Class TLcdGXYHatchedFillStyle
- All Implemented Interfaces:
ILcdCloneable
,ILcdGXYPainterStyle
,Serializable
,Cloneable
ILcdGXYPainterStyle
that fills areas by hatching them with a given line width and
color.
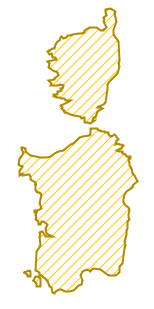
For performance reasons, a pattern is created by drawing one line in every necessary direction (horizontal, vertical, slash or back slash) on top of the background color (if background is enabled). This pattern is then tiled to fill the required area.
If you only intend to use this style for solid fills, it is better to use
TLcdGXYPainterColorStyle
for performance reasons.
Example code:
// Init painter modes
ALcdGXYAreaPainter painter = ...;
painter.setMode( ALcdGXYAreaPainter.OUTLINED_FILLED );
painter.setSelectionMode( ALcdGXYAreaPainter.OUTLINED_FILLED );
// Create fill style
painter.setFillStyle( new TLcdGXYHatchedFillStyle(
EnumSet.of( TLcdGXYHatchedFillStyle.Pattern.SLASH ), Color.ORANGE, Color.ORANGE.brighter() ) );
// Create matching line style
TLcdG2DLineStyle lineStyle = new TLcdG2DLineStyle();
lineStyle.setColor( Color.ORANGE.darker() );
lineStyle.setSelectionColor( Color.ORANGE );
lineStyle.setLineWidth( 2 );
lineStyle.setSelectionLineWidth( 2 );
lineStyle.setAntiAliasing( true );
painter.setLineStyle( lineStyle );
- Since:
- 10.0
- See Also:
-
Nested Class Summary
Nested Classes -
Constructor Summary
ConstructorsConstructorDescriptionCreates a newTLcdGXYHatchedFillStyle
with default values for all properties.TLcdGXYHatchedFillStyle
(EnumSet<TLcdGXYHatchedFillStyle.Pattern> aPattern, Color aLineColor) Creates a newTLcdGXYHatchedFillStyle
.TLcdGXYHatchedFillStyle
(EnumSet<TLcdGXYHatchedFillStyle.Pattern> aPattern, Color aLineColor, Color aSelectionLineColor) Creates a newTLcdGXYHatchedFillStyle
. -
Method Summary
Modifier and TypeMethodDescriptionvoid
addPropertyChangeListener
(PropertyChangeListener aListener) Adds the given listener to the list of listeners, so that it will receive property change events.void
addPropertyChangeListener
(String aProperty, PropertyChangeListener aListener) Adds the given listener to the list of listeners, so that it will receive notifications about changes inaProperty
.asIcon()
Returns this style as a tileable icon.clone()
MakesObject.clone()
public.protected void
firePropertyChange
(String aProperty, Object aOldValue, Object aNewValue) Fires the given event to the associated listeners.Returns the background paint.Returns the line color.int
Returns the line width.Returns the fill pattern.Returns the pattern size.Returns the selection background paint.Returns the selection line color.int
Returns the selection line width.Returns the fill pattern for selection mode.boolean
Returns true if anti aliasing is enabled, false otherwise.void
Removes the given listener so that it no longer receives property change events.void
removePropertyChangeListener
(String aProperty, PropertyChangeListener aListener) Removes the given listener for the given property, so that it no longer receives those change events.void
setAntiAliasing
(boolean aAntiAliasing) Sets the anti aliasing property.void
setBackgroundPaint
(Paint aBackgroundPaint) Sets the background paint (e.g. aColor
).void
setLineColor
(Color aLineColor) Sets the line color for filling shapes in their normal (unselected) mode.void
setLineWidth
(int aLineWidth) Sets the line width for filling shapes in their normal (unselected) mode.void
setPattern
(EnumSet<TLcdGXYHatchedFillStyle.Pattern> aPattern) Sets the filling pattern.void
setPatternSize
(Dimension aPatternSize) Sets the pixel size of the filling pattern.void
setSelectionBackgroundPaint
(Paint aSelectionBackgroundPaint) Sets the selection background paint (e.g. a Color).void
setSelectionLineColor
(Color aSelectionLineColor) Sets the line color for filling shapes in their selected mode.void
setSelectionLineWidth
(int aSelectionLineWidth) Sets the line width for filling shapes in their selected mode.void
setSelectionPattern
(EnumSet<TLcdGXYHatchedFillStyle.Pattern> aSelectionPattern) Sets the filling pattern for selection mode.void
setupGraphics
(Graphics aGraphics, Object aObject, int aMode, ILcdGXYContext aGXYContext) Set upaGraphics
before painting aObject.
