Class TLspRulerController
- All Implemented Interfaces:
ILcdUndoableSource
,ILcdAWTEventListener
,ILspController
A controller that allows to measure distances on the map.
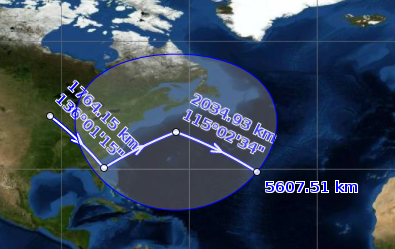
The measurement information is displayed on a label with the polyline as illustrated in the figure above.
The polylines can be edited after creation.
As such, TLspRulerController
is very similar to the TLspCreateController
and TLspEditController
.
The created polylines are instances of ALspRulerMeasurement
.
The ruler measurement class exposes the distances (in meters) and azimuths (in degrees) calculated by the ruler controller.
You can access the last measurement in the controller itself.
You can also register a change listener to be notified when the current polyline changes.
It is possible to customize various aspects of this controller:
- Control the styling of the lines with
setLineStyler(com.luciad.view.lightspeed.style.styler.ILspStyler)
. - Control the styling of the radius circles with
setCircleStyler(com.luciad.view.lightspeed.style.styler.ILspCustomizableStyler)
andsetDisplayEqualDistanceCircles(boolean)
. - Control the styling of the labels (including the distance unit) with
setLabelStyler(com.luciad.view.lightspeed.style.styler.ILspStyler)
, passing aTLspRulerLabelStyler
. - Control the styling of the editing handles with
setHandleStyler(com.luciad.view.lightspeed.style.styler.ILspStyler)
andsetFocusHandleStyler(com.luciad.view.lightspeed.style.styler.ILspStyler)
- Set the measurement mode using
setMeasureMode(com.luciad.view.lightspeed.controller.ruler.TLspRulerController.MeasureMode)
- Specify whether to keep measurements using
setKeepLayer(boolean)
andsetKeepMeasurements(boolean)
. - Control the distance and azimuth calculations by overriding the
calculateAzimuth(com.luciad.shape.ILcdPoint, com.luciad.shape.ILcdPoint, com.luciad.model.ILcdModelReference, com.luciad.view.lightspeed.controller.ruler.TLspRulerController.MeasureMode)
orcalculateDistance(com.luciad.shape.ILcdPoint, com.luciad.shape.ILcdPoint, com.luciad.model.ILcdModelReference, com.luciad.view.lightspeed.controller.ruler.TLspRulerController.MeasureMode)
methods.
The handleFXEvent(Event aEvent) converts the native JavaFX TouchEvent
to a TLcdTouchEvent
and passes it to the ALspController.handleAWTEvent(AWTEvent aEvent).
snappable layers
.- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Enumeration that describes how the distances and azimuths are calculated. -
Constructor Summary
ConstructorsConstructorDescriptionCreates a new ruler controller.TLspRulerController
(boolean aAddLayerToView) Creates a new ruler controller.TLspRulerController
(boolean aAddLayerToView, ALspCreateControllerModel aCreateControllerModel) Creates a new ruler controller with the givenALspCreateControllerModel
. -
Method Summary
Modifier and TypeMethodDescriptionvoid
addChangeListener
(ILcdChangeListener aListener) Adds a change listener.void
addUndoableListener
(ILcdUndoableListener aUndoableListener) Adds a listener to this source, so this listener is notified when something undoable has happened.protected double
calculateAzimuth
(ILcdPoint aPoint1, ILcdPoint aPoint2, ILcdModelReference aReference, TLspRulerController.MeasureMode aMeasureMode) This method is called to calculate the azimuth for a segment of a ruler measurement.protected double
calculateDistance
(ILcdPoint aPoint1, ILcdPoint aPoint2, ILcdModelReference aReference, TLspRulerController.MeasureMode aMeasureMode) This method is called to calculate the distance of a segment of a ruler measurement.void
cancel()
Cancels the creation of the ruler polyline.void
finish()
Finish the creation of the ruler polyline.Returns the customizable styler currently used to determine the style of equal distance circles.Returns the current measurement.Returns the mouse cursor for this controller.Returns the styler for focused editing handles.Returns the styler for editing handles.Returns the styler for distance and azimuth labels of ruler measurements that are being edited or created.getLabelStyler
(TLspPaintState aPaintState) Returns the styler for distance and azimuth labels of ruler measurements for measurements in the given paintstate.Returns anILcdLayered
