Class TLcdGXYComplexStroke
Stroke
implementation that can be used to draw one or more patterns repetitively along a path. Each
pattern is defined by a java.awt.Shape
, and has a pattern width. This width can be different from its
Shape
s' width, to allow for gaps between repeated patterns.
The stroke algorithm moves a pivot point over the segments of the path and, each time the pivot is moved, it draws the next pattern on the current pivot position, such that:
- the pivot point of the shape pattern (its origin) coincides with the current pivot position
- the base line of of the shape pattern (the X axis) is aligned with the current path segment (unless the path segment is too short, see below).
Whenever the pattern width is too small to fit in (the last part of ) the current path segment, the algorithm will try to fit the patterns
as good as possible by cutting off the corners of the segments. A tolerance can be specified to control how large
the cut-off error is allowed to be. If the error is too large, a fallback stroke will be used to paint the remaining part
of the path segment, the pivot will be moved to the next segment and the algorithm will try to continue from the new start point. In case the fallback stroke is null
,
these parts of the path won't be stroked. If the last part of the shape's path is too small to fit the next pattern,
the fallback stroke will also be used to draw this part.
The example below illustrates briefly how the algorithm works; two patterns and a basic fallback stroke are defined. The first row with shapes shows how the algorithm works when the tolerance level is not exceeded; notice that one of the corners has been cut-off and that the last part of the path is drawn using the fallback stroke (shown here in a different color, for clarity). The first image of the second row shows the errors that are introduced by cutting off corners, the last two images illustrate how these errors are avoided when the tolerance is decreased, by painting parts of the path using the fallback stroke.
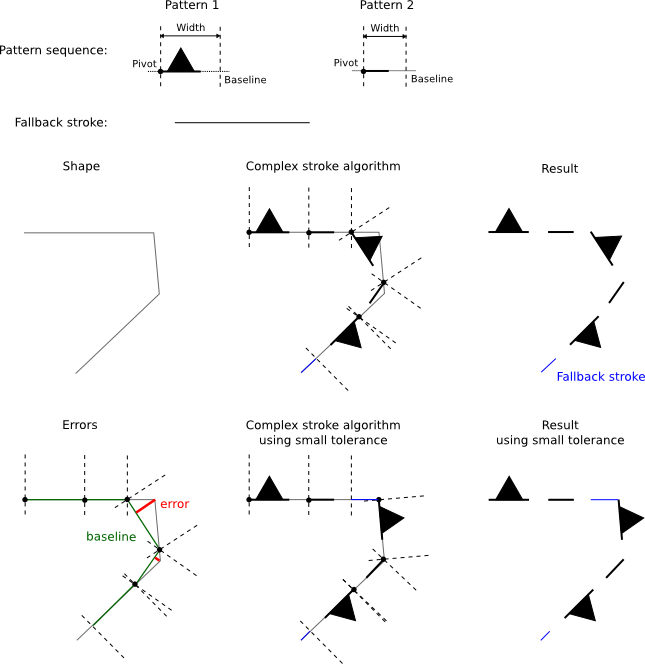
When multiple patterns are used, a flag can be passed to the constructor to force the stroke algorithm to paint either full sequences or nothing. This can be useful when the pattern sequence is only meaningful when drawn completely (for example, when the patterns are the characters of a word).
Known issues and limitations
- Strokes can only be drawn in the color that is configured on the
Graphics
. A multi-colored stroke can be achieved by defining one stroke for each color, and painting the shape multiple times, each time with a different stroke and its corresponding color. - The stroke algorithm does not know anything about the Graphics clip; drawing very long lines exceeding the Graphics clip can result in very slow rendering performance (the algorithm will scan the full line, not only the visible part). An additional constructor with a clip can be used in this case to improve performance.
TLcdStrokeLineStyle
.
