Class TLspExtrudedShapeEditor
- All Implemented Interfaces:
ILspEditor
ILcd2DEditablePointList
objects as well as
ILcdEditableExtrudedShape
with a base shape of type ILcd2DEditablePointList
is:
TLspCompositeEditor compositeEditor = new TLspCompositeEditor(); compositeEditor.addEditor(new TLspExtrudedShapeEditor(compositeEditor)); compositeEditor.addEditor(new TLsp2DPointListEditor());
Note that the shape editor
is already a
composite editor containing all LuciadLightspeed editors, including the extruded shape editor.
Handles
This editor defines the following as handles for an ILcdEditableExtrudedShape
object:
- Base shape handles: these are the handles that are provided by the base shape editor and allow the user to edit the base shape of the extruded shape. They are drawn on top of the terrain and function the same as when they are used to edit a 2D shape.
- Minimum height handle: only for extruded shapes in a 3D view. Allows the user to edit
the minimum height of the extruded shape. This handle generates
MOVE
operations, with aHANDLE_IDENTIFIER
property andMINIMUM_HEIGHT
as value. - Maximum height handle: only for extruded shapes in a 3D view. Allows the user to edit
the maximum height of the extruded shape. This handle generates
MOVE
operations, with aHANDLE_IDENTIFIER
property andMAXIMUM_HEIGHT
as value. - Height translation handle: only for extruded shapes in a 3D view. Allows the user to
edit the height of the extruded shape above the terrain, which boils down to simultaneously
changing the minimum- and maximum height of the extruded shape. This handle generates
MOVE
operations, with aHANDLE_IDENTIFIER
property andHEIGHT
as value. - Projected Shape handle: only for extruded shapes in a 3D view. Allows the user to
translate the extruded shape horizontally (i.e. parallel to the terrain). This handle is painted
as the base shape on the terrain and can be activated by pressing on that base shape. This handle
generates
MOVE
operations, without properties. These operations are delegated to the base shape editor.
ILcdEditableExtrudedShape
object with an ILcd2DEditableEllipse
object as base shape : the base shape handles
are indicates as red dots, the green area represents the projected shape handle, the blue
dots represent the min- and max height handle and finally the extruded shape itself represents
the height translation handle.
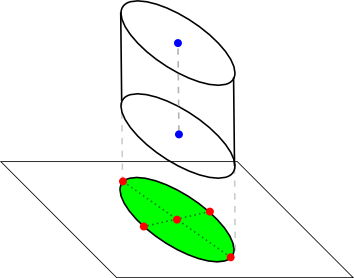
Editing
Based on theTLspEditOperation
, generated by an edit handle (see description
of handles above), this editor performs different edit operations on the associated extruded shape.
The images below illustrate the effect of the different handles. In each image the
gray color represents the previous state of the object and the red color represents the edited object:
-
Base shape handle: the editor adjusts the base shape by delegating to the set base
shape editor.
In this figure the minor axis handle of the ellipse base shape is edited.
-
Minimum height handle: 3D only, modifies the minimum height of the extruded shape.
-
Maximum height handle: 3D only, modifies the maximum height of the extruded shape.
-
Height translation handle: 3D only, modifies the height of the extruded shape.
-
Projected shape handle: 3D only, moves the shape parallel to the terrain.
Creation
The initialization process of an extruded shape consists of two distinct steps:- first the base shape is initialized at the initial height.