-
Constructor Details
-
TLcdGXYHatchedFillStyle
public TLcdGXYHatchedFillStyle()Creates a newTLcdGXYHatchedFillStyle
with default values for all properties. -
TLcdGXYHatchedFillStyle
Creates a newTLcdGXYHatchedFillStyle
.- Parameters:
aPattern
- The pattern set, used for both regular and selection mode.aLineColor
- The color for painting shapes in their normal (unselected) mode.
-
TLcdGXYHatchedFillStyle
public TLcdGXYHatchedFillStyle(EnumSet<TLcdGXYHatchedFillStyle.Pattern> aPattern, Color aLineColor, Color aSelectionLineColor) Creates a newTLcdGXYHatchedFillStyle
.- Parameters:
aPattern
- The pattern set, used for both regular and selection mode.aLineColor
- The color for painting shapes in their normal (unselected) mode.aSelectionLineColor
- The color for painting shapes in their selected mode.
-
-
Method Details
-
addPropertyChangeListener
Adds the given listener to the list of listeners, so that it will receive property change events.- Parameters:
aListener
- The listener to add.
-
addPropertyChangeListener
Adds the given listener to the list of listeners, so that it will receive notifications about changes inaProperty
.- Parameters:
aProperty
- The property to watch for changes.aListener
- The listener to add.
-
removePropertyChangeListener
Removes the given listener so that it no longer receives property change events.- Parameters:
aListener
- The listener to remove.
-
removePropertyChangeListener
Removes the given listener for the given property, so that it no longer receives those change events.- Parameters:
aProperty
- The property to stop listening to.aListener
- The listener to remove.
-
firePropertyChange
Fires the given event to the associated listeners.- Parameters:
aProperty
- The property that was changed.aOldValue
- The old value.aNewValue
- The new value.
-
getPattern
Returns the fill pattern.- Returns:
- the fill pattern.
-
setPattern
Sets the filling pattern.- Parameters:
aPattern
- An enum set of the definedPattern
s, for exampleEnumSet.of( Pattern.HORIZONTAL, Pattern.VERTICAL )
-
getSelectionPattern
Returns the fill pattern for selection mode.- Returns:
- the fill pattern for selection mode.
-
setSelectionPattern
Sets the filling pattern for selection mode.- Parameters:
aSelectionPattern
- An enum set of the definedPattern
s, for exampleEnumSet.of( Pattern.HORIZONTAL, Pattern.VERTICAL )
-
getPatternSize
Returns the pattern size.- Returns:
- the pattern size.
-
setPatternSize
Sets the pixel size of the filling pattern. The pattern is created by drawing one line in every necessary direction (horizontal, vertical, slash or back slash). This pattern is then tiled to fill the required area. Changing the size of this pattern allows to define the spacing between adjacent lines. Defining a non-square pattern size for slanted patterns (slash and back slash) allows to change the angle of the slanted lines. For example a slash pattern with a pattern size of 20x10 results in 26.6 degree lines (Math.atan(10/20)).
Width and height must be strictly larger than twice (line width + 2), where line width is the largest of the selection or regular line width. The values should be small for performance reasons, for example 10x10 or 20x10 pixels.
After the dimension is set, the instance must not be changed anymore.
- Parameters:
aPatternSize
- The new pattern size.
-
getLineWidth
public int getLineWidth()Returns the line width.- Returns:
- the line width.
-
setLineWidth
public void setLineWidth(int aLineWidth) Sets the line width for filling shapes in their normal (unselected) mode.- Parameters:
aLineWidth
- The line width in pixels.
