Class TLcdGXYArcPainter
- All Implemented Interfaces:
ILcdCloneable
,ILcdPropertyChangeSource
,ILcdGXYEditor
,ILcdGXYEditorProvider
,ILcdGXYPainter
,ILcdGXYPainterProvider
,ILcdGXYPathPainter
,Serializable
,Cloneable
ILcdArc
objects and enables visual editing of
ILcd2DEditableArc
objects in an ILcdGXYView
.
Painting an ILcdArc
Body
The body of an ILcdArc
object is painted as:
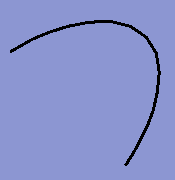
Handles
This painter defines the two arc corner points of the ILcdArc
object and the four axis
corner points as a handle of the arc. The following image clarifies the handle location. The arc
corner points are represented by blue points, the axis corner points by red points.
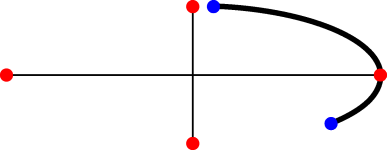
Note that the center point of the arc is painted in
BODY
mode depending on the drawCenter
property. When painted, it can be used as a handle to edit the object.
Snap targets
One of the arc corner points of the ILcdArc
object can be returned on the condition
that it is touched. If the center point is painted (see isDrawCenter()
) or when snapping
to invisible points is enabled (see isSnapToInvisiblePoints()
), the center point can also
be returned. The point returned as a snap target is highlighted with the snapIcon
.
Styling options
The visualization of the ILcdArc
object is governed by the line style set to this painter.
Locating an ILcdArc in a view
Anchor point of an ILcdArc
The location of the anchor point can be set using setAnchorPointLocation(int)
to the
middle of the arc bounds, the middle of the chord or the middle of the arc itself. By default,
the anchor point is located at the center point of the bounds, calculated by
boundsSFCT
. Note that the center point of the ILcdArc
object is not taken into account.
When is an ILcdArc touched
Depending on the paint mode, an arc is touched when either the arc, the arc handles, the axis handles or the center point of the arc is touched. Note that the center point can only be touched if it is painted.
Visual bounds of an ILcdArc
The bounds of the ILcdArc
object contains the contour of the arc and the center point
if it is painted.
Visually editing and creating an ILcd2DEditableArc
Modifying an ILcd2DEditableArc
This editor provides the following edit functionality for the different render modes:
RESHAPED
: an axis handle is reshaped, depending on whether it is touched. The image below illustrate the editing behavior. The small black arrow indicates the path of the mouse cursor while reshaping the arc. The rotation angle and the length of the axis are updated. The gray dotted lines indicate that the start angle and arc angle of the arc remain unchanged.
Moving an axis point. TRANSLATED
: an arc handles or the whole arc is translated, depending on whether an arc handle or the arc segment is touched. The images below illustrate the editing behavior. The small black arrow indicates the path of the mouse cursor while translating the arc.
Moving an arc point.
Moving the arc segment.
Note that, if the center point or the axis handles of the arc are touched, the whole arc can be translated as if the arc segment was touched.
Creating an ILcd2DEditableArc
When initializing an arc via interaction through the view, a number of user interactions are required to
complete the initialization. This number depends on the creation mode
and
it is either 2, 3 or 5 for the modes TWO_CLICK
, THREE_CLICK
or FIVE_CLICK
.
The creationOrientation
property defines the orientation of the arc
during creation.
Accepted snap targets
All points are accepted as snap target as long as:
- the point can be transformed to the reference of the
ILcdArc
object with themodel to model transformation
, - the point is not the center point of the
ILcdArc
object set to this painter.
Additional properties
Caching
This painter implementation supports caching for objects implementing ILcdCache
.
Caching can be turned on/off with the setPaintCache
method.
Drawing the center point
The drawCenter
and drawCenterWhenSelected
properties define when the center point needs to be painted. If the center point is painted, it can
be touched, moved,...
