEditableShape
- Parameters:
aX
- the x coordinate of the point.aY
- the y coordinate of the point.
-
translate2D
public void translate2D(double aX, double aY) Description copied from interface:ILcd2DEditablePointList
Translates all the points of thisILcd2DEditablePointList
from their current positions over the given translation vector in the 2D space. Only the first two dimensions of the points are considered. The third dimension is left unchanged.- Specified by:
translate2D
in interfaceILcd2DEditablePointList
- Specified by:
translate2D
in interfaceILcd2DEditableShape
- Parameters:
aX
- the x coordinate of the translation vector.aY
- the y coordinate of the translation vector.
-
translate3D
public void translate3D(double aX, double aY, double aZ) Description copied from interface:ILcd3DEditablePointList
Translates all the points of thisILcd3DEditablePointList
from their current positions over the given translation vector in the 3D space.- Specified by:
translate3D
in interfaceILcd3DEditablePointList
- Specified by:
translate3D
in interfaceILcd3DEditableShape
- Parameters:
aX
- the x coordinate of the translation vector.aY
- the y coordinate of the translation vector.aZ
- the z coordinate of the translation vector.
-
move2DPoint
public void move2DPoint(int aIndex, double aX, double aY) Description copied from interface:ILcd2DEditablePointList
Moves the specified point of thisILcd2DEditablePointList
to the given point in the 2D space. Only the first two dimensions of theILcdShape
are considered. The third dimension is left unchanged.- Specified by:
move2DPoint
in interfaceILcd2DEditablePointList
- Parameters:
aIndex
- a valid index in the list of points.aX
- the x coordinate of the point.aY
- the y coordinate of the point.
-
move3DPoint
public void move3DPoint(int aIndex, double aX, double aY, double aZ) Description copied from interface:ILcd3DEditablePointList
Moves the specified point of thisILcd3DEditablePointList
to the given point in the 3D space.- Specified by:
move3DPoint
in interfaceILcd3DEditablePointList
- Parameters:
aIndex
- a valid index in the list of points.aX
- the x coordinate of the point.aY
- the y coordinate of the point.aZ
- the z coordinate of the point.
-
translate2DPoint
public void translate2DPoint(int aIndex, double aX, double aY) Description copied from interface:ILcd2DEditablePointList
Translates the specified point of thisILcd2DEditablePointList
from its current position over the given translation vector in the 2D space. Only the first two dimensions of the points are considered. The third dimension is left unchanged.- Specified by:
translate2DPoint
in interfaceILcd2DEditablePointList
- Parameters:
aX
- the x coordinate of the translation vector.aY
- the y coordinate of the translation vector.
-
translate3DPoint
public void translate3DPoint(int aIndex, double aX, double aY, double aZ) Description copied from interface:ILcd3DEditablePointList
Translates the specified point of thisILcd3DEditablePointList
from its current position over the given translation vector in the 3D space.- Specified by:
translate3DPoint
in interfaceILcd3DEditablePointList
- Parameters:
aIndex
- a valid new index in the list of points.aX
- the x coordinate of the translation vector.aY
- the y coordinate of the translation vector.aZ
- the z coordinate of the translation vector.
-
insert2DPoint
public void insert2DPoint(int aIndex, double aX, double aY) Description copied from interface:ILcd2DEditablePointList
Inserts a point at the given index into thisILcd2DEditablePointList
.- Specified by:
insert2DPoint
in interfaceILcd2DEditablePointList
- Parameters:
aIndex
- a valid new index in the list of points.aX
- the x coordinate of the new point.aY
- the y coordinate of the new point.
-
insert3DPoint
public void insert3DPoint(int aIndex, double aX, double aY, double aZ) Description copied from interface:ILcd3DEditablePointList
Inserts a point at the given index into thisILcd3DEditablePointList
.- Specified by:
insert3DPoint
in interfaceILcd3DEditablePointList
- Parameters:
aIndex
- a valid new index in the list of points.aX
- the x coordinate of the new point.aY
- the y coordinate of the new point.aZ
- the z coordinate of the new point.