- Since:
- 8.0
-
Constructor Summary
ConstructorsConstructorDescriptionTLcdGXYComplexStroke
(TLcdGXYComplexStroke aStroke, Rectangle aClip) Constructs a new complex stroke that is identical to the given one, but with another clipping area.TLcdGXYComplexStroke
(Shape[] aPatterns, double[] aPatternWidths, boolean aAllowSplit) Constructs a new complex stroke, initialized with the given pattern sequence and pattern widths.TLcdGXYComplexStroke
(Shape[] aPatterns, double[] aPatternWidths, boolean[] aAllowPatternRotations, boolean aAllowSplit, Stroke aFallbackStroke, double aTolerance, Rectangle aClip) Constructs a new complex stroke, initialized with the given pattern sequence, pattern widths, pattern rotation settings, fallback stroke and tolerance.TLcdGXYComplexStroke
(Shape[] aPatterns, double[] aPatternWidths, boolean aAllowSplit, Stroke aFallbackStroke, double aTolerance) Constructs a new complex stroke, initialized with the given pattern sequence, pattern widths, fallback stroke and tolerance.TLcdGXYComplexStroke
(Shape[] aPatterns, double[] aPatternWidths, boolean aAllowSplit, Stroke aFallbackStroke, double aTolerance, Rectangle aClip) Constructs a new complex stroke, initialized with the given pattern sequence, pattern widths, fallback stroke and tolerance.TLcdGXYComplexStroke
(Shape aPattern, double aPatternWidth) Constructs a new complex stroke, initialized with the given pattern and pattern width.TLcdGXYComplexStroke
(Shape aPattern, double aPatternWidth, Stroke aFallbackStroke, double aTolerance) Constructs a new complex stroke, initialized with the given pattern, pattern width, fallback stroke and tolerance.TLcdGXYComplexStroke
(Shape aPattern, double aPatternWidth, Stroke aFallbackStroke, double aTolerance, Rectangle aClip) Constructs a new complex stroke, initialized with the given pattern, pattern width, fallback stroke and tolerance. -
Method Summary
-
Constructor Details
-
TLcdGXYComplexStroke
Constructs a new complex stroke, initialized with the given pattern and pattern width.The stroke will be configured with a
BasicStroke
of width 1 as fallback stroke, and a tolerance of 5 pixels.- Parameters:
aPattern
- a closed, fillable shape to be used as a pattern.aPatternWidth
- the width of a single pattern.
-
TLcdGXYComplexStroke
public TLcdGXYComplexStroke(Shape aPattern, double aPatternWidth, Stroke aFallbackStroke, double aTolerance) Constructs a new complex stroke, initialized with the given pattern, pattern width, fallback stroke and tolerance.- Parameters:
aPattern
- a closed, fillable shape to be used as a pattern.aPatternWidth
- the width of a single pattern.aFallbackStroke
- the stroke that should be used if the error introduced by using a pattern would be larger than the allowed tolerance.aTolerance
- the error that is allowed, this is the maximum allowed distance between the base line of the pattern and the real path, expressed in pixels.
-
TLcdGXYComplexStroke
public TLcdGXYComplexStroke(Shape aPattern, double aPatternWidth, Stroke aFallbackStroke, double aTolerance, Rectangle aClip) Constructs a new complex stroke, initialized with the given pattern, pattern width, fallback stroke and tolerance.A rectangular clip is provided outside which no stroking should be performed. If a clip is set, all pivot point positions will be computed correctly, including the pivots for the path segments outside the clip, but shape patterns will only be generated if they intersect with the clip.
Setting a clip should be considered when the
TLcdGXYComplexStroke
is not performant enough. This typically happens in zooming operations: when zooming in on a shape, the path segments defining the shape can become very long (although only a small subset of the path is visible on screen), and, unless the stroke knows which part is visible, it can only apply the stroke on the full path, including the (potentially very large) parts outside the visible area.- Parameters:
aPattern
- a closed, fillable shape to be used as a pattern.aPatternWidth
- the width of a single pattern.aFallbackStroke
- the stroke that should be used if the error introduced by using a pattern would be larger than the allowed tolerance.aTolerance
- the error that is allowed, this is the maximum allowed distance between the base line of the pattern and the real path, expressed in pixels.aClip
- the clip bounds within which stroking should be applied. Ifnull
, no clipping will be performed.
-
TLcdGXYComplexStroke
Constructs a new complex stroke, initialized with the given pattern sequence and pattern widths.The stroke will be configured with a
BasicStroke
of width 1 as fallback stroke, and a tolerance of 5 pixels.- Parameters:
aPatterns
- closed, fillable shapes to be used as a pattern.aPatternWidths
- the widths of the patterns.aAllowSplit
- flag indicating whether a path segment is allowed to end with a subset of the full pattern sequence. Set tofalse
when the pattern sequence is only meaningful when drawn completely.