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final int
Constant value indicating that the end point of the arc segment of the arc is touched.static final int
Constant value indicating that the start point of the arc segment of the arc is touched.static final int
Constant value indicating that the body of the arc is touched.static final int
Constant value indicating that the center point of the arc is touched.static final int
Constant for a clockwise orientation when creating an arc.static final int
Constant for a counter clockwise orientation when creating an arc.static final int
Constant for the default orientation when creating an arc.static final int
Constant value indicating that the anchor point should be chosen at the end of the curve if ILcdCurve is implemented.static final int
Constant for the five click creation mode.static final int
Constant value indicating that the anchor point should be chosen as the arc's focus point.static final int
Constant value indicating that the end point of the major axis of the arc is touched.static final int
Constant value indicating that the opposite end point of the major axis of the arc is touched.static final int
Constant value indicating that the anchor point should be chosen on the arc, at half of its length.static final int
Constant value indicating that the anchor point should be chosen in the middle of the arc's bounds, calculated byboundsSFCT
.static final int
Constant value indicating that the anchor point should be chosen in the middle of the chord determined by the start and end points.static final int
Constant value indicating that the anchor point should be chosen at the middle of the curve if ILcdCurve is implemented.static final int
Constant value indicating that the end point of the minor axis of the arc is touched.static final int
Constant value indicating that the opposite end point of the minor axis of the arc is touched.static final int
Constant value indicating that the anchor point should be chosen at the start of the curve if ILcdCurve is implemented.static final int
Constant for the three click creation mode.static final int
Constant for the two click creation mode.Fields inherited from class com.luciad.view.gxy.ALcdGXYPainter
defaultCreationFillStyle, defaultCreationLineStyle, defaultFillStyle, defaultLineStyle, fWorkBounds
Fields inherited from interface com.luciad.view.gxy.ILcdGXYEditor
CREATING, END_CREATION, RESHAPED, START_CREATION, TRANSLATED
Fields inherited from interface com.luciad.view.gxy.ILcdGXYPainter
CREATING, DEFAULT, HANDLES, RESHAPING, SELECTED, SNAPS, TRANSLATING
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs a defaultTLcdGXYArcPainter
and sets the display name to "Arc". -
Method Summary
Modifier and TypeMethodDescriptionboolean
acceptSnapTarget
(Graphics aGraphics, ILcdGXYContext aGXYContext) Determines whether a snap target should be accepted or not.void
anchorPointSFCT
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, Point aPointSFCT) Moves the specifiedaPointSFCT
to the anchor point of theILcdArc
object.boolean
appendAWTPath
(ILcdGXYContext aGXYContext, int aRenderMode, ILcdAWTPath aAWTPathSFCT) Appends a discretized representation in view coordinates to the given path.boolean
appendGeneralPath
(ILcdGXYContext aGXYContext, int aRenderMode, ILcdGeneralPath aGeneralPathSFCT) Appends a discretized representation in world coordinates to the given path.protected void
boundsOfEditingLabelsSFCT
(Graphics aGraphics, ILcdArc aArc, int aTouchedLocation, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) Called by theboundsSFCT
method when editing anILcd2DEditableArc
.void
boundsSFCT
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) Computes the bounds of the representation of anILcdArc
in AWT coordinates.clone()
MakesObject.clone()
public.boolean
edit
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) This implementation edits theILcdArc
object set to the painter.int
Returns the location of the anchor point (by default: MIDDLE_OF_BOUNDS).Returns the icon for the center point's default representation, used ifisDrawCenter()
returns true.Returns the icon for the center point's selected representation, used ifisDrawCenterWhenSelected()
returns true.int
Returns either 2, 3 or 5 depending on thecreation mode
(TWO_CLICK
,THREE_CLICK
orFIVE_CLICK
) as the number of required user interactions.int
Returns the orientation used while creatingILcdArc
objects.getCursor
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Returns aCursor
that clarifies the render mode and context this painter is operating in.getGXYEditor
(Object aObject) Returns this instance as editor for editing the specifiedObject
.Returns the line style used for painting the contour of theILcdArc
object.int
Returns the minimum pixel distance the input device (a mouse, for example) must move before editing the shape.Returns the object that can currently be painted or edited by this painter/editor.boolean
Returns whether caching is used when painting this painter's object.Returns the icon that is used to paint snap target points of the object set to this painter.boolean
Returns whether the center point of the arc should be displayed.boolean
Returns whether the center point of the arc should be displayed when it is painted in selected mode.boolean