- when in a 3D view, the next step defines the maximum height of the extruded shape
![]() |
![]() |
|
Creating the base shape | Initializing the height of the extruded shape |
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Describes the type of an edit handle created by the enclosing editor implementation.static enum
Defines keys used by the enclosing editor implementation to store properties in its edit handles. -
Constructor Summary
ConstructorsConstructorDescriptionCreates a newTLspExtrudedShapeEditor
.TLspExtrudedShapeEditor
(ILspEditor aBaseEditor) Creates a new extruded shape editor with the given base shape editor. -
Method Summary
Modifier and TypeMethodDescriptionboolean
canCopyGeometry
(Object aSourceGeometry, Object aDestinationGeometry) Tests whether this editor can apply the properties of a template source object to the given target object.boolean
canEdit
(TLspEditContext aContext) Determines whether or not thegeometry inside the given context
can be edited by this editor.boolean
canPerformOperation
(TLspEditOperation aOperation, TLspEditContext aContext) Determines whether or not the editor knows how to apply the given edit operation to the supplied geometry.void
copyGeometrySFCT
(Object aSourceGeometry, Object aDestinationGeometrySFCT) Method that takes the given source object and applies all geometry to the destination object.protected ALspEditHandle
createHeightHandle
(ILcdEditableExtrudedShape shape, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the minimum- and maximum height of the given extruded shape simultaneously by dragging the shape up/down.protected ALspEditHandle
createMaximumHandle
(ILcdEditableExtrudedShape shape, ILcdPoint basePoint, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the maximum height of the given extruded shape by dragging it up/down.protected ALspEditHandle
createMinimumHandle
(ILcdEditableExtrudedShape shape, ILcdPoint basePoint, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the minimum height of the given extruded shape by dragging it up/down.protected ALspEditHandle
Creates an edit handle that allows the user to translate the given extruded shape in 2D (i.e. parallel to the terrain).edit
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Applies an interaction obtained from a handle to the object being edited.protected TLspEditOperationResult
editImpl
(TLspEditOperation aEvent, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.Gets the editor that is used to edit or create the base shape of an extruded shape.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.double
The initial minimal and maximal z-value of a created extruded shape is set to this value.void
setInitialZValue
(double aInitialZValue) Set the initial minimal and maximal z-value used when creating new extruded shapes to the given value.Methods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
fireUndoableHappened
-
Constructor Details
-
TLspExtrudedShapeEditor
public TLspExtrudedShapeEditor()Creates a newTLspExtrudedShapeEditor
. This constructor does not initialize any state. When using this constructor, it will not be possible to edit the base shape, only its height. -
TLspExtrudedShapeEditor
Creates a new extruded shape editor with the given base shape editor.- Parameters:
aBaseEditor
- the editor that will be used to edit the base shapes
-
-
Method Details
-
getInitialZValue
public double getInitialZValue()The initial minimal and maximal z-value of a created extruded shape is set to this value. The default value is set to 10000.- Returns:
- the initial minimal and maximal z-value of a created extruded shape
-
setInitialZValue
public void setInitialZValue(double aInitialZValue) Set the initial minimal and maximal z-value used when creating new extruded shapes to the given value.- Parameters:
aInitialZValue
- the initial minimal and maximal z-value that a new extruded shape will be created with.
-
getBaseShapeEditor
Gets the editor that is used to edit or create the base shape of an extruded shape.- Returns:
- the base shape editor
-
canEdit
Determines whether or not thegeometry inside the given context
can be edited by this editor. Override this method to provide a custom implementation. The default implementation determines whether the given object is cloneable. This editor can only edit cloneable objects. By default, this implementation determines whether the given object is cloneable and if the given object is an instance ofILcdEditableExtrudedShape
.- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
edit
public TLspEditOperationResult edit(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Applies an interaction obtained from a handle to the object being edited.Note: Only override this method if you want to bypass the undo/redo support provided by this abstract class. For most use cases, it is preferred to override
By default, this editor can handle the following operations: If any of the operations contains theeditImpl
instead.HANDLE_IDENTIFIER
property, with aHandleIdentifier
as value, then this editor will only perform theMOVE
operation on that particular point. Further this implementation delegates to the base shape editor.Note: Only override this method if you want to bypass the undo/redo support provided by this abstract class. For most use cases, it is preferred to override
editImpl
instead.- Specified by:
edit
in interfaceILspEditor
- Overrides:
edit
in classALspEditor
