Class TLspEllipseEditor
java.lang.Object
com.luciad.view.lightspeed.editor.ALspEditor
com.luciad.view.lightspeed.editor.TLspEllipseEditor
- All Implemented Interfaces:
ILspEditor
Enables visual editing of
ILcd2DEditableEllipse
objects in an
ILspView
.
Handles
The ellipse editor defines the following edit handles for an ellipse object:- Corner point handles: allows the user to edit the corner points of the ellipse. The
user can change the location of these corner points by dragging the corresponding handle.
These handles generate
MOVE
operations, with aHANDLE_IDENTIFIER
property andMAJOR_AXIS
,MAJOR_AXIS_OPPOSITE
,MINOR_AXIS
orMINOR_AXIS_OPPOSITE
as value. - Center point handle: allows the user to translate the center of the ellipse. The
user can change the position of the center point by dragging the handle. This handle
generates
MOVE
operations, with aHANDLE_IDENTIFIER
property withCENTER
as value. - Object translation handle: allows the user to translate the ellipse object. This handle
generates
MOVE
operations, without any additional properties.
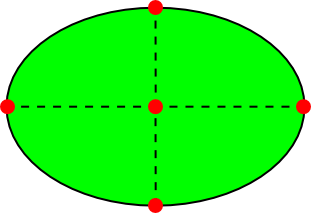
Editing
Based on theTLspEditOperation
, generated by an edit handle (see description
of handles above), this editor performs different edit operations on the associated ellipse object.
The images below illustrate the effect of the different handles. In each image the
gray color represents the previous state of the object and the red color represents the edited object:
-
Corner point handles: the editor adjusts the corresponding corner point of the ellipse,
based on the new location of the point handle. The figure below illustrates this behaviour.
-
Center point handle: the editor translates the entire ellipse. The figure below illustrates
this behaviour when moving the center point.
-
Object translation handle: the editor translates the entire ellipse. Note that the behaviour
for this handle is identical to the behaviour of the center point handle, with the exception of
how the handle is activated
Creation
The creation process of an ellipse object consists of the following steps:- the first step sets the center of the ellipse
- the second step initializes the major axis of the ellipse
- the third step initializes the minor axis of the ellipse
![]() |
![]() |
![]() |
||
Setting the center point | Initializing the major axis | Initializing the minor axis |
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Describes a control point of an ellipse.static enum
Defines keys used by the enclosing editor implementation to store properties in its edit handles. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionboolean
canEdit
(TLspEditContext aContext) protected ALspEditHandle
createCenterHandle
(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows to translate the ellipse by dragging the center point.protected ALspEditHandle
createMajorAxisHandle
(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows to change the major axis of the ellipse.protected ALspEditHandle
createMinorAxisHandle
(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows to change the minor axis of the ellipse.protected ALspEditHandle
createObjectTranslationHandle
(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given shape.protected TLspEditOperationResult
editImpl
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.Methods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
canCopyGeometry, canPerformOperation, copyGeometrySFCT, edit, fireUndoableHappened
-
Constructor Details
-
TLspEllipseEditor
public TLspEllipseEditor()Creates a newTLspEllipseEditor
. This constructor does not initialize any state.
-
-
Method Details
-
canEdit
- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this method delegates to the following methods: It returns a list containing the handles returned by those methods. These methods are added for convenience, so they can easily be overridden.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createObjectTranslationHandle
protected ALspEditHandle createObjectTranslationHandle(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given shape. By default this is aTLspObjectTranslationHandle
.- Parameters:
aEllipse
- the shape for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createMajorAxisHandle
protected ALspEditHandle createMajorAxisHandle(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows to change the major axis of the ellipse.- Parameters:
aEllipse
- the ellipseaContext
- provides context information such as the model reference in which the ellipse is defined- Returns:
- an edit handle or
null
if no handle is needed
-
createMinorAxisHandle
protected ALspEditHandle createMinorAxisHandle(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows to change the minor axis of the ellipse.- Parameters:
aEllipse
- the ellipseaContext
- provides context information such as the model reference in which the ellipse is defined- Returns:
- an edit handle or
null
if no handle is needed
-
createCenterHandle
protected ALspEditHandle createCenterHandle(ILcd2DEditableEllipse aEllipse, TLspEditContext aContext) Creates an edit handle that allows to translate the ellipse by dragging the center point.- Parameters:
aEllipse
- the ellipseaContext
- provides context information such as the model reference in which the ellipse is defined- Returns:
- an edit handle or
null
if no handle is needed
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcd2DEditableEllipse
, as described in the class javadoc.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle, or
null
if creation should not be allowed. - See Also:
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations: If any of the operations contains theHANDLE_IDENTIFIER
property, with aHandleIdentifier
as value, then this editor will only perform the operation on that particular point, otherwise it will perform it on the entire object.- Specified by:
editImpl
in classALspEditor
- Parameters:
aOperation
- the event that contains the information on how to edit the objectaInteractionStatus
- the interaction statusaContext
- the edit context- Returns:
- The result of the operation: Whether or not there was success, and whether or not the current handles of the object should be invalidated.
-