Class TLspVariableGeoBufferEditor
java.lang.Object
com.luciad.view.lightspeed.editor.ALspEditor
com.luciad.view.lightspeed.editor.TLspVariableGeoBufferEditor
- All Implemented Interfaces:
ILspEditor
Enables visual editing of 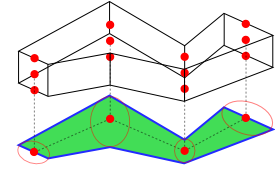
ILcd3DEditableVariableGeoBuffer
objects in an ILspView
.
Handles
The LLH buffer editor defines the following edit handles for a variable geo buffer object in addition to the 3D point list handles:- Point width/height handles: allows the user to edit the with of a single buffer point.
This handle generates
PROPERTY_CHANGE
operations, with ""width"" as property name, with aPOINT_INDEX
property and aDouble
as value. In 3D, it is also possible to edit the heights below and above of a single buffer point. In that case ""heightBelow"" or ""heightAbove"" is used as property name. - Outline handle: allows the user to change all widths of all points of the buffer by dragging
the outline. this handle generates
PROPERTY_CHANGE
operations, with ""width"" as property name, without aPOINT_INDEX
property, and aDouble
as value. In 3D it is also possible to edit the heights below and above. In that case ""heightBelow"" or ""heightAbove"" is used as property name.
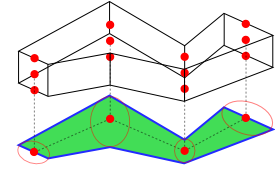
Editing
Based on theTLspEditOperation
, generated by an edit handle (see description of
handles above), this editor performs different edit operations on the associated buffer object.
The images below illustrate the effect of the different handles. In each image the gray color
represents the previous state of the object and the red color represents the edited object:
-
Point width handle: Allows to edit the width of a single buffer point.
-
Point height above/below handles: Allows to edit the height above/below of a single
buffer point.
-
Outline width handle: Allows to edit the width of the buffer by dragging its outline.
Creation
The creation process of a variable geo buffer object consists of a series of two distinct steps:- the first step initializes the XY location of the next axis point.
- the second step initializes the height of that point.
![]() |
![]() |
![]() |
![]() |
|||
Setting the XY location of the buffer's first axis point | Setting the height of the buffer's first axis point | Setting the XY location of the buffer's second axis point | Setting the height of the buffer's second axis point |
- Since:
- 2012.1
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Defines keys used by the enclosing editor implementation to store properties in its edit handles. -
Constructor Summary
ConstructorsConstructorDescriptionCreates a newTLspVariableGeoBufferEditor
.TLspVariableGeoBufferEditor
(TLsp3DPointListEditor aCustomPointListEditorDelegate) Creates a newTLspGeoBufferEditor
. -
Method Summary
Modifier and TypeMethodDescriptionboolean
canEdit
(TLspEditContext aContext) Returnstrue
ifsuper
returnstrue
and the given object is an instance ofILcd2DEditableVariableGeoBuffer
.protected ALspEditHandle
createObjectTranslationHandle
(ILcd2DEditableVariableGeoBuffer aBuffer, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given buffer horizontally (i.e. parallel to the terrain).protected ALspEditHandle
createOutlineWidthHandle
(ILcdVariableGeoBuffer aBuffer, TLspEditContext aContext) Creates a handle to edit the width of all points of the buffer.protected ALspEditHandle
createPointHeightAboveHandle
(ILcdVariableGeoBuffer aBuffer, int aPointIndex, TLspEditContext aContext) Creates a handle to edit the height above property of a point of the buffer.protected ALspEditHandle
createPointHeightBelowHandle
(ILcdVariableGeoBuffer aBuffer, int aPointIndex, TLspEditContext aContext) Creates a handle to edit the height below property of a point of the buffer.protected ALspEditHandle
createPointWidthHandle
(ILcdVariableGeoBuffer aBuffer, int aPointIndex, TLspEditContext aContext) Creates a handle to edit the width property of a point of the buffer.protected TLspEditOperationResult
editImpl
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.Methods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
canCopyGeometry, canPerformOperation, copyGeometrySFCT, edit, fireUndoableHappened
-
Constructor Details
-
TLspVariableGeoBufferEditor
public TLspVariableGeoBufferEditor()Creates a newTLspVariableGeoBufferEditor
. This constructor initializes a defaultTLsp3DPointListEditor
to be used as a delegate when editing and creating points in a variable geo buffer. -
TLspVariableGeoBufferEditor
Creates a newTLspGeoBufferEditor
. This constructor uses the givenTLsp3DPointListEditor
as a delegate when editing and creating the base shape of a variable geo buffer.- Parameters:
aCustomPointListEditorDelegate
- the delegate point list editor to be used for creating and editing points the the base shape of the variable geo buffer.
