Class ALcdTerrainElevationProvider
- All Implemented Interfaces:
ILcdAltitudeProvider
- Direct Known Subclasses:
ALcdRasterTerrainElevationProvider
,TLcdHeightProviderAdapter
Provides terrain elevation data. All terrain elevation analysis classes that require terrain
elevation data should retrieve the data through this implementation. Different strategies for
retrieving terrain data can then seamlessly be plugged into the terrain elevation analysis
classes by replacing the ALcdTerrainElevationProvider
implementation.
The altitude or elevation values, provided by implementations of this class, are defined in
meters above geoid. Special values should be interpreted as defined by the altitude
descriptor (from getAltitudeDescriptor()
). Keep in mind that Double.NaN
,
Double.NEGATIVE_INFINITY
and Double.POSITIVE_INFINITY
can also be
used as special values.
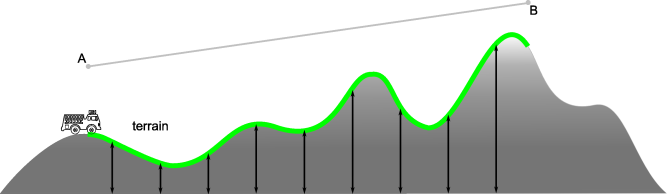
-
Constructor Summary
ConstructorsModifierConstructorDescriptionprotected
Defines anILcdAltitudeProvider
that returns terrain elevation values above the geoid from the specified location reference. -
Method Summary
Modifier and TypeMethodDescriptionReturns the descriptor which describes how to interpret the altitude values.ReturnsTLcdCoverageAltitudeMode.ABOVE_GEOID
.Returns the preferred reference for this terrain elevation provider.boolean
isValidElevation
(double aElevation) Determines whether the given elevation is valid.double
retrieveAltitudeAt
(ILcdPoint aPoint, ILcdGeoReference aPointReference) Returns the altitude in meters for the specified point.abstract double
retrieveElevationAt
(ILcdPoint aPoint, ILcdGeoReference aPointReference) Returns the elevation for a given location, defined in meters above the geoid from the given location reference.
-
Constructor Details
-
Method Details
-
retrieveElevationAt
Returns the elevation for a given location, defined in meters above the geoid from the given location reference.Special elevation values should be interpreted as defined by the altitude descriptor (from
getAltitudeDescriptor()
). Keep in mind thatDouble.NaN
,Double.NEGATIVE_INFINITY
andDouble.POSITIVE_INFINITY
can also be used as special values.- Parameters:
aPoint
- The point indicating the location for which to retrieve the elevation.aPointReference
- The reference in which the given location is defined. Note that the ellipsoid from this reference should be used to define the returning elevation value.- Returns:
- the elevation for a given location, defined in meters above the ellipsoid.
-
retrieveAltitudeAt
Returns the altitude in meters for the specified point. The representation of this value is defined by the altitude mode (from
ILcdAltitudeProvider.getAltitudeMode()
) and the altitude descriptor (fromILcdAltitudeProvider.getAltitudeDescriptor()
).If no altitude value can be found for the specified point, the provider should return a value with an appropriate interpretation. For example, when a point is chosen outside the bounds of the elevation raster data, the value corresponding to the interpretation
OUTSIDE_RASTER_BOUNDS
can be returned.This implementations calls the method
retrieveElevationAt(ILcdPoint, ILcdGeoReference)
to retrieve the altitude value for the given location.- Specified by:
retrieveAltitudeAt
in interfaceILcdAltitudeProvider
- Parameters:
aPoint
- The location for which to retrieve the altitude.aPointReference
- The reference in which the point is defined.- Returns:
- the altitude in meters for the specified point.
-
getPreferredReference
Returns the preferred reference for this terrain elevation provider. This reference is the reference with the best performance when used as a parameter in the methodretrieveElevationAt(ILcdPoint, ILcdGeoReference)
. It is perfectly correct to use points with other references. It will be faster to use the preferred.For performance reasons, the preferred reference should be the reference of the available elevation data to minimize the number of point transformations. By default, this method returns
null
.Keep in mind that this function can return
null
if no preferred reference is available.- Specified by:
getPreferredReference
in interfaceILcdAltitudeProvider
- Returns:
- the preferred reference of this terrain elevation provider. Note that
null
can be returned if no preferred reference is available. - See Also:
-
getAltitudeDescriptor
Description copied from interface:ILcdAltitudeProvider
Returns the descriptor which describes how to interpret the altitude values.- Specified by:
getAltitudeDescriptor
in interfaceILcdAltitudeProvider
- Returns:
- the descriptor which describes how to interpret the altitude values.
-
getAltitudeMode
ReturnsTLcdCoverageAltitudeMode.ABOVE_GEOID
. All altitudes returned by this provider should be interpreted as defined above the geoid of the specified location reference.- Specified by:
getAltitudeMode
in interfaceILcdAltitudeProvider
- Returns:
TLcdCoverageAltitudeMode.ABOVE_GEOID
.
-
isValidElevation
public boolean isValidElevation(double aElevation) Determines whether the given elevation is valid. This implementation returns
false
if the elevation is a special value according to the altitude descriptor.- Parameters:
aElevation
- the elevation to check.- Returns:
true
if the elevation is valid,false
otherwise.
-