Class TLcdVisibilityMatrixViewFactory
Creates an ILcdVisibilityMatrixView
object representing the visibility between two ILcdAltitudeMatrixView
objects. For example, the visibility from a polyline to a polygon. These
computations are based on a point-to-point propagation function describing the visibility between
two points.
The main method createVisibilityMatrixView
creates
the ILcdVisibilityMatrixView
and computes which points inside the second matrix view
(To
) can be seen from the first matrix view (From
). The From
argument represents the view information from where the other view is looked at. The To
argument represents the view information at which the other view is looking. The visibility matrix
view is created using the protected method createVisibilityMatrixView
.
This class is designed according to the Template design pattern (see "Design Patterns", Gamma et al., p. 325). The behavior of the implemented algorithm can be adapted by subclassing this class and overriding one or more of the protected methods that implement parts of the algorithm. The rounded rectangles in the figure below indicate all steps that can be customized via overriding (meaning: each rounded rectangle corresponds to one protected method that can be overridden).
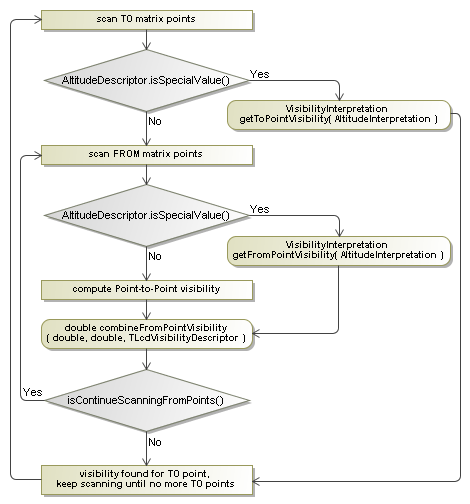
The flow chart shows how the visibility is computed and how the protected factory methods are used.
All To
points are scanned as they are part of the visibility result and thus required
to contain a visibility value. For regular values, the From
matrix view is scanned and
the combined visibility is computed. For special values, the required visibility value is found by
calling the method getToPointVisibility
.
When scanning the From
points, a visibility value is required for all From
points. For regular points, the result of the point-to-point intervisibility computation between the
From
point and the To
point is used. For special points, the method getFromPointVisibility
provides the visibility value. The From
point scanning can be stopped prematurely depending on the method isContinueScanningFromPoints
. The default implementation uses the visibility interpretation VISIBLE
as stop condition. The scanning of the From
points is therefore stopped when at least one point is found from which the processed To
point is visible.
The visibility interpretations of all From
points, for a given To
point,
are combined into one visibility interpretation for that To
point (see the method combineFromPointVisibility(TLcdVisibilityInterpretation, TLcdVisibilityInterpretation)
). The default
implementation uses the following priority order for combining the visibility interpretations (highest
first):
VISIBLE |
UNCERTAIN |
INVISIBLE + other interpretations, except OUTSIDE_SHAPE |
For example, if one
From
point has the visibility interpretation INVISIBLE
,
and another has visibility VISIBLE
, the combined result will be VISIBLE
. Once
there is a From
point having visibility VISIBLE
, the combined result for the
To
point will always remain VISIBLE
. VISIBLE
is therefore used
as the default stop condition (see isContinueScanningFromPoints
).
The visibility OUTSIDE_SHAPE
is not taken into account (ignored).
As clarified by the image below, the From
view argument can contain points from where
one cannot look. The red dots in the image represent points outside the shape, the point-to-point
intervisibility computation from one of these points to the To
matrix view should not
be incorporated in the resulting visibility value. The default implementation skips these points,
based on the OUTSIDE_SHAPE
interpretation, in
the combineFromPointVisibility
method.
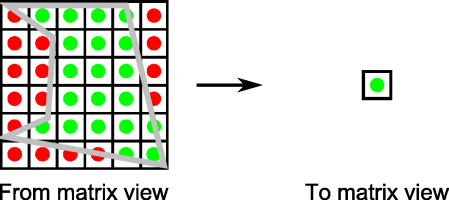
When, on the other hand, the To
view argument contains points which are represented by
the interpretation OUTSIDE_SHAPE
, a visibility result is required for these points in
the visibility view. The visibility descriptor provides a visibility result for these points, that
are represented by the red dots in the To
view.
