Class TLspBoundsEditor
java.lang.Object
com.luciad.view.lightspeed.editor.ALspEditor
com.luciad.view.lightspeed.editor.TLspBoundsEditor
- All Implemented Interfaces:
ILspEditor
Enables visual editing of
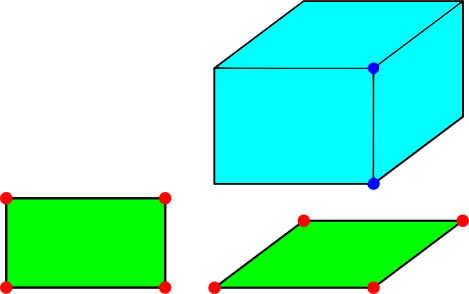
(left) handles for a 2D bounds object (right) handles for a 3D bounds object
ILcd2DEditableBounds
and ILcd3DEditableBounds
objects in an ILspView
.
Handles
The bounds editor defines the following edit handles for a bounds object:- Corner handles: allows the user to edit the bounds by moving its corners. The user can
change the position of the corners by dragging its handle. This handle generates
MOVE
operations, with aPOINT_IDNETIFIER
property, withNORTH_EAST
,NORTH_WEST
,SOUTH_EAST
orSOUTH_WEST
as value. - Object translation handle: allows the user to translate the bounds object.
This handle generates
MOVE
operations, without any additional properties. - Height translation handle: (only for 3D bounds in a 3D view) allows the user to edit
the height (above the terrain) of the bounds object. This handle generates
MOVE
operations, with aOPERATION_IDENTIFIER
property, withHEIGHT
as value. - Minimum height handle: (only for 3D bounds in a 3D view) allows the user to edit
the height above the terrain of the location point of the bounds. This handle generates
MOVE
operations, with aOPERATION_IDENTIFIER
property, withMINIMUM_HEIGHT
as value. - Maximum height handle: (only for 3D bounds in a 3D view) allows the user to edit
the depth of the bounds. This handle generates
MOVE
operations, with aOPERATION_IDENTIFIER
property, withMAXIMUM_HEIGHT
as value.
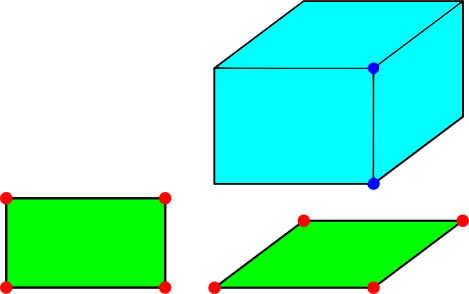
(left) handles for a 2D bounds object (right) handles for a 3D bounds object
Editing
Based on theTLspEditOperation
, generated by an edit handle (see
description of handles above), this editor performs different edit operations on the associated
bounds object. The images below illustrate the effect of the different handles. In each image the
gray color represents the previous state of the object and the red color represents the edited
object:
-
Corner handles: Translates one of the corners of the bounds.
(left) 2D bounds case (right) 3D bounds case
-
Object translation handle: the editor translates the entire circle.
(left) 2D bounds case (right) 3D bounds case
-
Height translation handle: 3D only, modifies the height of the 3D bounds.
-
Minimum height handle: 3D only, modifies the minimum height of the 3D bounds.
-
Maximum height handle: 3D only, modifies the maximum height of the 3D bounds.
Creation
The creation process of a 2D bounds object consists of two steps:- the first step initializes the location of the bounds
- the second step initializes the width and height of the bounds
- the third step initializes the depth of the bounds
- the fourth step initializes the height of the bounds above the terrain
![]() |
![]() |
![]() |
![]() |
|||
Adding points to the buffer | Initializing the width | (3D only) Initializing the depth | (3D only) Initializing the height |
- Since:
- 2012.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Describes a control point of a bounds.static enum
Defines keys used by the enclosing editor implementation to properties in its edit handles. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionboolean
canEdit
(TLspEditContext aContext) protected ALspEditHandle
createBoundsResizeHandle
(ILcd2DEditableBounds aBounds, TLspEditContext aContext, TLspBoundsEditor.HandleIdentifier aPointID) Creates a handle that allows the user to resize the given bounds object by translating one of its corner points.protected ALspEditHandle
createHeightTranslationHandle
(ILcd3DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to edit the height above the terrain of the given 3D bounds by dragging the bounds up/down.protected ALspEditHandle
createMaximumHeightHandle
(ILcd3DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to edit the depth of the given 3D bounds by dragging it up/down.protected ALspEditHandle
createMinimumHeightHandle
(ILcd3DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to edit the Z-value of the given 3D bounds' location by dragging it up/down.protected ALspEditHandle
createObjectTranslationHandle
(ILcd2DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given shape.protected TLspEditOperationResult
editImpl
(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
.getCreateHandle
(TLspEditContext aContext) Returns a handle that is used to create the given object.getEditHandles
(TLspEditContext aContext) Returns a set of handles for editing the given object.Methods inherited from class com.luciad.view.lightspeed.editor.ALspEditor
canCopyGeometry, canPerformOperation, copyGeometrySFCT, edit, fireUndoableHappened
-
Constructor Details
-
TLspBoundsEditor
public TLspBoundsEditor()Creates a newTLspBoundsEditor
. This constructor does not initialize any state.
