WMS and WMTS servers may advertise map layers with one or more dimensions. Common examples are layers with time and altitude dimensions. You can then request WMS maps or WMTS tiles for specific times and altitudes from those servers. The server defines the available dimensions in the capabilities metadata for the advertised layers.
The LuciadLightspeed WMS and WMTS client APIs provide full support to access the available dimensions for a layer, supply dimension values in requests, and visualize the resulting data in a 2D and 3D view.
Discovering the available dimensions
Through the multi-dimensional model
If you have decoded a model for a WMS or WMTS layer using TLcdOGCWMSProxyModelDecoder
or TLcdWMTSModelDecoder
,
you can access the available dimensions through the ILcdMultiDimensionalModel
interface.
LuciadLightspeed models that represent multi-dimensional data, such as WMS and WMTS layers, implement this interface.
For more information about using this interface to access the available dimensions,
see the How to add multi-dimensional data to an application? article.
Through the capabilities
If you don’t have a decoded model yet, you can also discover the available dimensions for a WMS or WMTS layer through the capabilities of the server.
Discovering the dimensions for a WMS layer
When you have discovered the available WMS layers in the capabilities, you can access the dimensions for a WMS layer as follows:
ALcdWMSNamedLayer layer = ...;
System.out.println("Available WMS dimensions for layer " + layer.getNamedLayerName());
for(int i = 0; i < layer.getDimensionCount(); i++) {
ALcdOGCWMSDimension dimension = layer.getDimension(i);
System.out.println(dimension.getName());
}
Using ALcdOGCWMSDimension
, you can retrieve the dimension name, but you can also get other information about the dimension, such as the unit
of measure and the possible dimension values.
Discovering the dimensions for a WMTS layer
When you have discovered the available WMTS layers in the capabilities, you can access the dimensions for a WMTS layer as follows:
TLcdWMTSLayer layer = ...;
System.out.println("Available WTMS dimensions for layer " + layer.getId());
layer.getDimensions().forEach(System.out::println);
Using TLcdWMTSLayer
, you can get more information about a dimension .
Requesting WMS or WMTS data for a given dimension
Through the multi-dimensional model
Similar to the discovery of the available dimensions, you can rely on the ILcdMultiDimensionalModel
interface
to set a desired dimension value. This code illustrates setting a time value for a time dimension:
ILcdDimension timeDimension = ...; // retrieved through ILcdMultiDimensionalModel.getDimensions
Date timeDimensionValue = (Date) new TLcdISO8601DateFormat().parseObject("2024-01-01T12:00:00Z");
TLcdDimensionFilter timeDimensionFilter = TLcdDimensionFilter.newBuilder()
.filterDimension(timeDimension.getAxis(), TLcdDimensionInterval.createSingleValue(Date.class, timeDimensionValue))
.build();
try (TLcdLockUtil.Lock lock = TLcdLockUtil.writeLock(model);) {
model.applyDimensionFilter(timeDimensionFilter, ILcdModel.FIRE_LATER);
}
model.fireCollectedModelChanges();
When this code runs, the data on your map refreshes with new data requested from the server for the given time dimension value.
For more information about setting dimension values using ILcdMultiDimensionalModel
,
see the How to add multi-dimensional data to an application? article.
Through the UI
The LuciadLightspeed applications Lucy and Lucy Map Centric have a UI. They automatically pick up time and altitude dimensions and turn them into dimension sliders on the map. These sliders allow users to choose dimension values, as illustrated in this image:
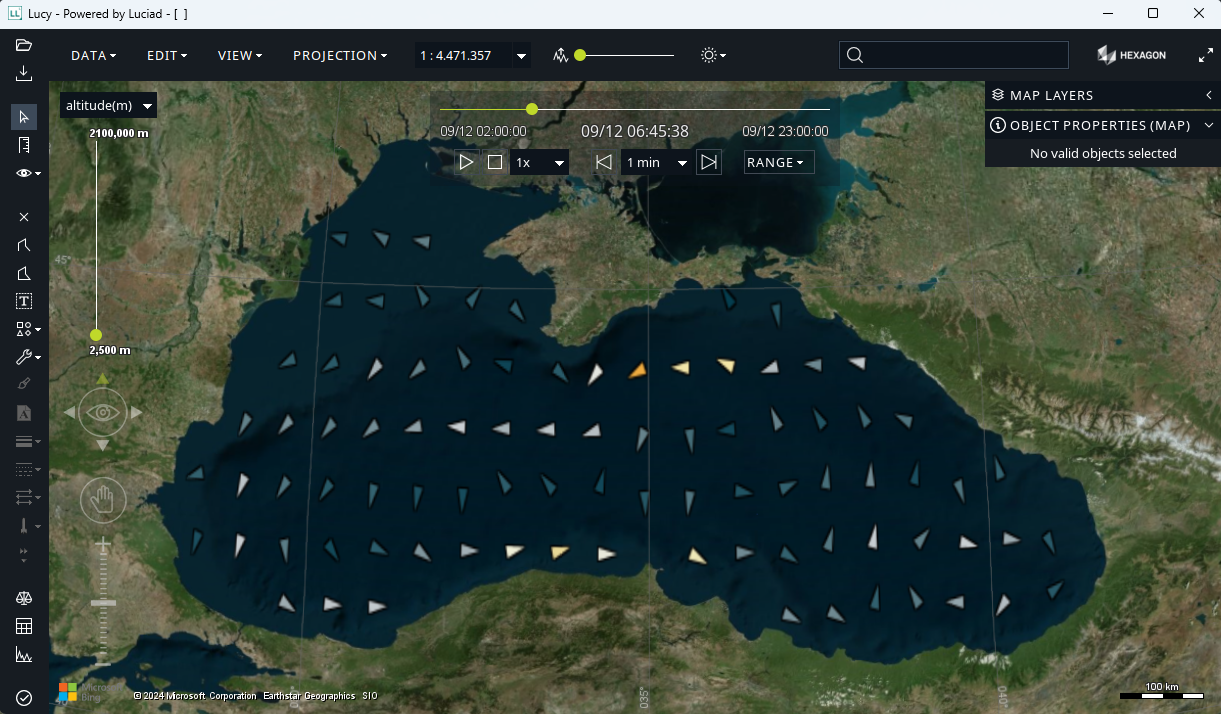
The GXY and Lightspeed decoder samples show the same behavior through a
samples.common.dimensionalfilter.LayerDimensionalFilterCustomizer
.