Returns whether the painter allows snapping to the invisible points of the shape.boolean
isTouched
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Depending on the rendering mode, returns whether the arc, the arc handles, the axis handles or the center point of the arc is touched.boolean
Deprecated.This method has been deprecated.void
paint
(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext) Displays the representation of the object in the given mode on the Graphics passed, taking into account the context passed.protected void
paintEditingLabels
(Graphics aGraphics, ILcdArc aArc, int aTouchedLocation, ILcdGXYContext aGXYContext) Called by thepaint
method when editing anILcd2DEditableArc
.void
setAnchorPointLocation
(int aAnchorPointLocation) Sets the preferred anchor point location.void
setCenterIcon
(ILcdIcon aIcon) Sets the icon for the center point's default representation, ifisDrawCenter()
returns true.void
Sets the icon for the center point's selected representation, ifisDrawCenterWhenSelected()
returns true.void
setCreationMode
(int aCreationMode) Sets the mode to decide how to create anILcdArc
object.void
setCreationOrientation
(int aCreationOrientation) Sets the orientation used while creatingILcdArc
objects.void
setDrawCenter
(boolean aDrawCenter) Sets whether the center point of the arc should be displayed or not.void
setDrawCenterWhenSelected
(boolean aDrawCenterWhenSelected) Sets whether the center point of the arc should be displayed when it is painted in selected mode or not.void
setLineStyle
(ILcdGXYPainterStyle aLineStyle) Sets the line style to use when painting the contour of theILcdArc
object.void
setMinimumEditDelta
(int aDelta) Sets the minimum pixel distance the input device (a mouse, for example) must move before editing the shape.void
setModelModelTransformationClass
(Class aModel2ModelTransformationClass) Sets the transformation class that should be used when snapping to points that are defined in a different reference than the reference of this painter's object.void
Sets the object to paint and edit.void
setPaintCache
(boolean aPaintCache) Turns caching of the representation of the object on or off.void
setSnapIcon
(ILcdIcon aSnapIcon) Sets the icon that marks snap targets of the object currently set to this painter.void
setSnapToInvisiblePoints
(boolean aSnapToInvisiblePoints) Sets whether the other shapes can snap to invisible points of this shape.void
setTraceOn
(boolean aTraceOn) Deprecated.This method has been deprecated.protected void
setupGraphicsForLine
(Graphics aGraphics, Object aObject, int aRenderMode, ILcdGXYContext aGXYContext) Called just before painting a line on the specifiedGraphics
object.snapTarget
(Graphics aGraphics, ILcdGXYContext aGXYContext) Returns the center point or one of the end points of theILcdArc
if it is touched.boolean
supportSnap
(Graphics aGraphics, ILcdGXYContext aGXYContext) This implementation supports snapping, always returnstrue
.Methods inherited from class com.luciad.view.gxy.ALcdGXYPainter
addPropertyChangeListener, firePropertyChangeEvent, firePropertyChangeEvent, getDisplayName, getGXYPainter, removePropertyChangeListener, setClassTraceOn, setDisplayName
Methods inherited from class java.lang.Object
equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface com.luciad.view.gxy.ILcdGXYEditor
getDisplayName
Methods inherited from interface com.luciad.view.gxy.ILcdGXYPainter
getDisplayName
Methods inherited from interface com.luciad.util.ILcdPropertyChangeSource
addPropertyChangeListener, removePropertyChangeListener
-
Field Details
-
CENTER
public static final int CENTERConstant value indicating that the center point of the arc is touched.- See Also:
-
BODY
public static final int BODYConstant value indicating that the body of the arc is touched.- See Also:
-
MAJOR_RADIUS_CORNER
public static final int MAJOR_RADIUS_CORNERConstant value indicating that the end point of the major axis of the arc is touched.- See Also:
-
MINOR_RADIUS_CORNER
public static final int MINOR_RADIUS_CORNERConstant value indicating that the end point of the minor axis of the arc is touched.- See Also:
-
MAJOR_RADIUS_OPPOSITE_CORNER
public static final int MAJOR_RADIUS_OPPOSITE_CORNERConstant value indicating that the opposite end point of the major axis of the arc is touched.- See Also:
-
MINOR_RADIUS_OPPOSITE_CORNER
public static final int MINOR_RADIUS_OPPOSITE_CORNERConstant value indicating that the opposite end point of the minor axis of the arc is touched.- See Also:
-
ARC_START_CORNER
public static final int ARC_START_CORNERConstant value indicating that the start point of the arc segment of the arc is touched.- See Also:
-
ARC_END_CORNER
public static final int ARC_END_CORNERConstant value indicating that the end point of the arc segment of the arc is touched.- See Also:
-
MIDDLE_OF_BOUNDS
public static final int MIDDLE_OF_BOUNDSConstant value indicating that the anchor point should be chosen in the middle of the arc's bounds, calculated byboundsSFCT
. Note that the center point of theILcdArc
object is not taken into account.- See Also:
-
FOCUS_POINT
public static final int FOCUS_POINTConstant value indicating that the anchor point should be chosen as the arc's focus point. If this point cannot be calculated, theMIDDLE_OF_BOUNDS