for this controller.Returns the styler for ruler polylines that are being edited or created.Returns the current measurement mode.Returns theILcdStringTranslator
set on this controller.handleAWTEventImpl
(AWTEvent aAWTEvent) Called byhandleAWTEvent
.void
handleFXEvent
(Event aEvent) Delegates tohandleFXEventImpl
.handleFXEventImpl
(Event aEvent) Called byhandleFXEvent
.boolean
Returns true if a layer containing the measurements is added to the view (and as such visible in the layer tree).boolean
Returns whether or not the ruler controller is currently creating or editing.boolean
Returns whether or not azimuths are displayed for each segment.boolean
Returns true if equal distance circles are displayed for polyline nodes that are moved.boolean
Returns true if the layer is kept after the interaction with the ruler controller is terminated.boolean
Returns true if measurements are kept after a new measurement is started.paintImpl
(ILcdGLDrawable aGLDrawable, ILspView aView, TLspPaintPhase aPaintPhase) The specific implementation ofpaint
for this controller.void
removeChangeListener
(ILcdChangeListener aListener) Removes a change listener if it is present.void
removeUndoableListener
(ILcdUndoableListener aUndoableListener) Removes the specified listener so it is no longer notified.void
setCircleStyler
(ILspCustomizableStyler aCircleStyler) Sets the customizable styler currently used to paint equal distance circles.void
setDisplayAzimuth
(boolean aDisplayAzimuth) Sets whether or not azimuths are displayed for each segment.void
setDisplayEqualDistanceCircles
(boolean aDisplayEqualDistanceCircles) Sets whether or not equal distance circles are displayed for polyline nodes that are moved.void
setFocusHandleStyler
(ILspStyler aFocusHandleStyler) Sets the styler for focused editing handles.void
setHandleStyler
(ILspStyler aHandleStyler) Sets the styler for editing handles.void
setKeepLayer
(boolean aKeepLayer) Set to true if the layer is to be kept after the interaction with the ruler controller is terminated.void
setKeepMeasurements
(boolean aKeepMeasurements) Set to true is measurements should be kept after a new measurement is started.void
setLabelStyler
(TLspPaintState aState, ILspStyler aLabelStyler) Sets the styler for distance and azimuth labels, for the given paint state.void
setLabelStyler
(ILspStyler aLabelStyler) Sets the styler for distance and azimuth labels, for all supported paint states.void
setLineStyler
(TLspPaintState aPaintState, ILspStyler aLineStyler) Sets the styler for the ruler polylines for the given paint state.void
setLineStyler
(ILspStyler aLineStyler) Sets the styler for the ruler polylines, for all supported paint states.void
setMeasureMode
(TLspRulerController.MeasureMode aMeasureMode) Sets the measurement mode.void
setStringTranslator
(ILcdStringTranslator aStringTranslator) Sets theILcdStringTranslator
that this controller should use to translate theStrings
that will be visible in the user interface.void
startInteractionImpl
(ILspView aView) The specific implementation ofstartInteraction
for this controller.void
terminateInteractionImpl
(ILspView aView) The specific implementation ofterminateInteraction
for this controller.Methods inherited from class com.luciad.view.lightspeed.controller.ALspController
addPropertyChangeListener, addStatusListener, appendController, firePropertyChange, fireStatusEvent, getAWTFilter, getFXCursor, getFXFilter, getIcon, getName, getNextController, getShortDescription, getView, handleAWTEvent, paint, registerViewPropertyNameForReset, removePropertyChangeListener, removeStatusListener, setAWTFilter, setCursor, setFXCursor, setFXFilter, setIcon, setName, setShortDescription, startInteraction, terminateInteraction
-
Constructor Details
-
TLspRulerController
public TLspRulerController()Creates a new ruler controller. Equivalent to
.new TLspRulerController(false, null)
-
TLspRulerController
public TLspRulerController(boolean aAddLayerToView) Creates a new ruler controller. Based on the passed value, the ruler measurements will be painted in a layer added to the view, or in a layer offered by thelayered
of this controller. If the layer is added to the view, it is possible to keep it, even if the interaction with this controller is terminated. Equivalent to
.new TLspRulerController(aAddLayerToView, null)
- Parameters:
aAddLayerToView
- true to add layer in view, false otherwise.