-
-
Method Details
-
canEdit
Returnstrue
ifsuper
returnstrue
and the given object is an instance ofILcd2DEditableVariableGeoBuffer
.- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this method delegates to the following methods:createPointHeightBelowHandle
createPointHeightAboveHandle
createPointWidthHandle
createOutlineWidthHandle
createObjectTranslationHandle
TLsp3DPointListEditor
.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createObjectTranslationHandle
protected ALspEditHandle createObjectTranslationHandle(ILcd2DEditableVariableGeoBuffer aBuffer, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given buffer horizontally (i.e. parallel to the terrain).Note that by default this method returns a different handle depending on whether the view is a 2D- or a 3D view: in the 2D case, a
TLspObjectTranslationHandle
is returned, otherwise aTLspProjectedObjectTranslationHandle
is returned.- Parameters:
aBuffer
- the buffer for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
when no handle is needed
-
createPointWidthHandle
protected ALspEditHandle createPointWidthHandle(ILcdVariableGeoBuffer aBuffer, int aPointIndex, TLspEditContext aContext) Creates a handle to edit the width property of a point of the buffer. Override this method to change the behavior of the handle, or make it returnnull
to disable creation of these handles.- Parameters:
aBuffer
- the buffer for which to create a width point handle.aPointIndex
- the index of the point for which to create a handle.aContext
- context information.- Returns:
- an edit handle to change the width property of a point of the baseshape, or
null
if this handle should not be created.
-
createPointHeightBelowHandle
protected ALspEditHandle createPointHeightBelowHandle(ILcdVariableGeoBuffer aBuffer, int aPointIndex, TLspEditContext aContext) Creates a handle to edit the height below property of a point of the buffer. Override this method to change the behavior of the handle, or make it returnnull
to disable creation of these handles. By default this method only returns a handle when the view is a 3D view, and when the buffer object is aILcd3DEditableVariableGeoBuffer
.- Parameters:
aBuffer
- the buffer for which to create a height below point handle.aPointIndex
- the index of the point for which to create a handle.aContext
- context information.- Returns:
- an edit handle to change the height below of a point of the baseshape, or
null
if this handle should not be created.
-
createPointHeightAboveHandle
protected ALspEditHandle createPointHeightAboveHandle(ILcdVariableGeoBuffer aBuffer, int aPointIndex, TLspEditContext aContext) Creates a handle to edit the height above property of a point of the buffer. Override this method to change the behavior of the handle, or make it returnnull
to disable creation of these handles. By default this method only returns a handle when the view is a 3D view, and when the buffer object is aILcd3DEditableVariableGeoBuffer
.- Parameters:
aBuffer
- the buffer for which to create a height above point handle.aPointIndex
- the index of the point for which to create a handle.aContext
- context information.- Returns:
- an edit handle to change the height above of a point of the baseshape, or
null
if this handle should not be created.
-
createOutlineWidthHandle
protected ALspEditHandle createOutlineWidthHandle(ILcdVariableGeoBuffer aBuffer, TLspEditContext aContext) Creates a handle to edit the width of all points of the buffer. Override this method to change the behavior of the handle, or make it returnnull
to disable creation of these handles.- Parameters:
aBuffer
- the buffer for which to create a handle.aContext
- context information.- Returns:
- an edit handle to change the width of all points of the buffer, or
null
if this handle should not be created.
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations:TLspEditOperationType.MOVE
TLspEditOperationType.INSERT_POINT
TLspEditOperationType.REMOVE_POINT
TLspEditOperationType.PROPERTY_CHANGE
PROPERTY_CHANGE
operation is used, the following property names are supported:""width""
: The width of the buffer is modified. Depending on the presence of aPOINT_INDEX
property, the width is modified at one of the axis points, or the entire buffer width is modified.""heightBelow""
: The height below property of the buffer is modified. Depending on the presence of aPOINT_INDEX
property, the height below property is modified at one of the axis points, or the height below property is modified for all axis points in the buffer.""heightAbove""
: The height above property of the buffer is modified. Depending on the presence of aPOINT_INDEX
property, the height above property is modified at one of the axis points, or the height above property is modified for all axis points in the buffer.
1.0
, the width of each point remains unchanged. When the new value is2.0
, the width is doubled for every point. If the width (or height below or height above) property is changed for a single axis point, the new value represents the new width. If any of the operations contains thePOINT_INDEX
property, with an integer value, then this editor will only perform the operation on that particular point, otherwise it will perform it on the entire point list.- Specified by:
editImpl
in classALspEditor
- Parameters:
aOperation
- the event that contains the information on how to edit the objectaInteractionStatus
- the interaction statusaContext
- the edit context- Returns:
- The result of the operation: Whether or not there was success, and whether or not the current handles of the object should be invalidated.
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcd3DEditableVariableGeoBuffer
, as described in the class javadoc.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle that is used to initialize the state of the object being
created, or
null
if it should not be possible to create the object. - See Also:
-