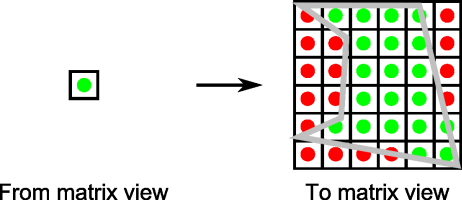
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs a new visibility matrix view factory. -
Method Summary
Modifier and TypeMethodDescriptionvoid
addStatusListener
(ILcdStatusListener aStatusListener) Starts notifying a listener with the progress of the visibility calculations.protected double
combineFromPointVisibility
(double aPreviousValue, double aCurrentValue, TLcdVisibilityDescriptor aVisibilityDescriptor) Combines the specified visibility values depending on their interpretation according to the given visibility descriptor.protected TLcdVisibilityInterpretation
combineFromPointVisibility
(TLcdVisibilityInterpretation aPreviousInterpretation, TLcdVisibilityInterpretation aCurrentInterpretation) Combines the specified visibility interpretations.createVisibilityMatrixView
(ILcdAltitudeMatrixView aFromAltitudeMatrixView, ILcdAltitudeMatrixView aToAltitudeMatrixView, double aStepSize, ILcdP2PPropagationFunction aP2PPropagationFunction, TLcdVisibilityDescriptor aVisibilityDescriptor) Creates anILcdVisibilityMatrixView
containing the result of the matrix-to-matrix visibility computation.protected ILcdEditableVisibilityMatrixView
createVisibilityMatrixView
(ILcdAltitudeMatrixView aAltitudeMatrixView, TLcdVisibilityDescriptor aVisibilityDescriptor) Creates anILcdEditableVisibilityMatrixView
using the specified altitude matrix view.protected TLcdVisibilityInterpretation
getFromPointVisibility
(TLcdAltitudeInterpretation aInterpretation) Returns the visibility interpretation corresponding to the specified altitude interpretation.protected TLcdVisibilityInterpretation
getToPointVisibility
(TLcdAltitudeInterpretation aInterpretation) Returns the visibility interpretation corresponding to the specified altitude interpretation.protected boolean
isContinueScanningFromPoints
(double aCurrentValue, TLcdVisibilityDescriptor aVisibilityDescriptor) Returns whether the algorithm should keep scanning theFrom
matrix view or not.void
removeStatusListener
(ILcdStatusListener aStatusListener) Terminates notifying a listener of the progress of the visibility calculations.
-
Constructor Details
-
TLcdVisibilityMatrixViewFactory
public TLcdVisibilityMatrixViewFactory()Constructs a new visibility matrix view factory.
-
-
Method Details
-
addStatusListener
Starts notifying a listener with the progress of the visibility calculations.- Parameters:
aStatusListener
- will be notified of progress of the visibility calculations.- See Also:
-
removeStatusListener
Terminates notifying a listener of the progress of the visibility calculations.- Parameters:
aStatusListener
- will no longer be notified of progress of the visibility calculations.- See Also:
-
createVisibilityMatrixView
public ILcdVisibilityMatrixView createVisibilityMatrixView(ILcdAltitudeMatrixView aFromAltitudeMatrixView, ILcdAltitudeMatrixView aToAltitudeMatrixView, double aStepSize, ILcdP2PPropagationFunction aP2PPropagationFunction, TLcdVisibilityDescriptor aVisibilityDescriptor) Creates an
ILcdVisibilityMatrixView
containing the result of the matrix-to-matrix visibility computation. The method computes which points inside the second altitude matrix view can be seen from the first altitude matrix view and stores the result into anILcdVisibilityMatrixView
, created using thecreateVisibilityMatrixView
method.The method will only store the result of the visibility computations in the resulting matrix view and will not adjust the associated points of the created visibility matrix view. The values are described by the specified
TLcdVisibilityDescriptor
.- Parameters:
aFromAltitudeMatrixView
- The matrix view to look from.aToAltitudeMatrixView
- The matrix view to look at.aStepSize
- The intermediate step size used for a point-to-point computation.aP2PPropagationFunction
- The propagation function used for a point-to-point computation.aVisibilityDescriptor
- Describes the resulting visibility matrix view.- Returns:
- an
ILcdVisibilityMatrixView
containing the result of the matrix-to-matrix visibility computations. - Throws:
IllegalArgumentException
- when the argumentaFromAltitudeMatrixView
oraToAltitudeMatrixView
uses theABOVE_OBJECT
altitude mode.
-
-
createVisibilityMatrixView
protected ILcdEditableVisibilityMatrixView createVisibilityMatrixView(ILcdAltitudeMatrixView aAltitudeMatrixView, TLcdVisibilityDescriptor aVisibilityDescriptor) Creates an
ILcdEditableVisibilityMatrixView
using the specified altitude matrix view. The resulting matrix view should be consistent with the specified altitude matrix view, meaning that the column count, row count and the associated point coordinates of both matrices need to be the same.The default implementation creates a visibility matrix view which delegates the associated point methods to the specified altitude matrix view. This means that a reference to the altitude matrix view is stored in the returned visibility matrix view. If this is not wanted, the method should be overridden to return a custom implementation.
