You can add balloons to your Map
for a specific layer by configuring a BalloonContentProvider
on the layer.
The default behavior of the Map
is to display a balloon when a user selects an object of a layer with a balloonContentProvider
.
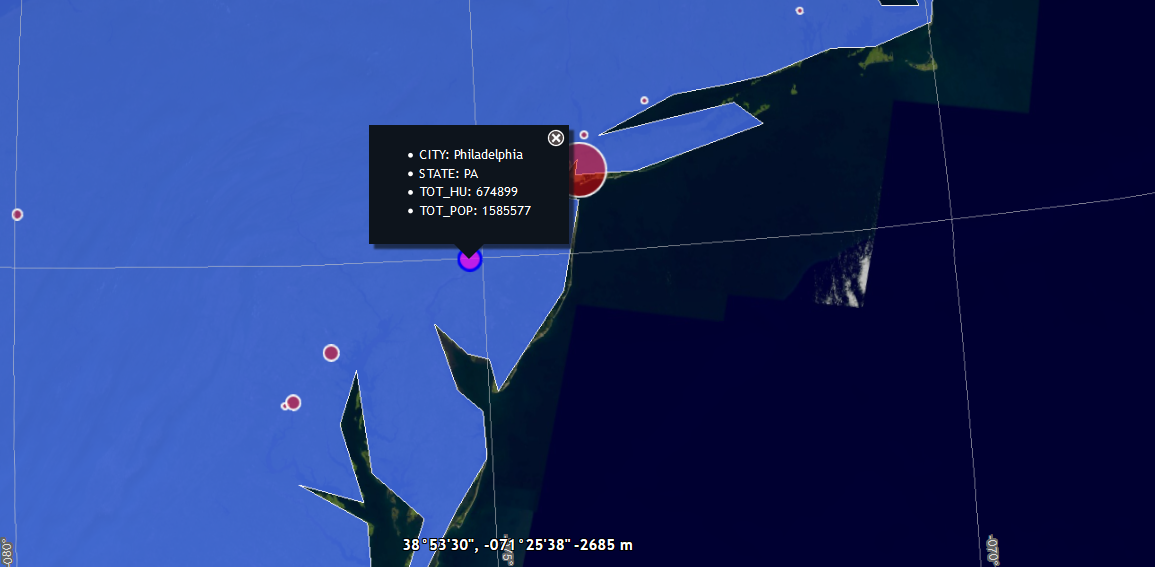
Figure 1. The Balloon sample
In this example, extracted from the balloons sample, we create a BalloonContentProvider
that:
-
Displays the name of the city for small cities
-
For large cities with a population of over one million, displays an HTML list with more information about the city.
Program: Setting a
balloonContentProvider
on a layer with city features. (from samples/balloon/main.tsx
)
function contentProvider(prefix: string, property: string): (o: Feature | Shape) => string | HTMLElement {
return (o): string | HTMLElement => prefix + ((o as Feature).properties[property] || "no property");
}
// Create a balloon content provider for the cities layer, which creates HTML
// for cities with more than 1.000.000 inhabitants and uses the "default"
// content provider if not.
const citiesDefaultProvider = contentProvider("CITY: ", "CITY");
citiesLayer.balloonContentProvider = (o: Feature | Shape): string | HTMLElement => {
const cityProps = (o as Feature).properties;
let node;
if (cityProps.TOT_POP > 1000000) {
node = document.createElement("ul", {});
for (const key in cityProps) {
if (cityProps.hasOwnProperty(key) && key !== "uid") {
const item = document.createElement("li");
item.innerHTML = `${key}: ${cityProps[key]}`;
node.appendChild(item);
}
}
return node;
} else {
return citiesDefaultProvider(o);
}
};