Class TLspRadarVideoStyle
java.lang.Object
com.luciad.view.lightspeed.style.ALspStyle
com.luciad.realtime.lightspeed.radarvideo.TLspRadarVideoStyle
- All Implemented Interfaces:
ILspStyler
Style that configures the visualization of a
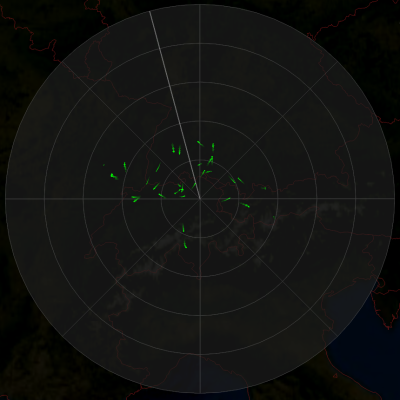
What follows is an overview of the elements of the radar display and how they
can be configured using this style.
Background
The circular area covered by the radar is filled in a constant color as given
by Detected objects
Objects detected by the radar (also referred to as "blips") are colored using
If a blip is not "confirmed" by a subsequent pass of the radar (i.e. an object that was previously detected appears to be no longer there), the blip changes from the blip color to
The blip/afterglow color is modulated by the amplitude of the blip, i.e. blips with a high amplitude will be more clearly visible. A blip with maximal amplitude will always have the exact blip color specified by this style.Improving the "readability" of the display
The
radar video layer
.
Radar video styles can be created using the supplied TLspRadarVideoStyle.Builder
.
The following screenshot is an example of what a radar video layer might display:
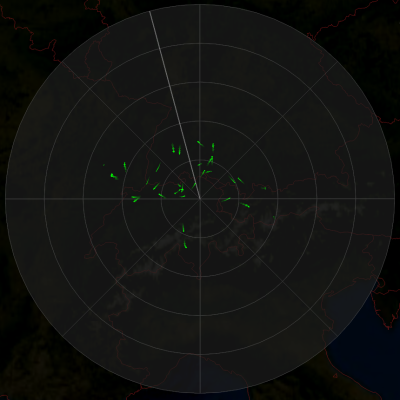
Background 
The circular area covered by the radar is filled in a constant color as given
by getBackgroundColor()
(dark gray in the screenshot). It is possible to
use a translucent color so that any background data is visible through the
radar feed.
Detected objects 
Objects detected by the radar (also referred to as "blips") are colored using
getBlipColor()
(green in the screenshot). After the sweep has passed
them, blips begin to fade into the background color over a time interval determined by
getBlipAfterglow()
.
If a blip is not "confirmed" by a subsequent pass of the radar (i.e. an object that was previously detected appears to be no longer there), the blip changes from the blip color to
getBlipAfterglowColor()
. This allows the user to visually
distinguish "fresh" blips from ones that are only still visible due to afterglow.
If the afterglow color is set to null, the blip color is always used and this
visual distinction will not be made.
The blip/afterglow color is modulated by the amplitude of the blip, i.e. blips with a high amplitude will be more clearly visible. A blip with maximal amplitude will always have the exact blip color specified by this style.
Improving the "readability" of the display 
The getIntensity()
property can be used to bias the intensity of the
blips and bring out more contrast in the display. The default value is 1.
Higher values increase the intensity of the blips, lower values decrease it.
The intensity setting has no effect on blips which already have maximal
amplitude; it only makes blips with a lower amplitude appear brighter.
If the radar signal contains a lot of noise, getAmplitudeThreshold()
can be used to filter out cells which produced only a low amplitude. The threshold
is a normalized value: 0 means that all non-zero amplitudes are displayed, and
1 means that only cells which have the highest possible amplitude are kept.
The radar video feed can also be antialiased. This reduces "shimmering" effects
for feeds that have a lot of noise or other high-frequency detail. Antialiasing
incurs a performance penalty, obviously, and is therefore configurable by a
quality hint
.
