Class TLspFrustum
java.lang.Object
com.luciad.view.lightspeed.geometry.TLspFrustum
Describes a view frustum: a volume delimited by six planes. A frustum can
represent the volume of space that is visible in an
ILspView
, and
can hence be used to perform culling of invisible objects. To this effect,
this class contains overlap tests for the frustum with shapes such as points
and bounds.
The figure below shows a schematic view of a frustum. The frustum itself is defined by the thick
lines. With relation to a scene that is drawn in an ILspView
,
all objects that are located inside the volume defined by those thick lines, will appear on the
screen. All the objects that are outside these bounds will be culled.
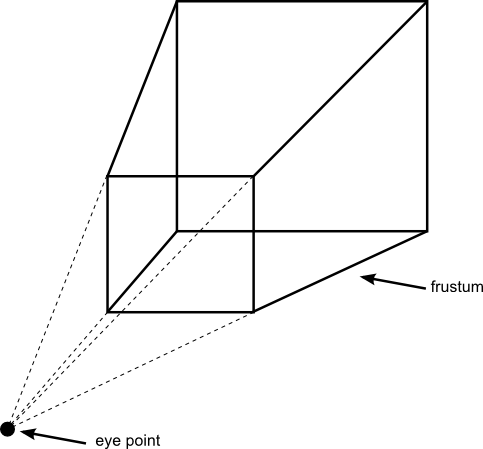
- Since:
- 2012.0
-
Method Summary
Modifier and TypeMethodDescriptionstatic TLspFrustum
createFromModelViewProjectionMatrix
(double[][] aMVPMatrix) Creates a frustum, based on the given modelview/projection matrix.static TLspFrustum
createFromViewRectangle
(Rectangle aViewRectangle, ALspViewXYZWorldTransformation aViewXYZWorldTransformation) Creates a frustum, based on the given rectangle in view coordinates and the given view to world transformation.Gets the bounds of the frustum.Gets the 8 corners of this frustum.double[][]
Gets the 6 planes that bounds this frustum.double[]
getPlaneSFCT
(int aIndex, double[] aCoefficientsSFCT) Returns the plane equation coefficients for a particular side of the frustum.boolean
isBoundsVisible
(ILcdBounds aBounds) Checks whether the given bounds (in world coordinates) are visible in this frustum.boolean
isPointVisible
(ILcdPoint aPoint) Checks whether the given point (in world coordinates) is visible in this frustum.toString()
Returns a textual representation of this frustum.
-
Method Details
-
createFromModelViewProjectionMatrix
Creates a frustum, based on the given modelview/projection matrix. The matrix is specified in row major order, i.e. it is to be indexed asaMVPMatrix[row][column]
.- Parameters:
aMVPMatrix
- the combined modelview and projection matrices for which to determine the frustum planes- Returns:
- a frustum, based on the given modelview/projection matrix.
- Throws:
IllegalArgumentException
- when the given matrix isnull
.
-
createFromViewRectangle
public static TLspFrustum createFromViewRectangle(Rectangle aViewRectangle, ALspViewXYZWorldTransformation aViewXYZWorldTransformation) Creates a frustum, based on the given rectangle in view coordinates and the given view to world transformation.- Parameters:
aViewRectangle
- the rectangle around which to fit a frustumaViewXYZWorldTransformation
- the view to world transform for which to determine the frustum- Returns:
- a frustum, based on the given rectangle in view coordinates and the given view to world transformation.
- Throws:
IllegalArgumentException
- when the given rectangle or view to world transformation arenull
-
toString
Returns a textual representation of this frustum. -
isBoundsVisible
Checks whether the given bounds (in world coordinates) are visible in this frustum. A bounds is visible if it intersects the volume defined by this frustum.- Parameters:
aBounds
- the bounds to be tested- Returns:
- true if the bounds are visible
- Throws:
IllegalArgumentException
- when the given bounds arenull
-
isPointVisible
Checks whether the given point (in world coordinates) is visible in this frustum.- Parameters:
aPoint
- the point to be tested- Returns:
- true if the point is visible
- Throws:
IllegalArgumentException
- when the given point isnull
-
getPlaneSFCT
public double[] getPlaneSFCT(int aIndex, double[] aCoefficientsSFCT) Returns the plane equation coefficients for a particular side of the frustum. Given the plane equationAx + By + Cz + D = 0
, this method returns the values ofA
,B
,C
andD
in an array. IfaCoefficientsSFCT
is not null and has at least four elements, it receives the coefficients. Otherwise, a new array is allocated. This method returns the array into which the coefficients were written.- Parameters:
aIndex
- the index of the plane to be retrieved (between 0 and 5, inclusive)aCoefficientsSFCT
- if not null, receives the plane equation coefficients in its first four elements- Returns:
- the array containing the plane equation coefficients
- Throws:
IllegalArgumentException
- when the given index is not in range[0, 5]
or whenaCoefficientsSFCT
is notnull
andaCoefficientsSFCT.length < 4
-
getPlanes
public double[][] getPlanes()Gets the 6 planes that bounds this frustum.
The result array should not be modified. To adapt the frustum, please create a new one using
createFromModelViewProjectionMatrix
orcreateFromViewRectangle
.- Returns:
- A double array containing the plane equations' coefficients
-
getCorners
Gets the 8 corners of this frustum. The result list and points should not be modified. To adapt the frustum, please usecomputeFromModelViewProjectionMatrix(double[][])
orcomputeFromViewRectangle(java.awt.Rectangle, com.luciad.view.lightspeed.camera.ALspViewXYZWorldTransformation)
.- Returns:
- the eight corner points that define this frustum
-
getBounds
Gets the bounds of the frustum.- Returns:
- the bounds of the frustum.
-