Class TLsp3DPointListEditor
java.lang.Object
com.luciad.view.lightspeed.editor.ALspEditor
com.luciad.view.lightspeed.editor.TLsp3DPointListEditor
- All Implemented Interfaces:
ILspEditor
- Direct Known Subclasses:
TLspLonLatHeightBufferEditor
Enables visual editing of
ILcd3DEditablePointList
objects in an
ILspView
.
Note that the discussion of the editing behaviour below focuses on how a 3D point list is edited
in a 3D view. In a 2D view, editing a 3D point list works the same as when using a
TLsp2DPointListEditor
.
Handles
The 3D point list editor defines the following edit handles for a 3D point list object:- 3D point position handles: allows the user to edit the positions of points in the point list. The
user can change the XY position of the points by dragging the handle that is projected on the earth. This handle
generates
MOVE
operations, with an XY constraint, and aPOINT_INDEX
property. - 3D point height handles: Allows the user to edit the height (Z-value) of the individual points in the point
list, by dragging the handle. This handle generates
MOVE
operations, with a Z constraint, and a and aPOINT_INDEX
property. - Object translation handle: allows the user to translate the 3D point list object.
In a 2D view, this handle is activated by pressing and dragging the shape itself. In a 3D view,
this handle is drawn on the terrain as the base shape of the 3D point list. (the base shape is the shape
that is projected on the earth). This handle
generates
MOVE
operations, with an XY constraint, without any additional properties.
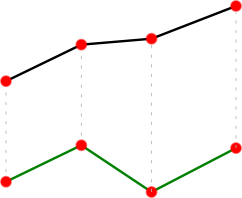
Editing
Based on theTLspEditOperation
, generated by an edit handle (see description
of handles above), this editor performs different edit operations on the associated 3D point
list object. The images below illustrate the effect of the different handles. In each image the
gray color represents the previous state of the object and the red color represents the edited object:
-
3D point handle
NOTE: only part of the new state (red) is drawn to avoid cluttering the image. -
Object translation handle
Creation
The creation process of a 3D point list object consists of a series of two distinct steps:- the first step initializes the XY location of the next axis point
- the second step initializes the height of that point
![]() |
![]() |
![]() |
![]() |
|||
Setting the XY location of the first point | Setting the height of the first point | Setting the XY location of the second point | Setting the height of the second point |
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Defines keys used by the enclosing editor implementation to store properties in its edit handles. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionboolean
canEdit
(TLspEditContext aContext) protected ALspEditHandle
createInsertPointHandle
(ILcd2DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to insert points into the given point list.protected ALspEditHandle
createObjectTranslationHandle
(ILcd3DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given point list horizontally (i.e. parallel to the terrain).protected ALspEditHandle
createPointHeightTranslationHandle
(ILcd3DEditablePointList aPointList, int aPointIndex, TLspEditContext aContext) Creates a point height handle.protected ALspEditHandle
createPointPositionHandle
(ILcd3DEditablePointList aPointList, int aPointIndex, TLspEditContext aContext) Creates a point position handle.protected ALspEditHandle
createRemovePointHandle
(ILcd2DEditablePointList aPointList, int aIndex, TLspEditContext aContext) Creates a point removal handle for the point with indexaIndex
of the given point list.protected TLspEditOperationResult
editImpl
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.Methods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
canCopyGeometry, canPerformOperation, copyGeometrySFCT, edit, fireUndoableHappened
-
Constructor Details
-
TLsp3DPointListEditor
public TLsp3DPointListEditor()Creates a newTLsp3DPointListEditor
. This constructor does not initialize any state.
-
-
Method Details
-
canEdit
- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations: If any of the operations contains thePOINT_INDEX
property, with an integer value, then this editor will only perform the operation on that particular point, otherwise it will perform it on the entire point list.- Specified by:
editImpl
in classALspEditor
- Parameters:
aOperation
- the event that contains the information on how to edit the objectaInteractionStatus
-aContext
- the edit context- Returns:
SUCCESS
if the above conditions are met,FAILED
otherwise. The invalidation hint will be set if a point was added or removed.
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this method delegates to the following methods: It returns a list containing the handles returned by those methods. These methods are added for convenience, so they can easily be overridden. Note that for the point handles, it delegates multiple times to those methods with different indices. By default this method returns a list that is twice the size, plus one, of the size of the pointlist.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createInsertPointHandle
protected ALspEditHandle createInsertPointHandle(ILcd2DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to insert points into the given point list.- Parameters:
aPointList
- the point list for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createRemovePointHandle
protected ALspEditHandle createRemovePointHandle(ILcd2DEditablePointList aPointList, int aIndex, TLspEditContext aContext) Creates a point removal handle for the point with indexaIndex
of the given point list. APOINT_INDEX
user property with the given index as value is set on the handle.- Parameters:
aPointList
- the point list for which the handle is returnedaIndex
- the index of the point in the point list, for which the handle is createdaContext
- the current context- Returns:
- a handle that allows the user to remove the point with given index, or
null
if no handle is needed
-
createPointHeightTranslationHandle
protected ALspEditHandle createPointHeightTranslationHandle(ILcd3DEditablePointList aPointList, int aPointIndex, TLspEditContext aContext) Creates a point height handle. Override this method to change the behavior of point height handles, or make it returnnull
to disable creation of point height handles. By default this method returns aTLspObjectHeightTranslationHandle
. APOINT_INDEX
user property with the given index as value is set on the handle.- Parameters:
aPointList
- the pointlist for which to create a point height handleaPointIndex
- the index of the point for which to create a point height handleaContext
- edit context information- Returns:
- An edit handle to change the height of a point, or
null
if this handle should not be created.
-
createPointPositionHandle
protected ALspEditHandle createPointPositionHandle(ILcd3DEditablePointList aPointList, int aPointIndex, TLspEditContext aContext) Creates a point position handle. By default this will be an instance ofTLspPointTranslationHandle
, with a user property containing the index of the point. Override this method to change the behavior of point position handles, or make it returnnull
to disable creation of point position handles.- Parameters:
aPointList
- the pointlist for which to create a point position handleaPointIndex
- the index of the point for which to create a point position handleaContext
- edit context information- Returns:
- An edit handle to change the position of a point, or
null
if this handle should not be created.
-
createObjectTranslationHandle
protected ALspEditHandle createObjectTranslationHandle(ILcd3DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given point list horizontally (i.e. parallel to the terrain).Note that by default this method returns a different handle depending on whether the view is a 2D- or a 3D view: in the 2D case, a
TLspObjectTranslationHandle
is returned, otherwise aTLspProjectedObjectTranslationHandle
is returned.- Parameters:
aPointList
- the point list for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
when no handle is needed
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcd3DEditablePointList
, as described in the class javadoc.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle, or
null
if creation should not be allowed. - See Also:
-