The method initializes the matrix with the visibility value, corresponding to the interpretation
NOT_COMPUTED
.The resulting visibility matrix view is used in the main factory method
createVisibilityMatrixView
, which will store the actual matrix values. Note that the latter method will only adjust the matrix values, the associated point coordinates will not be updated.- Parameters:
aAltitudeMatrixView
- The altitude matrix view for which the visibility is needed.aVisibilityDescriptor
- Describes the resulting visibility matrix view.- Returns:
- an
ILcdEditableVisibilityMatrixView
using the specified altitude matrix view.
-
getFromPointVisibility
protected TLcdVisibilityInterpretation getFromPointVisibility(TLcdAltitudeInterpretation aInterpretation) Returns the visibility interpretation corresponding to the specified altitude interpretation. This method is called when processing the
From
altitude matrix view.The default implementation returns
TLcdVisibilityInterpretation.OUTSIDE_SHAPE
when the specified interpretation isTLcdAltitudeInterpretation.OUTSIDE_SHAPE
, otherwiseTLcdVisibilityInterpretation.UNCERTAIN
is returned.- Parameters:
aInterpretation
- The altitude interpretation to convert.- Returns:
- the visibility interpretation corresponding to the specified altitude interpretation.
-
combineFromPointVisibility
protected double combineFromPointVisibility(double aPreviousValue, double aCurrentValue, TLcdVisibilityDescriptor aVisibilityDescriptor) Combines the specified visibility values depending on their interpretation according to the given visibility descriptor. This method is used while processing the
From
altitude matrix view and is called for all points in theFrom
matrix view.The default implementation converts the two visibility values to visibility interpretations using the specified visibility descriptor. These interpretations are then used as input for the method
combineFromPointVisibility(TLcdVisibilityInterpretation, TLcdVisibilityInterpretation)
to compute a combined visibility interpretation. The combined visibility interpretation is converted to a visibility value that is returned by this method. Note that this means that both values need to be special visibility values according to the specified visibility descriptor, if not anIllegalArgumentException
shall be thrown. In the default implementation both values will be special.- Parameters:
aPreviousValue
- The previous combined visibility value.aCurrentValue
- The current visibility value to add.aVisibilityDescriptor
- Describes the visibility values.- Returns:
- the combined visibility value.
-
combineFromPointVisibility
protected TLcdVisibilityInterpretation combineFromPointVisibility(TLcdVisibilityInterpretation aPreviousInterpretation, TLcdVisibilityInterpretation aCurrentInterpretation) Combines the specified visibility interpretations. This method is called from the method
combineFromPointVisibility
.The default implementation returns the previous visibility interpretation when one of the following conditions is met. In all other cases, the current visibility interpretation is returned.
- The current interpretation is equal to
OUTSIDE_SHAPE
. - The previous interpretation is equal to
UNCERTAIN
and the current interpretation is notVISIBLE
.
- Parameters:
aPreviousInterpretation
- The previous combined visibility interpretation.aCurrentInterpretation
- The current visibility interpretation to add.- Returns:
- the combined visibility interpretation.
- The current interpretation is equal to
-
isContinueScanningFromPoints
protected boolean isContinueScanningFromPoints(double aCurrentValue, TLcdVisibilityDescriptor aVisibilityDescriptor) Returns whether the algorithm should keep scanning the
From
matrix view or not. This method implements a stop condition when a specific visibility value is found.The default implementation returns
true
if the visibility value is not special or if the visibility interpretation corresponding to the special visibility value differs fromTLcdVisibilityInterpretation.VISIBLE
, otherwisefalse
is returned.- Parameters:
aCurrentValue
- The current visibility value.aVisibilityDescriptor
- Describes the visibility values.- Returns:
- whether the algorithm should keep scanning the
From
matrix view or not.
-
getToPointVisibility
protected TLcdVisibilityInterpretation getToPointVisibility(TLcdAltitudeInterpretation aInterpretation) Returns the visibility interpretation corresponding to the specified altitude interpretation. This method is called when processing the
TO
altitude matrix view.The default implementation returns
TLcdVisibilityInterpretation.OUTSIDE_SHAPE
when the specified interpretation isTLcdAltitudeInterpretation.OUTSIDE_SHAPE
, otherwiseTLcdVisibilityInterpretation.UNCERTAIN
is returned.- Parameters:
aInterpretation
- The altitude interpretation to convert.- Returns:
- the visibility interpretation corresponding to the specified altitude interpretation.