-
getAxisPolyline
-
contains2D
Description copied from class:ALcdShape
Checks whether thisALcdShape
contains the given point in the 2D cartesian plane.- Specified by:
contains2D
in interfaceILcdShape
- Overrides:
contains2D
in classALcdShape
- Parameters:
aPoint
- the point to test.- Returns:
- the boolean result of the containment test.
- See Also:
-
contains2D
public boolean contains2D(double x, double y) Description copied from interface:ILcdShape
Checks whether thisILcdShape
contains the given point in the 2D space. Only the first two dimensions of theILcdShape
are considered.- Specified by:
contains2D
in interfaceILcdShape
- Parameters:
x
- the x coordinate of the point.y
- the y coordinate of the point.- Returns:
- the boolean result of the containment test.
-
contains3D
Description copied from class:ALcdShape
Checks whether thisALcdShape
contains the given point in the 3D space.- Specified by:
contains3D
in interfaceILcdShape
- Overrides:
contains3D
in classALcdShape
- Parameters:
aPoint
- the point to test.- Returns:
- the boolean result of the containment test.
- See Also:
-
contains3D
public boolean contains3D(double aX, double aY, double aZ) Description copied from class:ALcd2DEditableShape
Provides an approximate default implementation of the 3D containment test based on the 2D containment test.- Specified by:
contains3D
in interfaceILcdShape
- Overrides:
contains3D
in classALcd2DEditableShape
- Parameters:
aX
- the x coordinate of the point.aY
- the y coordinate of the point.aZ
- the z coordinate of the point.- Returns:
true
if the bounds of this shape contain the point in the 3D space and the shape itself contains the point in the 2D space,false
otherwise.
-
getFocusPoint
Description copied from interface:ILcdShape
Returns the focus point of thisILcdShape
.- Specified by:
getFocusPoint
in interfaceILcdShape
- Returns:
- the focus point of this
ILcdShape
.
-
getBounds
Description copied from interface:ILcdBounded
Returns theILcdBounds
by which the geometry of thisILcdBounded
object is bounded.If the geometry does not allow retrieving valid bounds (for example a polyline with 0 points) the return value is unspecified. It is highly recommended to return an
undefined
bounds. You can create undefined bounds using the default constructors ofTLcdLonLatBounds
orTLcdXYBounds
.- Specified by:
getBounds
in interfaceILcdBounded
- Returns:
- the
ILcdBounds
by which the geometry of thisILcdBounded
object is bounded.
-
getContour
-
insertIntoCache
Description copied from interface:ILcdCache
Inserts a cache Object corresponding to the given key Object.- Specified by:
insertIntoCache
in interfaceILcdCache
- Parameters:
aKey
- the key Object that will be used to identify the Object. The key must therefore be a unique identifier, typically the caller itself:insertIntoCache(this, ...)
.aObject
- the Object to be cached.
-
getCachedObject
Description copied from interface:ILcdCache
Looks up and returns the cached Object corresponding to the given key.- Specified by:
getCachedObject
in interfaceILcdCache
- Parameters:
aKey
- the key Object that was used for storing the cache Object.- Returns:
- the cached Object, or null if there is no Object corresponding to the given key.
-
removeCachedObject
Description copied from interface:ILcdCache
Looks up and removes the cached Object corresponding to the given key.- Specified by:
removeCachedObject
in interfaceILcdCache
- Parameters:
aKey
- the key Object that was used for storing the cache Object.- Returns:
- the cached Object, or null if there was no Object corresponding to the given key.
-
clearCache
public void clearCache()Description copied from interface:ILcdCache
Clears the cache.- Specified by:
clearCache
in interfaceILcdCache
-
clone
Creates and returns a copy of this object.The axis polyline is cloned.
The contained ellipsoid is copied without being cloned.
The cache is not copied or cloned at all.
- Specified by:
clone
in interfaceILcdCloneable
- Overrides:
clone
in classALcdShape
- See Also:
-
equals
Returns whether the given object has the same class and the same coordinates and parameters. -
hashCode
public int hashCode()Description copied from class:ALcdShape
The hash code of this shape is the hash code of its class, in order to be consistent with theALcdShape.equals(Object)
method. Extensions should refine this implementation, based on their properties. -
toString
-