- Parameters:
aOperation
- describes the edit that should occur. This is usually generated by the handles. It is up to the concrete editor to determine how to handle this event.aInteractionStatus
-aContext
- the edit context @return the result of the edit operation- Returns:
- information about the result of the operation
- See Also:
-
canPerformOperation
Determines whether or not the editor knows how to apply the given edit operation to the supplied geometry. By default this method returnstrue
if the model reference of the edit operation is equal to that of the edited object. By default this method returnstrue
if the model reference of the edit operation is equal to that of the edited object.- Specified by:
canPerformOperation
in interfaceILspEditor
- Overrides:
canPerformOperation
in classALspEditor
- Parameters:
aOperation
- the operation to be appliedaContext
- the edit context- Returns:
- true if the model reference of the edit operation is equal to that of the edited object
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcdExtrudedShape
, as described in the class javadoc. If the given object is not anILcdExtrudedShape
, this method returns a create handle that is capable of creating the base shape only.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle, or
null
if creation should not be allowed. - See Also:
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this method delegates to the base shape editor. It also delegates to the following methods: These methods are added for convenience, so they can easily be overridden.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createMinimumHandle
protected ALspEditHandle createMinimumHandle(ILcdEditableExtrudedShape shape, ILcdPoint basePoint, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the minimum height of the given extruded shape by dragging it up/down.- Parameters:
shape
- the extruded shape for which the handle is createdbasePoint
- the base point which provides the XY location of the resulting handleaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createHeightHandle
protected ALspEditHandle createHeightHandle(ILcdEditableExtrudedShape shape, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the minimum- and maximum height of the given extruded shape simultaneously by dragging the shape up/down.- Parameters:
shape
- the extruded shape for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createMaximumHandle
protected ALspEditHandle createMaximumHandle(ILcdEditableExtrudedShape shape, ILcdPoint basePoint, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the maximum height of the given extruded shape by dragging it up/down.- Parameters:
shape
- the extruded shape for which the handle is createdbasePoint
- the base point which provides the XY location of the resulting handleaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createProjectedShapeTranslationHandle
protected ALspEditHandle createProjectedShapeTranslationHandle(ILcdEditableExtrudedShape shape, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given extruded shape in 2D (i.e. parallel to the terrain). This handle is visualized by painting the base shape on the terrain. Dragging the base shape around will also move the extruded shape.- Parameters:
shape
- the extruded shape for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aEvent, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations: If any of the operations contains theHANDLE_IDENTIFIER
property, with aHandleIdentifier
as value, then this editor will only perform theMOVE
operation on that particular point. Further this implementation delegates to the base shape editor.- Specified by:
editImpl
in classALspEditor
- Parameters:
aEvent
- the event that contains the information on how to edit the objectaInteractionStatus
- the interaction statusaContext
- the edit context- Returns:
- The result of the operation: Whether or not there was success, and whether or not the current handles of the object should be invalidated.
-
canCopyGeometry
Description copied from class:ALspEditor
Tests whether this editor can apply the properties of a template source object to the given target object. This method is not allowed to modify the parameters of the given objects.- Overrides:
canCopyGeometry
in classALspEditor
- Parameters:
aSourceGeometry
- a template source object from which the geometry will be copied fromaDestinationGeometry
- a destination object to which the geometry will be copied to- Returns:
- true if a call to
copyGeometrySFCT
would succeed, false otherwise. - See Also:
-
copyGeometrySFCT
Description copied from class:ALspEditor
Method that takes the given source object and applies all geometry to the destination object.
This method is called when performing an undo or redo operation. Before editing an object, a clone is created from the geometry. When performing e.g. an undo operation, the state of the clone is applied on the geometry object using this method. Something similar happens for a redo operation.
By default, this method supports all default (editable) shapes in LuciadLightspeed. Custom editors should override this method to allow this editor to apply changes to the original object given a modified instance of it.
- Overrides:
copyGeometrySFCT
in classALspEditor
- Parameters:
aSourceGeometry
- A template source object to copy the geometry from.aDestinationGeometrySFCT
- A destination object to copy the geometry to.
-