method is used to calculate the anchor point.- See Also:
-
MIDDLE_OF_CHORD
public static final int MIDDLE_OF_CHORDConstant value indicating that the anchor point should be chosen in the middle of the chord determined by the start and end points.- See Also:
-
MIDDLE_OF_ARC
public static final int MIDDLE_OF_ARCConstant value indicating that the anchor point should be chosen on the arc, at half of its length.- See Also:
-
MIDDLE_OF_CURVE
public static final int MIDDLE_OF_CURVEConstant value indicating that the anchor point should be chosen at the middle of the curve if ILcdCurve is implemented. Depending on the implementation of the arc, this method might give a different result then theMIDDLE_OF_ARC
method. If ILcdCurve is not implemented, or if no valid anchor point can be found, theMIDDLE_OF_BOUNDS
method is used.- See Also:
-
START_OF_CURVE
public static final int START_OF_CURVEConstant value indicating that the anchor point should be chosen at the start of the curve if ILcdCurve is implemented. If ILcdCurve is not implemented, or if no valid anchor point can be found, theMIDDLE_OF_BOUNDS
method is used.- See Also:
-
END_OF_CURVE
public static final int END_OF_CURVEConstant value indicating that the anchor point should be chosen at the end of the curve if ILcdCurve is implemented. If ILcdCurve is not implemented, or if no valid anchor point can be found, theMIDDLE_OF_BOUNDS
method is used.- See Also:
-
DEFAULT_ORIENTATION
public static final int DEFAULT_ORIENTATIONConstant for the default orientation when creating an arc. This constant is provided for backward compatibility as its behavior is not very consistent: it switches orientation at the 270 degrees azimuth mark, independent of the start angle of the arc.- See Also:
-
CLOCKWISE
public static final int CLOCKWISEConstant for a clockwise orientation when creating an arc.- See Also:
-
COUNTERCLOCKWISE
public static final int COUNTERCLOCKWISEConstant for a counter clockwise orientation when creating an arc.- See Also:
-
TWO_CLICK
public static final int TWO_CLICKConstant for the two click creation mode. The clicks define:- the position of the center point, and
- the semi-major axis and semi-minor axis length.
0
,45
and0
after creation. The semi-major and semi-minor axes are equal.- See Also:
-
THREE_CLICK
public static final int THREE_CLICKConstant for the three click creation mode. The clicks define:- the position of the center point,
- the semi-major axis and semi-minor axis length and the rotation angle of the ellipse on which the arc resides, and
- the end angle (and thus the arc angle) of the arc.
0
and semi-major and semi-minor axes are equal. For backwards compatibility reasons, this is the default creation mode.- See Also:
-
FIVE_CLICK
public static final int FIVE_CLICKConstant for the five click creation mode. The clicks define:- the position of the center point,
- the length of the semi-major axis and the rotation angle of the ellipse on which the arc resides,
- the length of the semi-minor axis,
- the start angle of the arc, and
- the end angle (and thus the arc angle) of the arc.
- See Also:
-
-
Constructor Details
-
TLcdGXYArcPainter
public TLcdGXYArcPainter()Constructs a defaultTLcdGXYArcPainter
and sets the display name to "Arc".
-
-
Method Details
-
setTraceOn
public void setTraceOn(boolean aTraceOn) Deprecated.This method has been deprecated. It is recommended to use the standard Java logging framework directly.Enables tracing for this class instance. Calling this method with eithertrue
orfalse
as argument automatically turns off tracing for all other class instances for whichsetTraceOn
has not been called. If the argument isfalse
then only the informative, warning and error log messages are recorded.- Overrides:
setTraceOn
in classALcdGXYPainter
- Parameters:
aTraceOn
- if true then all log messages are recorded for this instance. If false, then only the informative, warning and error log messages are recorded.
-
isTraceOn
public boolean isTraceOn()Deprecated.This method has been deprecated. It is recommended to use the standard Java logging framework directly.Returnstrue
if tracing is enabled for this class.- Overrides:
isTraceOn
in classALcdGXYPainter
- Returns:
- true if tracing is enabled for this class, false otherwise.
-
setObject
Sets the object to paint and edit. The object should be an instance of
ILcdArc
for painting and an instance ofILcd2DEditableArc
for editing.This painter/editor can be used to paint the object without using the edit functionality. This method shall therefore only check if the object implements
ILcdArc
and shall throw aClassCastException
if it does not.When this painter is used as editor with an object that does not implement the interface
ILcd2DEditableArc
, theedit
method shall throw the necessary exception.- Specified by:
setObject
in interfaceILcdGXYEditor
- Specified by:
setObject
in interfaceILcdGXYPainter
- Parameters:
aObject
- The object to paint and edit.- Throws:
ClassCastException
- when the object does not implementILcdArc
.- See Also:
-
getObject
Returns the object that can currently be painted or edited by this painter/editor.- Specified by:
getObject
in interfaceILcdGXYEditor
- Specified by:
getObject
in interfaceILcdGXYPainter
- Returns:
- the object that can currently be painted or edited by this painter/editor.
