Class TLsp3DIconStyle
- All Implemented Interfaces:
ILspEffectsHintStyle
,ILspWorldElevationStyle
,ILspStyler
3D Icon style for styling points with 3D icons in 2D and 3D views.
Construction of a 3D icon style is done through the
Builder
design pattern mechanism.
A typical use-case of a TLsp3DIconStyle
is to visualize moving tracks,
where the icon could be a car, an airplane, ... .
Such an icon has a specific orientation.
For example, the car should be visualized in such a way that it is driving forwards and not sideways or backwards.
LuciadLightspeed assumes a "Z-axis up convention", meaning that the icons should be oriented as illustrated below with an airplane:
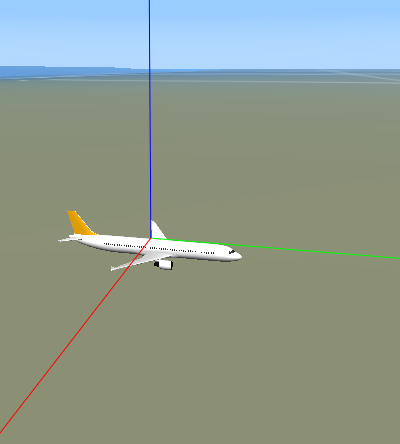
- The axis colors on the screen shot are RGB, pointing respectively to the positive XYZ axis.
- The front of the airplane should be pointing in the positive Y-direction (green) in order for the airplane to fly forward.
- The wings of the airplane should be aligned with the X-axis (red) to ensure that the airplane does not fly sideways.
Note that when you want to visualize a moving airplane,
it is recommended to let your domain object (or geometry) implement ILcd3DOriented
.
LuciadLightspeed will then automatically apply the pitch, yaw and roll angles on your airplane icon, making it fly in a very realistic manner.
This is illustrated in the samples.lightspeed.icons3d
sample.
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
TLsp3DIconStyle.Builder<B extends TLsp3DIconStyle.Builder<B>>
Builder for 3D icon styles.static enum
Mode that indicates how the icon size is determined.Nested classes/interfaces inherited from interface com.luciad.view.lightspeed.style.ILspEffectsHintStyle
ILspEffectsHintStyle.EffectsHint
Nested classes/interfaces inherited from interface com.luciad.view.lightspeed.style.ILspWorldElevationStyle
ILspWorldElevationStyle.ElevationMode
-
Constructor Summary
ConstructorsModifierConstructorDescriptionprotected
TLsp3DIconStyle
(TLsp3DIconStyle.Builder<?> aBuilder) Creates a new style with the properties that are set on the builder -
Method Summary
Modifier and TypeMethodDescriptionCreates a new builder initialized with all the properties of this style.boolean
Returns the effects hints specified for this style.Returns the elevation mode of this style.getIcon()
Gets the icon.int
Returns the minimum icon size in pixels.Gets the color that is modulated with the icon.float
Gets the opacity of the icon.int
Gets the icon size in pixels.double
Gets the counterclockwise rotation angle in degrees around the X axis.double
Gets the counterclockwise rotation angle in degrees around the Y axis.double
Gets the counterclockwise rotation angle in degrees around the Z axis.double
getScale()
Deprecated.double
Gets the icon scale factor on the X-axis.double
Gets the icon scale factor on the Y-axis.double
Gets the icon scale factor on the Z-axis.Gets the scaling mode of the icon.double
Gets the translation via the X axisdouble
Gets the translation via the Y axisdouble
Gets the translation via the Z axisdouble
Gets the vertical offset factor of the icon.double
Gets the icon world size in meters.int
hashCode()
boolean
Checks whether or not the painter should recenter icons on the point at which the icons are drawn.boolean
Determines whether the icon has a transparent surface or not.static TLsp3DIconStyle.Builder
<?> Creates a new builder with the default values.toString()
Methods inherited from class com.luciad.view.lightspeed.style.ALspStyle
addStyleChangeListener, getZOrder, isCompatible, removeStyleChangeListener, style
-
Constructor Details
-
TLsp3DIconStyle
Creates a new style with the properties that are set on the builder- Parameters:
aBuilder
- a builder which will be used to initialize this style.
-
-
Method Details
-
newBuilder
Creates a new builder with the default values.- Returns:
- the new builder.