-
TLspRulerController
public TLspRulerController(boolean aAddLayerToView, ALspCreateControllerModel aCreateControllerModel) Creates a new ruler controller with the givenALspCreateControllerModel
. Use this constructor to:- let the ruler controller create custom
ALspRulerMeasurement
implementations - limit the number of points the user can create measurements with
- be notified when a new measurement is created
- be notified when measurement creation is canceled
- Parameters:
aAddLayerToView
- true to add layer in view, false otherwise. SeeTLspRulerController(boolean)
for more information.aCreateControllerModel
- The ruler controller uses this to create new measurements. When null, the ruler controller will use a defaultALspCreateControllerModel
implementation. Note that itsgetLayer
implementation must returnnull
. Ifnull
is returned from itscreate
, then a default measurement will be created.- Since:
- 2018.0
- let the ruler controller create custom
-
-
Method Details
-
paintImpl
public TLspPaintProgress paintImpl(ILcdGLDrawable aGLDrawable, ILspView aView, TLspPaintPhase aPaintPhase) Description copied from class:ALspController
The specific implementation ofpaint
for this controller.- Overrides:
paintImpl
in classALspController
- Parameters:
aGLDrawable
- the drawable that should be painted onaView
- the view that is painted onaPaintPhase
- the current paint phase- Returns:
- whether or not the painting was finished
-
startInteractionImpl
Description copied from class:ALspController
The specific implementation ofstartInteraction
for this controller.- Overrides:
startInteractionImpl
in classALspController
- Parameters:
aView
- the view to start interaction with.
-
terminateInteractionImpl
Description copied from class:ALspController
The specific implementation ofterminateInteraction
for this controller.- Overrides:
terminateInteractionImpl
in classALspController
- Parameters:
aView
- the view to terminate interaction with.
-
handleAWTEventImpl
Description copied from class:ALspController
Called byhandleAWTEvent
. Returnsnull
when the controller consumed the event or a partially consumed event when the controller partially consumed the event (which could happen withTLcdTouchEvent
s). When the controller did not use the given event, it is returned unaltered.- Specified by:
handleAWTEventImpl
in classALspController
- Parameters:
aAWTEvent
- the event to be handled.- Returns:
null
when the input event was consumed, the (possibly modified) input event when it was (partially) consumed.
-
handleFXEvent
Description copied from class:ALspController
Delegates tohandleFXEventImpl
. If that method does not returnnull
, the result is passed on to the next controller in the chain (if one exists). If the controller has a filter that does not accept the event, the event is not delegated and is immediately passed on to the next controller.- Specified by:
handleFXEvent
in interfaceILspController
- Overrides:
handleFXEvent
in classALspController
- Parameters:
aEvent
- the event to be handled
-
handleFXEventImpl
Description copied from class:ALspController
Called byhandleFXEvent
. Returnsnull
when the controller consumed the event. When the controller did not use the given event, it is returned unaltered.- Overrides:
handleFXEventImpl
in classALspController
- Parameters:
aEvent
- the event to be handled.- Returns:
null
when the input event was consumed, the (possibly modified) input event when it was (partially) consumed.
-
setStringTranslator
Sets the
ILcdStringTranslator
that this controller should use to translate theStrings
that will be visible in the user interface.This method should be called before this controller is used. Any
Strings
already created by this controller will not be translated with the specified translator. TheILcdStringTranslator
does not translate the controller's name and description.- Parameters:
aStringTranslator
- TheILcdStringTranslator
that should be used. Must not benull
.The following list of
Strings
are translated by the given instance:- "Clear measurements"
- "Undo {0}".