- See Also:
-
setCreationMode
public void setCreationMode(int aCreationMode) Sets the mode to decide how to create anILcdArc
object. An arc can be created in the following modes. The default value isTWO_CLICK
.TWO_CLICK
, which enables setting the center point and the radius,THREE_CLICK
, which enables setting the center point, the radius and the rotation angle. and the arc angle, orFIVE_CLICK
, which enables setting the center point, the major axis extent and the rotation angle, the minor axis extent, the start angle of the arc, and the arc angle of the arc.
- Parameters:
aCreationMode
- The mode deciding how to create anILcdArc
object.
-
setCreationOrientation
public void setCreationOrientation(int aCreationOrientation) Sets the orientation used while creating
ILcdArc
objects. The value passed should beDEFAULT_ORIENTATION
,CLOCKWISE
orCOUNTERCLOCKWISE
. The default value isDEFAULT_ORIENTATION
for backward compatibility reasons.This value will only be taken into account in creation modes where the choice of the start and arc angle are free (currently the three and five click creation modes).
- Parameters:
aCreationOrientation
- A flag indicating the orientation of the arc during creation.- See Also:
-
getCreationOrientation
public int getCreationOrientation()Returns the orientation used while creatingILcdArc
objects.- Returns:
- the orientation used while creating
ILcdArc
objects. - See Also:
-
setPaintCache
public void setPaintCache(boolean aPaintCache) Turns caching of the representation of the object on or off. Caching greatly reduces the time to paint an object but requires more memory. The representation of an object can only be cached for objects which implementILcdCache
. By default, caching is turned on.- Parameters:
aPaintCache
- A flag indicating whether to use caching when painting an object.- See Also:
-
getPaintCache
public boolean getPaintCache()Returns whether caching is used when painting this painter's object.- Returns:
true
if caching is used to paint this painter's object,false
otherwise.- See Also:
-
setDrawCenter
public void setDrawCenter(boolean aDrawCenter) Sets whether the center point of the arc should be displayed or not. The default value of this property isfalse
. This setting has an influence on the methodsboundsSFCT
,isTouched
and snapping. Regardless of this setting, the center point is always displayed as a handle in HANDLES mode to allow editing.- Parameters:
aDrawCenter
- A flag indicating whether the center point should be displayed or not.- See Also:
-
isDrawCenter
public boolean isDrawCenter()Returns whether the center point of the arc should be displayed.- Returns:
- whether the center point of the arc should be displayed.
- See Also:
-
setDrawCenterWhenSelected
public void setDrawCenterWhenSelected(boolean aDrawCenterWhenSelected) Sets whether the center point of the arc should be displayed when it is painted in selected mode or not. The default value of this property istrue
. This setting has an influence on the methodsboundsSFCT
,isTouched
and snapping. Regardless of this setting, the center point is always displayed as a handle in HANDLES mode to allow editing.- Parameters:
aDrawCenterWhenSelected
- A flag indicating whether the center point should be displayed when it is painted in selected mode or not.- See Also:
-
isDrawCenterWhenSelected
public boolean isDrawCenterWhenSelected()Returns whether the center point of the arc should be displayed when it is painted in selected mode.- Returns:
- whether the center point of the arc should be displayed when it is painted in selected mode.
- See Also:
-
setCenterIcon
Sets the icon for the center point's default representation, ifisDrawCenter()
returns true.- Parameters:
aIcon
- the icon for the center point's default representation- See Also:
-
getCenterIcon
Returns the icon for the center point's default representation, used ifisDrawCenter()
returns true.- Returns:
- the icon for the center point's default representation
-
setCenterIconWhenSelected
Sets the icon for the center point's selected representation, ifisDrawCenterWhenSelected()
returns true.- Parameters:
aIcon
- the icon for the center point's selected representation- See Also:
-
getCenterIconWhenSelected
Returns the icon for the center point's selected representation, used ifisDrawCenterWhenSelected()
returns true.- Returns:
- the icon for the center point's selected representation
-
setSnapToInvisiblePoints
public void setSnapToInvisiblePoints(boolean aSnapToInvisiblePoints) Sets whether the other shapes can snap to invisible points of this shape. For anILcdArc
object, there's only one invisible point, being the center point of the arc when it is not drawn. The default value isfalse
.- Parameters:
aSnapToInvisiblePoints
- A flag indicating whether snapping to the invisible points is allowed.- See Also:
-
isSnapToInvisiblePoints
public boolean isSnapToInvisiblePoints()Returns whether the painter allows snapping to the invisible points of the shape.- Returns:
- whether the painter allows snapping to the invisible points of the shape.
