Class TLspGeoBufferEditor
java.lang.Object
com.luciad.view.lightspeed.editor.ALspEditor
com.luciad.view.lightspeed.editor.TLspGeoBufferEditor
- All Implemented Interfaces:
ILspEditor
Enables visual editing of
ILcd2DEditableGeoBuffer
and
TLcdLonLatBuffer
objects in an ILspView
.
Handles
The buffer editor defines the following edit handles for a buffer object:- Point handles: allows the user to edit the base shape of the buffer object. The
user can change the points of the base shape by dragging the handles. This handle generates
MOVE
operations, with aPOINT_INDEX
property. - Outline handle: allows the user to change the width of the buffer by dragging the
outline. this handle generates
PROPERTY_CHANGE
operations, with ""width"" as property name, and aDouble
as value. - Object translation handle: allows the user to translate the buffer object. This handle
generates
MOVE
operations, without any additional properties.
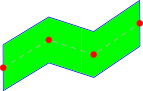
Editing
Based on theTLspEditOperation
, generated by an edit handle (see description
of handles above), this editor performs different edit operations on the associated buffer object.
The images below illustrate the effect of the different handles. In each image the
gray color represents the previous state of the object and the red color represents the edited object:
-
Point handles: Allows to edit the base shape of the buffer object.
-
Outline handle: Allows to edit the width of the buffer by dragging its outline.
-
Object translation handle: the editor translates the entire buffer.
Creation
The creation process of a buffer object consists of 2 steps:- Add new points to the buffer
- After adding all points to the buffer, initialize the width of the buffer
![]() |
![]() |
|
Adding points to the buffer | Initializing the width |
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Defines keys used by the enclosing editor implementation to store properties in its edit handles. -
Constructor Summary
ConstructorsConstructorDescriptionCreates a newTLspGeoBufferEditor
.TLspGeoBufferEditor
(TLsp2DPointListEditor aCustomPointListEditorDelegate) Creates a newTLspGeoBufferEditor
. -
Method Summary
Modifier and TypeMethodDescriptionboolean
canEdit
(TLspEditContext aContext) Returnstrue
ifsuper
returnstrue
and the given object is an instance ofTLcdLonLatBuffer
or an instance ofILcd2DEditableGeoBuffer
.protected ALspEditHandle
createBufferOutlineHandle
(Object aObject, TLspEditContext aContext) Creates an edit handle that allows the user to adjust the width of the given buffer by modifying its outline.protected TLspEditOperationResult
editImpl
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.Returns how the delegate point list editor behaves during creation.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.void
setCreationMode
(ELspCreationMode aCreationMode) Returns how the delegate point list editor behaves during creation.Methods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
canCopyGeometry, canPerformOperation, copyGeometrySFCT, edit, fireUndoableHappened
-
Constructor Details
-
TLspGeoBufferEditor
public TLspGeoBufferEditor()Creates a newTLspGeoBufferEditor
. This constructor initializes a defaultTLsp2DPointListEditor
to be used as a delegate when editing and creating points in a geobuffer. -
TLspGeoBufferEditor
Creates a newTLspGeoBufferEditor
. This constructor uses the givenTLsp2DPointListEditor
as a delegate when editing and creating points inside a geobuffer.- Parameters:
aCustomPointListEditorDelegate
- the delegate point list editor to be used for creating and editing points in this pointlist.
-
-
Method Details
-
canEdit
Returnstrue
ifsuper
returnstrue
and the given object is an instance ofTLcdLonLatBuffer
or an instance ofILcd2DEditableGeoBuffer
.- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this method delegates to thecreateBufferOutlineHandle
method. It is added for convenience, so it can easily be overridden. Further, it returns the same list of handles asTLsp2DPointListEditor
.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createBufferOutlineHandle
Creates an edit handle that allows the user to adjust the width of the given buffer by modifying its outline.- Parameters:
aObject
- the buffer object for which the handle is created, this can be of typeTLcdLonLatBuffer
orTLcdLonLatGeoBuffer
aContext
- the current context- Returns:
- an edit handle or
null
when no handle is needed
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcd2DEditableGeoBuffer
or aTLcdLonLatBuffer
, as described in the class javadoc.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle, or
null
if creation should not be allowed. - See Also:
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations:TLspEditOperationType.MOVE
TLspEditOperationType.INSERT_POINT
TLspEditOperationType.REMOVE_POINT
TLspEditOperationType.PROPERTY_CHANGE
POINT_INDEX
property, with an integer value, then this editor will only perform the operation on that particular point, otherwise it will perform it on the entire point list. If the operation type isTLspEditOperationType.PROPERTY_CHANGE
, and the name of the changed property is"width"
, the width of the buffer is modified.- Specified by:
editImpl
in classALspEditor
- Parameters:
aOperation
- the event that contains the information on how to edit the objectaInteractionStatus
- the interaction statusaContext
- the edit context- Returns:
SUCCESS
if the above conditions are met,FAILED
otherwise. The invalidation hint will be set if a point was added or removed.
-
getCreationMode
Returns how the delegate point list editor behaves during creation.- Returns:
- the creation mode of the delegate point list editor
-
setCreationMode
Returns how the delegate point list editor behaves during creation.- Parameters:
aCreationMode
- the creation mode to be set on the delegate point list editor
-