{0}
will be replaced by the display name of the action that is to be undone. E.g. "Undo Clear measurements". - "Redo {0}".
{0}
will be replaced by the display name action that is to be redone. E.g. "Redo Clear measurements" - "Edit {0}".
{0}
will be replaced by the result of callingObject.toString()
on the edited object. E.g. "Edit ruler measurement". - "Create object"
- The result of calling
Object.toString()
on the usedALspMeasurement
objects. For the defaultALspMeasurement
implementation, this is "ruler measurement".
ILcdStringTranslator
needs to be able to translate what is passed in{0}
, but not the entire formattedString
. For example, for "Undo {0}" it needs to be able to translate "Undo {0}" itself and "Clear measurements", but not "Undo Clear measurements".
-
getStringTranslator
Returns the
ILcdStringTranslator
set on this controller.- Returns:
- The translator set on this controller. Never
null
.
-
getCurrentMeasurement
Returns the current measurement. This is either the measurement currently under construction or the last created or edited measurement.- Returns:
- the current measurement.
-
addChangeListener
Adds a change listener. This listener will be informed when the current measurement is changed.- Parameters:
aListener
- the listener that should be added.- See Also:
-
removeChangeListener
Removes a change listener if it is present.- Parameters:
aListener
- the listener that should be removed.- See Also:
-
isAddLayerToView
public boolean isAddLayerToView()Returns true if a layer containing the measurements is added to the view (and as such visible in the layer tree).- Returns:
- true if the measurement layer is added to the view, false otherwise.
- See Also:
-
isKeepLayer
public boolean isKeepLayer()Returns true if the layer is kept after the interaction with the ruler controller is terminated. This will only have effect is the layer isadded
to the view. Otherwise this method will have no effect.- Returns:
- true if the layer is kept after the interaction with the ruler controller is terminated.
- See Also:
-
setKeepLayer
public void setKeepLayer(boolean aKeepLayer) Set to true if the layer is to be kept after the interaction with the ruler controller is terminated. For this to work, the ruler controller must be configured toadd a layer to the view
. The default value isfalse
.- Parameters:
aKeepLayer
- True to keep layer in view, false otherwise.- See Also:
-
isKeepMeasurements
public boolean isKeepMeasurements()Returns true if measurements are kept after a new measurement is started.- Returns:
- true if measurements are kept.
- See Also:
-
setKeepMeasurements
public void setKeepMeasurements(boolean aKeepMeasurements) Set to true is measurements should be kept after a new measurement is started. Default is false.- Parameters:
aKeepMeasurements
- True to keep measurements, false otherwise.- See Also:
-
getLayered
Description copied from interface:ILspController
Returns an
ILcdLayered
for this controller. Custom implementations of this method should take care to return the sameILcdLayered
instance every time this method is called to guarantee correct working of theILcdLayered
interface.The returned layered object should contain layers that are relevant to this controller. Any view using this controller should take into account the layers of its active controller.
Note that the ILcdLayered is only allowed to contain
ILspLayer
instances.- Specified by:
getLayered
in interfaceILspController
- Overrides:
getLayered
in classALspController
- Returns:
- an ILcdLayered object, or null if this controller has no layers.
-
getMeasureMode
Returns the current measurement mode. The default mode isMEASURE_GEODETIC
.- Returns:
- the current measurement mode
-
setMeasureMode
Sets the measurement mode. The mode must be one ofMEASURE_GEODETIC
,MEASURE_RHUMB
orMEASURE_CARTESIAN
.- Parameters:
aMeasureMode
- the new measurement mode
-
getLineStyler
Returns the styler for ruler polylines that are being edited or created.- Returns:
- the current styler used by the polyline painter.