- See Also:
-
setLineStyle
Sets the line style to use when painting the contour of theILcdArc
object. Note that this property can be set tonull
.- Parameters:
aLineStyle
- the line style to use when painting the contour of theILcdArc
object.- See Also:
-
getLineStyle
Returns the line style used for painting the contour of theILcdArc
object. Note that this method can returnnull
.- Returns:
- the line style used for painting the contour of the
ILcdArc
object. - See Also:
-
setSnapIcon
Sets the icon that marks snap targets of the object currently set to this painter. This icon is painted when thepaint
method is called with the render modeILcdGXYPainter.SNAPS
.- Parameters:
aSnapIcon
- The icon that should be used to paint snap target points.- See Also:
-
getSnapIcon
Returns the icon that is used to paint snap target points of the object set to this painter.- Returns:
- the icon that is used to paint snap target points of the object set to this painter.
- See Also:
-
setMinimumEditDelta
public void setMinimumEditDelta(int aDelta) Sets the minimum pixel distance the input device (a mouse, for example) must move before editing the shape. A larger value ensures that the object easily "snaps" back in place, a small value allows fine editing.- Parameters:
aDelta
- the minimum pixel distance the input device should move in either the X or Y direction
-
getMinimumEditDelta
public int getMinimumEditDelta()Returns the minimum pixel distance the input device (a mouse, for example) must move before editing the shape. A larger value ensures that the object easily "snaps" back in place, a small value allows fine editing. The default value is 3 pixels.- Returns:
- the minimum pixel distance the input device should move in either the X or Y direction
-
getAnchorPointLocation
public int getAnchorPointLocation()Returns the location of the anchor point (by default: MIDDLE_OF_BOUNDS).- Returns:
- one of
MIDDLE_OF_BOUNDS
,FOCUS_POINT
,MIDDLE_OF_CHORD
,MIDDLE_OF_ARC
,MIDDLE_OF_CURVE
,START_OF_CURVE
,END_OF_CURVE
.
-
setAnchorPointLocation
public void setAnchorPointLocation(int aAnchorPointLocation) Sets the preferred anchor point location.- Parameters:
aAnchorPointLocation
- one ofMIDDLE_OF_BOUNDS
,FOCUS_POINT
,MIDDLE_OF_CHORD
,MIDDLE_OF_ARC
,MIDDLE_OF_CURVE
,START_OF_CURVE
,END_OF_CURVE
.
-
setupGraphicsForLine
protected void setupGraphicsForLine(Graphics aGraphics, Object aObject, int aRenderMode, ILcdGXYContext aGXYContext) Called just before painting a line on the specified
Graphics
object. It can be redefined in order to set specificGraphics
properties likeColor
, etc...This implementation calls
ILcdGXYPainterStyle.setupGraphics(java.awt.Graphics, java.lang.Object, int, com.luciad.view.gxy.ILcdGXYContext)
on the lineStyle property if a line style has been set to this instance.- Parameters:
aGraphics
- The graphics to paint the object on.aObject
- The object to paint.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.
-
paint
Displays the representation of the object in the given mode on the Graphics passed, taking into account the context passed.This implementation will use the specified render mode to determine how the object needs to be rendered. The object is rendered as shown in the images of the class documentation.
- Specified by:
paint
in interfaceILcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.