- Since:
- 2014.1
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Mode that determines how theafterglow duration
is interpreted.static enum
Mode that determines the quality of the antialiasing applied to radar video feeds.static final class
Builder used to createTLspRadarVideoStyle
objects. -
Method Summary
Modifier and TypeMethodDescriptionCreates a new builder initialized with all the properties of this style.boolean
double
Returns the minimum amplitude required for a blip to be displayed, normalized into [0, 1] range.Returns the amount of antialiasing to be applied to the radar video feed.Returns the background color used to visualize the circular area covered by the radar.double
Returns the afterglow duration of the blips.Returns the afterglow color used to visualize objects that were not detected by the last pass of the radar but which are still visible due to afterglow.Determines the interpretation of the blip afterglow time.Returns the blip color used to visualize the detected objects.double
Returns the intensity of the blips.int
hashCode()
boolean
Determines whether this style is transparent.static TLspRadarVideoStyle.Builder
<?> Creates a new builder with the default values.Methods inherited from class com.luciad.view.lightspeed.style.ALspStyle
addStyleChangeListener, getZOrder, isCompatible, removeStyleChangeListener, style
-
Method Details
-
newBuilder
Creates a new builder with the default values.- Returns:
- the new builder.
-
asBuilder
Creates a new builder initialized with all the properties of this style. -
getBlipColor
Returns the blip color used to visualize the detected objects.- Returns:
- the blip color
-
getBlipAfterglowColor
Returns the afterglow color used to visualize objects that were not detected by the last pass of the radar but which are still visible due to afterglow. If the afterglow color is set to null, the blip color is used (so current blips cannot be visually distinguished from fading ones).- Returns:
- the afterglow color
-
getBackgroundColor
Returns the background color used to visualize the circular area covered by the radar.- Returns:
- the background color
-
getBlipAfterglow
public double getBlipAfterglow()Returns the afterglow duration of the blips. This is used to fade the blips over time. After the time specified, the blip will completely disappear. The interpretation of the afterglow duration depends on theafterglow mode
. It is either measured in seconds or as the number of full 360 degree sweeps of the radar.- Returns:
- the afterglow duration of the blips in seconds
-
getBlipAfterglowMode
Determines the interpretation of the blip afterglow time. If set toTLspRadarVideoStyle.AfterglowMode.ABSOLUTE
, theafterglow
is measured in seconds. If set toTLspRadarVideoStyle.AfterglowMode.RELATIVE
, the afterglow is defined as a number of full 360 degree sweeps of the radar.- Returns:
- the interpretation of the blip afterglow duration
-
getAmplitudeThreshold
public double getAmplitudeThreshold()Returns the minimum amplitude required for a blip to be displayed, normalized into [0, 1] range. This can be used to filter out cells which produced only a low amplitude.- Returns:
- the minimum displayed blip amplitude
-
getIntensity
public double getIntensity()Returns the intensity of the blips. Higher values increase the intensity of the blips, lower values decrease it. This can be used to bias the intensity of the blips and bring out more contrast in the display.- Returns:
- the intensity of the blips
-
getAntialiasingHint
Returns the amount of antialiasing to be applied to the radar video feed. Antialiasing can reduce visual artifacts when displaying high-frequency data at low zoom levels, but incurs a performance cost. Since noise can also be filtered out at no cost by changing theamplitude threshold
, antialiasing is disabled by default. This should be fine for most data, but you can enable antialiasing if you don't want to use the amplitude threshold or if that alone does not solve all aliasing artifacts for your data.- Returns:
- the amount of antialiasing applied to the data
-
isTransparent
public boolean isTransparent()Description copied from class:ALspStyle
Determines whether this style is transparent.Shapes that are transparent can be painted in a different paint phase than shapes that are not transparent.
- Specified by:
isTransparent
in classALspStyle
- Returns:
- whether or not this style is transparent
-
equals
-
hashCode
public int hashCode()
-