- See Also:
-
setLineStyler
Sets the styler for the ruler polylines, for all supported paint states. The objects to provide styles for extendALspRulerMeasurement
and implementILcdPolyline
.- Parameters:
aLineStyler
- the styler to be used by the polyline painter.- See Also:
-
setLineStyler
Sets the styler for the ruler polylines for the given paint state. The objects to provide styles for extendALspRulerMeasurement
and implementILcdPolyline
.- Parameters:
aPaintState
- the paintstate to set the styler for.aLineStyler
- the styler to be used by the polyline painter.- See Also:
-
isDisplayEqualDistanceCircles
public boolean isDisplayEqualDistanceCircles()Returns true if equal distance circles are displayed for polyline nodes that are moved. Currently this is only relevant forMEASURE_GEODETIC
.- Returns:
- true if equal distance circles are displayed.
-
setDisplayEqualDistanceCircles
public void setDisplayEqualDistanceCircles(boolean aDisplayEqualDistanceCircles) Sets whether or not equal distance circles are displayed for polyline nodes that are moved. Currently this is only relevant for measure modesMEASURE_GEODETIC
. When another measure mode is used no circles will be displayed. Default is true.- Parameters:
aDisplayEqualDistanceCircles
- true if equal distance circles have to be displayed, false otherwise.
-
getCircleStyler
Returns the customizable styler currently used to determine the style of equal distance circles.- Returns:
- the current styler used by the circle painter.
- See Also:
-
setCircleStyler
Sets the customizable styler currently used to paint equal distance circles. The customizable styler is in effect treated as a list of styles. All enabled customizable styles that are relevant will be used when styling the circles.- Parameters:
aCircleStyler
- the styler to be used by the circle painter.- See Also:
-
getLabelStyler
Returns the styler for distance and azimuth labels of ruler measurements that are being edited or created. This is equivalent to callinggetLabelStyler(TLspPaintState.EDITED)
.- Returns:
- the current styler used by the label painter.
- See Also:
-
getLabelStyler
Returns the styler for distance and azimuth labels of ruler measurements for measurements in the given paintstate.- Parameters:
aPaintState
- the paintstate to get the label styler for.- Returns:
- the current styler used by the label painter.
- See Also:
-
setLabelStyler
Sets the styler for distance and azimuth labels, for all supported paint states. The objects to provide styles for extendALspRulerMeasurement
and implementILcdPolyline
. By default a standardTLspRulerLabelStyler
is used. The styler will be used to paint measurements in the REGULAR, EDITED and SELECTED paintstates.- Parameters:
aLabelStyler
- the styler to be used by the label painter.- See Also:
-
setLabelStyler
Sets the styler for distance and azimuth labels, for the given paint state. The objects to provide styles for extendALspRulerMeasurement
and implementILcdPolyline
. By default a standardTLspRulerLabelStyler
is used.- Parameters:
aState
- the paintstate to get the styler foraLabelStyler
- the styler to be used by the label painter.- See Also:
-
setHandleStyler
Sets the styler for editing handles. By default, a standardTLspEditHandleStyler
is used.- Parameters:
aHandleStyler
- The styler to be used for editing handles.
-
getHandleStyler
Returns the styler for editing handles. By default, this is a standardTLspEditHandleStyler
.- Returns:
- The styler for editing handles.
-
setFocusHandleStyler
Sets the styler for focused editing handles. By default, a standardTLspEditHandleStyler
with a red icon style for points, is used.- Parameters:
aFocusHandleStyler
- The styler to be used for focused editing handles.
-
getFocusHandleStyler
Returns the styler for focused editing handles. By default, a standardTLspEditHandleStyler
with a red icon style for points, is used.- Returns:
- The styler for focused editing handles.
-
isDisplayAzimuth
public boolean isDisplayAzimuth()Returns whether or not azimuths are displayed for each segment.- Returns:
- whether or not azimuths are displayed for each segment.
- See Also:
-
setDisplayAzimuth
public void setDisplayAzimuth(boolean aDisplayAzimuth) Sets whether or not azimuths are displayed for each segment. When set totrue
, an azimuth label is shown for every segment. Note that this method is a convenience method; it works when the ruler label styler is not customized. More specifically, it works under the following conditions:- the set label styler is a customizable styler
- one of the customizable styles in this styler is a
TLspRulerSegmentLabelContentStyle
.