-
appendAWTPath
Description copied from interface:ILcdGXYPathPainter
Appends a discretized representation in view coordinates to the given path.- Specified by:
appendAWTPath
in interfaceILcdGXYPathPainter
- Parameters:
aGXYContext
- the graphics contextaRenderMode
- the rendering modeaAWTPathSFCT
- the path to append to- Returns:
- true if a discretization of the shape could be successfully appended to the path
-
appendGeneralPath
public boolean appendGeneralPath(ILcdGXYContext aGXYContext, int aRenderMode, ILcdGeneralPath aGeneralPathSFCT) Description copied from interface:ILcdGXYPathPainter
Appends a discretized representation in world coordinates to the given path.- Specified by:
appendGeneralPath
in interfaceILcdGXYPathPainter
- Parameters:
aGXYContext
- the graphics contextaRenderMode
- the rendering modeaGeneralPathSFCT
- the path to append to- Returns:
- true if a discretization of the shape could be successfully appended to the path
-
paintEditingLabels
protected void paintEditingLabels(Graphics aGraphics, ILcdArc aArc, int aTouchedLocation, ILcdGXYContext aGXYContext) Called by the
paint
method when editing anILcd2DEditableArc
. It draws labels indicating the values of properties being changed. For example, the coordinates of the center point of the arc, the major or minor axis extent, the start angle, etc ... This method is empty and therefore does not draw the labels but it can be redefined for specific needs.The
aTouchedLocation
argument indicates which part of the arc is being edited. For example,MAJOR_RADIUS_CORNER
corresponds to the end point of the major axis of the arc. It should be one of the following constants:CENTER
,BODY
,MAJOR_RADIUS_CORNER
,MINOR_RADIUS_CORNER
,MAJOR_RADIUS_OPPOSITE_CORNER
,MINOR_RADIUS_OPPOSITE_CORNER
,ARC_START_CORNER
,ARC_END_CORNER
- Parameters:
aGraphics
- The graphics to paint the object on.aArc
- The arc that is being edited.aTouchedLocation
- The part of the arc that is being edited.aGXYContext
- The context to render the object in.
-
boundsSFCT
public void boundsSFCT(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) throws TLcdNoBoundsException Computes the bounds of the representation of anILcdArc
in AWT coordinates. The bounds will include the center point of the arc depending on whether it is rendered or not. This in turn depends on the painter mode passed and the values of the propertiesdrawCenter
anddrawCenterWhenSelected
.- Specified by:
boundsSFCT
in interfaceILcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.aBoundsSFCT
- The bounds to update.- Throws:
TLcdNoBoundsException
- if no bounds can be determined for the representation of the object. This can be when the object does not have a representation in the given context, for example when it is located in a part of the world which is not visible in the projection as set in the views world reference.- See Also:
-
boundsOfEditingLabelsSFCT
protected void boundsOfEditingLabelsSFCT(Graphics aGraphics, ILcdArc aArc, int aTouchedLocation, ILcdGXYContext aGXYContext, ILcd2DEditableBounds aBoundsSFCT) Called by the
boundsSFCT
method when editing anILcd2DEditableArc
. It calculates the bounds of the labels indicating the values of properties being changed. For example, the coordinates of the center point of the arc, the major or minor axis extent, the start angle, etc ... Since by default, no labels are painted, this implementation is empty and theaBoundsSFCT
argument remains unchanged.Note that the
ILcd2DEditableBounds
object already contains the bounds of the edited object. Upon exit of this method, this object should be set to the union of the bounds of the edited object and the labels painted while editing.The
aTouchedLocation
argument indicates which part of the arc is being edited. For example,MAJOR_RADIUS_CORNER
corresponds to the end point of the major axis of the arc. It should be one of the following constants:CENTER
,MAJOR_RADIUS_CORNER
,MINOR_RADIUS_CORNER
,MAJOR_RADIUS_OPPOSITE_CORNER
,MINOR_RADIUS_OPPOSITE_CORNER
,ARC_START_CORNER
,ARC_END_CORNER
- Parameters:
aGraphics
- The graphics to paint the object on.aArc
- The arc that is being edited.aTouchedLocation
- The part of the arc that is being edited.aGXYContext
- The context to render the object in.aBoundsSFCT
- The bounds in view coordinates to be updated.
-
isTouched
Depending on the rendering mode, returns whether the arc, the arc handles, the axis handles or the center point of the arc is touched. Note that the center point can only be touched if it is painted. The coordinate for which the check is done is derived from the context passed as argument.- Specified by:
isTouched
in interfaceILcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context in which the object is rendered.- Returns:
- whether the arc, the arc handles, the axis handles or the center point of the arc is touched.
- See Also:
-
getCursor
Returns aCursor
that clarifies the render mode and context this painter is operating in. When no specificCursor
is required,null
is returned.- Specified by:
getCursor
in interfaceILcdGXYPainter
- Overrides:
getCursor
in classALcdGXYPainter
- Parameters:
aGraphics
- The graphics on which the object is painted.aRenderMode
- The mode to render the object in.aGXYContext
- The context in which the object is rendered.- Returns:
- a cursor to indicate the type of operating mode and context. Returns
null
if no particular cursor is required.
-
getGXYEditor
Returns this instance as editor for editing the specifiedObject
. If thisObject
is not the same as theObject
set to this painter, thesetObject(Object)
method is called to update the object set to the painter.- Specified by:
getGXYEditor
in interfaceILcdGXYEditorProvider
- Parameters:
aObject
- the object to be edited.- Returns:
- this instance as editor for editing the specified
Object
.