-
TLcdGXYComplexStroke
public TLcdGXYComplexStroke(Shape[] aPatterns, double[] aPatternWidths, boolean aAllowSplit, Stroke aFallbackStroke, double aTolerance) Constructs a new complex stroke, initialized with the given pattern sequence, pattern widths, fallback stroke and tolerance.- Parameters:
aPatterns
- closed, fillable shapes to be used as a pattern.aPatternWidths
- the widths of the patterns.aAllowSplit
- iffalse
, the resulting strokes will only contain complete sequences of patterns. Iftrue
, a stroke can end with a partial pattern sequence.aFallbackStroke
- the stroke that should be used if the error introduced by using a pattern would be larger than the allowed tolerance.aTolerance
- the error that is allowed, this is the maximum allowed distance between the base line of the pattern and the real path, expressed in pixels.
-
TLcdGXYComplexStroke
Constructs a new complex stroke that is identical to the given one, but with another clipping area.- Parameters:
aStroke
- The stroke to be copied.aClip
- The clip to be used by this stroke. Ifnull
, no clipping will be performed.
-
TLcdGXYComplexStroke
public TLcdGXYComplexStroke(Shape[] aPatterns, double[] aPatternWidths, boolean aAllowSplit, Stroke aFallbackStroke, double aTolerance, Rectangle aClip) Constructs a new complex stroke, initialized with the given pattern sequence, pattern widths, fallback stroke and tolerance.A rectangular clip is provided outside which no stroking should be performed. If a clip is set, all pivot point positions will be computed correctly, including the pivots for the path segments outside the clip, but shape patterns will only be generated if they intersect with the clip.
Setting a clip should be considered when the
TLcdGXYComplexStroke
is not performant enough. This typically happens in zooming operations: when zooming in on a shape, the path segments defining the shape can become very long (although only a small subset of the path is visible on screen), and, unless the stroke knows which part is visible, it can only apply the stroke on the full path, including the (potentially very large) parts outside the visible area.- Parameters:
aPatterns
- closed, fillable shapes to be used as a pattern.aPatternWidths
- the widths of the patterns.aAllowSplit
- iffalse
, the resulting strokes will only contain complete sequences of patterns. Iftrue
, a stroke can end with a partial pattern sequence.aFallbackStroke
- the stroke that should be used if the error introduced by using a pattern would be larger than the allowed tolerance.aTolerance
- the error that is allowed, this is the maximum allowed distance between the base line of the pattern and the real path, expressed in pixels.aClip
- the clip bounds within which stroking should be applied. Ifnull
, no clipping will be performed.
-
TLcdGXYComplexStroke
public TLcdGXYComplexStroke(Shape[] aPatterns, double[] aPatternWidths, boolean[] aAllowPatternRotations, boolean aAllowSplit, Stroke aFallbackStroke, double aTolerance, Rectangle aClip) Constructs a new complex stroke, initialized with the given pattern sequence, pattern widths, pattern rotation settings, fallback stroke and tolerance.A rectangular clip is provided outside which no stroking should be performed. If a clip is set, all pivot point positions will be computed correctly, including the pivots for the path segments outside the clip, but shape patterns will only be generated if they intersect with the clip.
Setting a clip should be considered when the
TLcdGXYComplexStroke
is not performant enough. This typically happens in zooming operations: when zooming in on a shape, the path segments defining the shape can become very long (although only a small subset of the path is visible on screen), and, unless the stroke knows which part is visible, it can only apply the stroke on the full path, including the (potentially very large) parts outside the visible area.- Parameters:
aPatterns
- closed, fillable shapes to be used as a pattern.aPatternWidths
- the widths of the patterns.aAllowPatternRotations
- if an entry isfalse
, the corresponding pattern will never be rotated. Iftrue
, the pattern is rotated along the stroke. Ifnull
, * all patterns will be rotated.aAllowSplit
- iffalse
, the resulting strokes will only contain complete sequences of patterns. Iftrue
, a stroke can end with a partial pattern sequence.aFallbackStroke
- the stroke that should be used if the error introduced by using a pattern would be larger than the allowed tolerance.aTolerance
- the error that is allowed, this is the maximum allowed distance between the base line of the pattern and the real path, expressed in pixels.aClip
- the clip bounds within which stroking should be applied. Ifnull
, no clipping will be performed.- Since:
- 2019.0
-
-
Method Details
-
createStrokedShape
- Specified by:
createStrokedShape
in interfaceStroke
-
equals
Returnstrue
if the specified object is aTLcdGXYComplexStroke
, equal to this object. Two complex strokes are considered equal if they have equal pattern sequences, pattern widths, splitting policy, fallback stroke, tolerance and clip. -
hashCode
public int hashCode()Returns the hashcode of this object.
-