Class TLsp2DPointListEditor
java.lang.Object
com.luciad.view.lightspeed.editor.ALspEditor
com.luciad.view.lightspeed.editor.TLsp2DPointListEditor
- All Implemented Interfaces:
ILspEditor
Enables visual editing of
ILcd2DEditablePointList
objects in an ILspView
.
Handles
The 2D point list editor defines the following edit handles for a 2D point list object:- 2D point position handles: allows the user to edit the positions of points in the point list. The
user can change the position of the points by dragging the handle. This handle generates
MOVE
operations, with aPOINT_INDEX
property. - Object translation handle: allows the user to translate the 2D point list object.
This handle is activated by pressing and dragging the shape itself. This handle generates
MOVE
operations, without any additional properties. - Point remove handle: allows the user to remove a point from the point list. This handle
generates
REMOVE_POINT
operations. - Point insert handle: Allows the user to insert a point to the point list. This handle
generates
INSERT_POINT
operations.
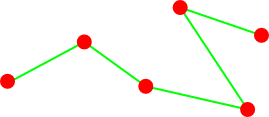
Editing
Based on theTLspEditOperation
, generated by an edit handle (see description
of handles above), this editor performs different edit operations on the associated 2D point
list object. The images below illustrate the effect of the different handles. In each image the
gray color represents the previous state of the object and the red color represents the edited object:
- Point handle: the editor adjusts the corresponding point of the point list
- Object translation handle: the editor translates the entire point list
Creation
The creation process of a 2D point list object consists of a series of steps where the XY location of the next point is initialized. This process continues until the user performs a finishing event (such as a double-click when using the mouse) which results in creation being finalized. Each of these steps can be undone by using the BACKSPACE key. The figures below illustrate the the creation process. Relevant changes that are new in each step are marked in red. |
||||||
![]() |
|
![]() |
|
![]() |
|
![]() |
Adding a first point | |
Adding a second point | |
Adding a third point | |
Adding a fourth point and finalizing the creation process |
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Defines keys used by the enclosing editor implementation to store properties in its edit handles. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionboolean
canEdit
(TLspEditContext aContext) protected ALspEditHandle
createInsertPointHandle
(ILcd2DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to insert points into the given point list.protected ALspEditHandle
createObjectTranslationHandle
(ILcd2DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given shape.protected ALspEditHandle
createPointHandle
(ILcd2DEditablePointList aPointList, int aIndex, TLspEditContext aContext) Creates an edit handle for the point with indexaIndex
of the given point list.protected ALspEditHandle
createRemovePointHandle
(ILcd2DEditablePointList aPointList, int aIndex, TLspEditContext aContext) Creates a point removal handle for the point with indexaIndex
of the given point list.protected TLspEditOperationResult
editImpl
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.Returns how the editor behaves during creation.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.void
setCreationMode
(ELspCreationMode aCreationMode) Sets how the editor behaves during creationMethods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
canCopyGeometry, canPerformOperation, copyGeometrySFCT, edit, fireUndoableHappened
-
Constructor Details
-
TLsp2DPointListEditor
public TLsp2DPointListEditor()Creates a newTLsp2DPointListEditor
. This constructor does not initialize any state.
-
-
Method Details
-
canEdit
- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this editor delegates to the following methods: It returns a list containing the handles returned by those methods. These methods are added for convenience, so they can easily be overridden. Note that for the point handles, it delegates multiple times to those methods with different indices. The only exception is the insert point handle, which is only a single instance. By default this method returns a list that is twice the size, plus two, of the size of the pointlist.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createObjectTranslationHandle
protected ALspEditHandle createObjectTranslationHandle(ILcd2DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given shape. By default this is aTLspObjectTranslationHandle
.- Parameters:
aPointList
- the point list for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createInsertPointHandle
protected ALspEditHandle createInsertPointHandle(ILcd2DEditablePointList aPointList, TLspEditContext aContext) Creates an edit handle that allows the user to insert points into the given point list.This handle generates a
INSERT_POINT
operation, with aTLspIndexedPointDescriptor
in the properties. This object describes where the new point should be inserted, and at what index in the point list.- Parameters:
aPointList
- the point list for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createPointHandle
protected ALspEditHandle createPointHandle(ILcd2DEditablePointList aPointList, int aIndex, TLspEditContext aContext) Creates an edit handle for the point with indexaIndex
of the given point list. By default this method returns a point drag handle that allows the user to move the associated point to another location. APOINT_INDEX
property with the given index as value is set on the handle.- Parameters:
aPointList
- the point list for which the handle is returnedaIndex
- the index of the point in the point list, for which the handle is createdaContext
- the current context- Returns:
- a handle that allows the user to edit the point with given index, or
null
if no handle is needed
-
createRemovePointHandle
protected ALspEditHandle createRemovePointHandle(ILcd2DEditablePointList aPointList, int aIndex, TLspEditContext aContext) Creates a point removal handle for the point with indexaIndex
of the given point list. This handle generates aREMOVE_POINT
operation, with aTLspIndexedPointDescriptor
in the properties to denote which point should be removed.- Parameters:
aPointList
- the point list for which the handle is returnedaIndex
- the index of the point in the point list, for which the handle is createdaContext
- the current context- Returns:
- a handle that allows the user to remove the point with given index, or
null
if no handle is needed
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcd2DEditablePointList
, as described in the class javadoc.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle, or
null
if creation should not be allowed. - See Also:
-
getCreationMode
Returns how the editor behaves during creation. ELspCreationMode determines the behavior of the handles obtained ingetCreateHandle(TLspEditContext)
to create point lists.- Returns:
- the ELspCreationMode of the point list editor
-
setCreationMode
Sets how the editor behaves during creation- Parameters:
aCreationMode
- the ELspCreationMode
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations: If any of the operations contains thePOINT_INDEX
property, with an integer value, then this editor will only perform the operation on that particular point, otherwise it will perform it on the entire point list.- Specified by:
editImpl
in classALspEditor
- Parameters:
aOperation
- the event that contains the information on how to edit the objectaInteractionStatus
-aContext
- the edit context- Returns:
SUCCESS
if the above conditions are met,FAILED
otherwise. The invalidation hint will be set if a point was added or removed.
-