-
asBuilder
Creates a new builder initialized with all the properties of this style. -
getIcon
Gets the icon.- Returns:
- the resulting icon
-
getScale
Deprecated.Gets the icon scale factor.- Returns:
- the scale factor
- Throws:
IllegalStateException
- if the scale factor isn't the same for each axis.- See Also:
-
getScaleX
public double getScaleX()Gets the icon scale factor on the X-axis.- Returns:
- the scale factor on the X-axis
- See Also:
-
getScaleY
public double getScaleY()Gets the icon scale factor on the Y-axis.- Returns:
- the scale factor on the Y-axis
- See Also:
-
getScaleZ
public double getScaleZ()Gets the icon scale factor on the Z-axis.- Returns:
- the scale factor on the Z-axis
- See Also:
-
getRotationX
public double getRotationX()Gets the counterclockwise rotation angle in degrees around the X axis.- Returns:
- the rotation angle around the X axis
-
getRotationY
public double getRotationY()Gets the counterclockwise rotation angle in degrees around the Y axis.- Returns:
- the rotation angle around the X axis
-
getRotationZ
public double getRotationZ()Gets the counterclockwise rotation angle in degrees around the Z axis.- Returns:
- the rotation angle around the Z axis
-
getTranslationX
public double getTranslationX()Gets the translation via the X axis- Returns:
- the translation via the X axis.
-
getTranslationY
public double getTranslationY()Gets the translation via the Y axis- Returns:
- the translation via the Y axis.
-
getTranslationZ
public double getTranslationZ()Gets the translation via the Z axis- Returns:
- the translation via the Z axis.
-
getWorldSize
public double getWorldSize()Gets the icon world size in meters.- Returns:
- the world size
- See Also:
-
getPixelSize
public int getPixelSize()Gets the icon size in pixels.- Returns:
- the pixel size
- See Also:
-
getMinimumPixelSize
public int getMinimumPixelSize()Returns the minimum icon size in pixels. This size only affects the style if the scaling mode is set toTLsp3DIconStyle.ScalingMode.WORLD_SCALING
. As soon as the largest dimension (i.e. width, height or depth) of the 3D icon is smaller than this minimum pixel size, the icon will be scaled to satisfy this minimum size. By default this value is set to 0, which indicates that no minimum size will be enforced.- Returns:
- A positive number expressing the minimum icon size in pixels.
-
getVerticalOffsetFactor
public double getVerticalOffsetFactor()Gets the vertical offset factor of the icon. This offset factor is used to reposition an icon relative to the point at which it is drawn.The factor is relative to the size of the icon: a value of 1.0 will shift the icon up by half its height, a value of -1.0 will shift it down by the same distance.
- Returns:
- the vertical offset factor of the icon
-
getScalingMode
Gets the scaling mode of the icon.- Returns:
- the icon size mode
-
isTransparent
public boolean isTransparent()Determines whether the icon has a transparent surface or not.- Specified by:
isTransparent
in classALspStyle
- Returns:
- whether or not the icon is transparent
-
isRecenterIcon
public boolean isRecenterIcon()Checks whether or not the painter should recenter icons on the point at which the icons are drawn.If
true
, 3D icons will be translated such that the center of their bounds coincides with the location at which they are being painted.If
false
, the origin of the icon's coordinate system will be mapped to the point being painted.- Returns:
true
if recentering is enabled,false
otherwise
-
getOpacity
public float getOpacity()Gets the opacity of the icon.- Returns:
- the opacity of the icon
-
getModulationColor
Gets the color that is modulated with the icon. For example using an icon with gray-scale colors and a red modulation color will result in a resulting painted icon with red hues.- Returns:
- the modulation color
-
getEffectsHints
Description copied from interface:ILspEffectsHintStyle
Returns the effects hints specified for this style.- Specified by:
getEffectsHints
in interfaceILspEffectsHintStyle
- Returns:
- the effects hints specified for this style
-
getElevationMode
Description copied from interface:ILspWorldElevationStyle
Returns the elevation mode of this style.- Specified by:
getElevationMode
in interfaceILspWorldElevationStyle
- Returns:
- The elevation mode.
-
equals
-
hashCode
public int hashCode() -
toString
-