-
getSelectionLineWidth
public int getSelectionLineWidth()Returns the selection line width.- Returns:
- the selection line width.
-
setSelectionLineWidth
public void setSelectionLineWidth(int aSelectionLineWidth) Sets the line width for filling shapes in their selected mode.- Parameters:
aSelectionLineWidth
- The selection line width in pixels.
-
getLineColor
Returns the line color.- Returns:
- the line color.
-
setLineColor
Sets the line color for filling shapes in their normal (unselected) mode.- Parameters:
aLineColor
- The line color
-
getSelectionLineColor
Returns the selection line color.- Returns:
- the selection line color.
-
setSelectionLineColor
Sets the line color for filling shapes in their selected mode.
Note that the
ILcdGXYPainter
should paint its objects filled when selected for this property to have effect, e.g.ALcdGXYAreaPainter painter = ...; painter.setSelectionMode( ALcdGXYAreaPainter.OUTLINED_FILLED ); painter.setFillStyle( new TLcdGXYHatchedFillStyle() );
- Parameters:
aSelectionLineColor
- The color of the line when painting objects in their selected state.
-
getBackgroundPaint
Returns the background paint.- Returns:
- the background paint.
-
setBackgroundPaint
Sets the background paint (e.g. a
Color
). Only applicable if the fill pattern containsTLcdGXYHatchedFillStyle.Pattern.BACKGROUND
.In combination with
Paint
's other than a simpleColor
(e.g. aGradientPaint
), one should realize that a (small) pattern is tiled to fill the desired area. As a result it is for example not possible to have a gradient that extends from the top to the bottom of the view.- Parameters:
aBackgroundPaint
- The paint to fill the background with, in regular (unselected) mode.
-
getSelectionBackgroundPaint
Returns the selection background paint.- Returns:
- the selection background paint.
-
setSelectionBackgroundPaint
Sets the selection background paint (e.g. a Color). Only applicable if the fill pattern contains
TLcdGXYHatchedFillStyle.Pattern.BACKGROUND
. SeesetBackgroundPaint(java.awt.Paint)
for more details.- Parameters:
aSelectionBackgroundPaint
- The paint to fill the background with, in selected mode.
-
isAntiAliasing
public boolean isAntiAliasing()Returns true if anti aliasing is enabled, false otherwise.- Returns:
- true if anti aliasing is enabled, false otherwise.
-
setAntiAliasing
public void setAntiAliasing(boolean aAntiAliasing) Sets the anti aliasing property. Anti-aliasing yields smoother results.- Parameters:
aAntiAliasing
- True to enable anti-aliasing, false otherwise.
-
setupGraphics
public void setupGraphics(Graphics aGraphics, Object aObject, int aMode, ILcdGXYContext aGXYContext) Description copied from interface:ILcdGXYPainterStyle
Set upaGraphics
before painting aObject. For example, if(aMode & ILcdGXYPainter.SELECTED) != 0
, you may want to callaGraphics.setColor(Color.red)
for instance, to see the selected representation of a aObject in red.- Specified by:
setupGraphics
in interfaceILcdGXYPainterStyle
- Parameters:
aGraphics
- theGraphics
to set up.aObject
- theObject
to paint.aMode
- the painting mode to consider (defined inILcdGXYPainter
).aGXYContext
- theILcdGXYContext
to consider.- See Also:
-
asIcon
Returns this style as a tileable icon. The resulting icon will have the same size as the pattern size. When changing the style instance after having created an icon, the icon will not change. I.e. the changes to the style are not applied to the icon.
- Returns:
- this style as an icon.
-
clone
Description copied from interface:ILcdCloneable
Makes
When for example extending fromObject.clone()
public.java.lang.Object
, it can be implemented like this:public Object clone() { try { return super.clone(); } catch ( CloneNotSupportedException e ) { // Cannot happen: extends from Object and implements Cloneable (see also Object.clone) throw new RuntimeException( e ); } }
- Specified by:
clone
in interfaceILcdCloneable
- Overrides:
clone
in classObject
- See Also:
-