-
-
Method Details
-
canEdit
- Specified by:
canEdit
in interfaceILspEditor
- Overrides:
canEdit
in classALspEditor
- Parameters:
aContext
- provides context information to the editor- Returns:
true
if the above conditions are met,false
otherwise.
-
getEditHandles
Returns a set of handles for editing the given object. These handles will be able to generate edit operations, that are passed to theedit
method. As a way to communicate with this method, handles will copy their properties to the edit operation properties. By default this method delegates to the following methods:createMaximumHeightHandle
createMinimumHeightHandle
createHeightTranslationHandle
createBoundsResizeHandle
createObjectTranslationHandle
createBoundsResizeHandle
is called 4 times, one time for each corner of the bounds. This method only delegate to the first three methods is the object is anILcd3DEditableBounds
and if the current view world transformation is aTLspViewXYZWorldTransformation3D
.- Parameters:
aContext
- provides context information such as the layer for which the object is being edited- Returns:
- the edit handles to edit the given object, or an empty list if it should not be possible to edit the given object.
- See Also:
-
createMinimumHeightHandle
protected ALspEditHandle createMinimumHeightHandle(ILcd3DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to edit the Z-value of the given 3D bounds' location by dragging it up/down.The handle is painted at the location of the focus point of the given bounds. The handle's height above the terrain is always equal to
Note that by default this method only returns a height translation handle when the current view is a 3D view. If this is not the case, this method returnsaBounds.getLocation().getZ()
.null
since in a 2D view, the height translation handle is not useful. This method is only called when the bounds is aILcd3DEditableBounds
. By default this method returns a handle with aOPERATION_IDENTIFIER
user property, withMINIMUM_HEIGHT
as value.- Parameters:
aBounds
- the bounds for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
when no handle is needed
-
createHeightTranslationHandle
protected ALspEditHandle createHeightTranslationHandle(ILcd3DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to edit the height above the terrain of the given 3D bounds by dragging the bounds up/down. Note that by default this method only returns a height translation handle when the current view is a 3D view. If this is not the case, this method returnsnull
since in a 2D view, the height translation handle is not useful. This method is only called when the bounds is aILcd3DEditableBounds
. By default this method returns a handle with aOPERATION_IDENTIFIER
user property, withHEIGHT
as value.- Parameters:
aBounds
- the bounds for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
when no handle is needed
-
createMaximumHeightHandle
protected ALspEditHandle createMaximumHeightHandle(ILcd3DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to edit the depth of the given 3D bounds by dragging it up/down.The handle is painted at the location of the focus point of the given bounds. The handle's height above the terrain is always equal to
Note that by default this method only returns a height translation handle when the current view is a 3D view. If this is not the case, this method returnsaBounds.getLocation().getZ() + aBounds.getDepth()
.null
since in a 2D view, the height translation handle is not useful. This method is only called when the bounds is aILcd3DEditableBounds
. By default this method returns a handle with aOPERATION_IDENTIFIER
user property, withMAXIMUM_HEIGHT
as value.- Parameters:
aBounds
- the bounds for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
when no handle is needed
-
createObjectTranslationHandle
protected ALspEditHandle createObjectTranslationHandle(ILcd2DEditableBounds aBounds, TLspEditContext aContext) Creates an edit handle that allows the user to translate the given shape. By default this is aTLspObjectTranslationHandle
.- Parameters:
aBounds
- the shape for which the handle is createdaContext
- the current context- Returns:
- an edit handle or
null
if no handle is needed
-
createBoundsResizeHandle
protected ALspEditHandle createBoundsResizeHandle(ILcd2DEditableBounds aBounds, TLspEditContext aContext, TLspBoundsEditor.HandleIdentifier aPointID) Creates a handle that allows the user to resize the given bounds object by translating one of its corner points.- Parameters:
aBounds
- the bounds object for which the handle is createdaContext
- the current contextaPointID
- corner of the bounds for which to create a handle- Returns:
- a resize handle or
null
when no resize handle is needed
-
getCreateHandle
Returns a handle that is used to create the given object. The returned handle is used by the controller to initialize the other of the object. Typically the returned handle is an instance ofALspCreateHandle
which is a specialized handle implementation used for creating an object. By default, this method returns anALspCreateHandle
capable of creating anILcd2DEditableBounds
, as described in the class javadoc.- Parameters:
aContext
- provides context information such as the layer for which the object is being created- Returns:
- an edit handle, or
null
if creation should not be allowed. - See Also:
-
editImpl
protected TLspEditOperationResult editImpl(TLspEditOperation aOperation, ELspInteractionStatus aInteractionStatus, TLspEditContext aContext) Called by theedit
method to edit the given object based on the givenedit operation
. Note that this method does not need to lock the model of the object, this already happens in theedit
method. By default, this editor can handle the following operations: If any of the operations contains theHANDLE_IDENTIFIER
property, with aHandleIdentifier
as value, then this editor will only perform the operation on that particular point. If any of the operations contains theOPERATION_IDENTIFIER
property, with aHandleIdentifier
as value, then this editor will only perform that particular operation. Otherwise it will move the entire object.- Specified by:
editImpl
in classALspEditor
- Parameters:
aOperation
- the event that contains the information on how to edit the objectaInteractionStatus
- the interaction statusaContext
- the edit context- Returns:
- The result of the operation: Whether or not there was success, and whether or not the current handles of the object should be invalidated.
-