TLspRulerSegmentLabelContentStyle.Builder#displayAzimuth
is used to create a newTLspRulerSegmentLabelContentStyle
that is capable of returning the current azimuth value. On top of that, calling this method also adds an arrow to the middle of every line segment. Note that this is a convenience method as well. It works by default, or when the used line styler- doesn't call
ALspStyleCollector#geometry
. - uses one or more
TLspLineStyle
objects.
- Parameters:
aDisplayAzimuth
- whether or not azimuths are displayed for each segment- See Also:
-
addUndoableListener
Description copied from interface:ILcdUndoableSource
Adds a listener to this source, so this listener is notified when something undoable has happened.- Specified by:
addUndoableListener
in interfaceILcdUndoableSource
- Parameters:
aUndoableListener
- The listener to be notified when something undoable has happened.
-
removeUndoableListener
Description copied from interface:ILcdUndoableSource
Removes the specified listener so it is no longer notified.- Specified by:
removeUndoableListener
in interfaceILcdUndoableSource
- Parameters:
aUndoableListener
- The listener to remove.
-
calculateAzimuth
protected double calculateAzimuth(ILcdPoint aPoint1, ILcdPoint aPoint2, ILcdModelReference aReference, TLspRulerController.MeasureMode aMeasureMode) This method is called to calculate the azimuth for a segment of a ruler measurement. The default implementation returns an azimuth depending on the measure mode as follows:MEASURE_GEODETIC
: the two dimensional forward azimuth between the two points, according to the ellipsoid the model reference is based on (seeILcdEllipsoid.forwardAzimuth2D
).MEASURE_RHUMB
: the two dimensional rhumbline azimuth between the two points, according to the ellipsoid the model reference is based on (seeILcdEllipsoid.rhumblineAzimuth2D
).MEASURE_CARTESIAN
:Double.NaN
, there is no reliable way to calculate an azimuth for a line in world space.
- Parameters:
aPoint1
- the first point of the segment to calculate the azimuth foraPoint2
- the second point of the segment to calculate the azimuth foraReference
- the reference the points are defined in.aMeasureMode
- the measure mode to take into account.- Returns:
- an azimuth for the passed segment, of
Double.NaN
if no azimuth could be calculated.
-
calculateDistance
protected double calculateDistance(ILcdPoint aPoint1, ILcdPoint aPoint2, ILcdModelReference aReference, TLspRulerController.MeasureMode aMeasureMode) This method is called to calculate the distance of a segment of a ruler measurement. The default implementation returns an distance depending on the measure mode as follows:MEASURE_GEODETIC
: the geodetic distance between the two points, according to the ellipsoid the model reference is based on (seeILcdEllipsoid.geodesicDistance
).MEASURE_RHUMB
: the rhumbline distance between the two points, according to the ellipsoid the model reference is based on (seeILcdEllipsoid.rhumblineDistance
).MEASURE_CARTESIAN
: the cartesian distance between the two points (seeTLcdCartesian.distance3D
), taking into account the unit of measure of the model reference (in this case the world reference of the view) if available.
- Parameters:
aPoint1
- the first point of the segment to calculate the distance foraPoint2
- the second point of the segment to calculate the distance foraReference
- the reference the points are defined in.aMeasureMode
- the measure mode to take into account.- Returns:
- an distance for the passed segment
-
isCreating
public boolean isCreating()Returns whether or not the ruler controller is currently creating or editing.- Returns:
- true if a ruler polyline is being created, false otherwise.
-
finish
public void finish()Finish the creation of the ruler polyline. -
cancel
public void cancel()Cancels the creation of the ruler polyline. -
getCursor
Description copied from class:ALspController
Returns the mouse cursor for this controller. The cursor is typically retrieved by the view to configure its host component.
This method returns the cursor for this controller, not the rest of chain. However, the property change listeners of the controller will be informed about cursor changes for the entire controller chain. A cursor property change event's value will be the first non-null cursor along the controller chain. For JavaFX, see {#linkALspController.getFXCursor()
}.- Overrides:
getCursor
in classALspController
- Returns:
- the cursor for this controller, or null if the controller does not have a specific cursor
- See Also:
-