-
edit
This implementation edits the
ILcdArc
object set to the painter. The object needs to implement the interfaceILcd2DEditableArc
otherwise aClassCastException
will be thrown.In
ILcdGXYEditor.TRANSLATED
render mode, theILcdArc
object is edited as follows:- While touching an arc handle, the arc handle point gets translated.
- While touching an axis handle, the object gets translated as a whole.
- While touching the center point, the object gets translated as a whole.
- While touching the arc, the object gets translated as a whole.
In
ILcdGXYEditor.RESHAPED
render mode, theILcdArc
object is edited as follows:- While touching an axis handle, the length of the corresponding axis will change. Also the rotation angle of the axis will be affected. The start angle and the arc angle are unaffected.
The creation render mode behavior is defined by the
creation mode
. Further changes have to be done within edition mode.- In the
TWO_CLICK
mode, the first point determines the center point of the arc and the second the radius of the arc circle. The start angle of the arc will be0
degrees and the arc angle extent45
degrees counter-clockwise. - In the
THREE_CLICK
mode, the first point determines the center point of the arc, the second the radius and the rotation angle of the arc circle, and the third the end angle (and thus the arc angle) of the arc. The start angle of the arc angle will be0
degrees. - In the
FIVE_CLICK
mode, the first point determines the center point of the arc, the second the length of the semi-major axis and the rotation angle of the arc, the third the length of the semi-minor axis, the fourth the start angle of the arc, and the fifth the end angle (and thus the arc angle) of the arc.
If, in all render modes, a snap target is found for a moved or created point, the snap target location is used for the moved/created point.
- Specified by:
edit
in interfaceILcdGXYEditor
- Parameters:
aGraphics
- The graphics to edit the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.- Returns:
- true if the object has changed when this method returns, false otherwise.
- Throws:
ClassCastException
- if the painter's object does not implementILcd2DEditableArc
.- See Also:
-
getCreationClickCount
public int getCreationClickCount()Returns either 2, 3 or 5 depending on thecreation mode
(TWO_CLICK
,THREE_CLICK
orFIVE_CLICK
) as the number of required user interactions.- Specified by:
getCreationClickCount
in interfaceILcdGXYEditor
- Returns:
- either 2, 3 or 5 depending on the
creation mode
. - See Also:
-
setModelModelTransformationClass
Sets the transformation class that should be used when snapping to points that are defined in a different reference than the reference of this painter's object. The transformation will be instantiated and setup to transform points from the model of the snap layer to points in the model which contains the current object of this painter. The default value is the
TLcdGeoReference2GeoReference
class.This property allows the painter to snap to points defined in a different model reference.
Instances of this specified class should implement
ILcdModelModelTransformation
, otherwise the snapping functionality to different model references will not work.- Parameters:
aModel2ModelTransformationClass
- the transformation used as described above.
-
acceptSnapTarget
Determines whether a snap target should be accepted or not. A snap target is accepted if the following conditions are met:
- The current object is an
ILcd2DEditableArc
. - The snap target is an
ILcdPoint
whose coordinates are expressed in the same coordinate system as the current objects coordinate system or the model to model transformation can transform the point to a point in the objects coordinate system. - The snap object is not the center point of the current object. If this is wanted, override the function and return true if the snap object is the center point.
- One of the axis corner points or the center point is touched or when both minor and
major axis are
0
. This enables snapping when creating an arc.
- Specified by:
acceptSnapTarget
in interfaceILcdGXYEditor
- Parameters:
aGraphics
- the graphics on which the snap target should be checked.aGXYContext
- the context in which the snap target should be checked.- Returns:
true
if the above conditions are met,false
otherwise.- See Also:
- The current object is an
-
snapTarget
Returns the center point or one of the end points of the
ILcdArc
if it is touched. If no point was touched,null
will be returned. Note that the center point is only returned if the center point is drawn (seeisDrawCenter()
) or when snapping to invisible points is enabled (seeisSnapToInvisiblePoints()
).- Specified by:
snapTarget
in interfaceILcdGXYPainter
- Overrides:
snapTarget
in classALcdGXYPainter
- Parameters:
aGraphics
- the graphics on which is worked.aGXYContext
- the context of the snapping.- Returns:
- the center point or one of the end points of the
ILcdArc
if it is touched,null
otherwise. - See Also:
-
supportSnap
This implementation supports snapping, always returnstrue
.- Specified by:
supportSnap
in interfaceILcdGXYPainter
- Overrides:
supportSnap
in classALcdGXYPainter
- Parameters:
aGraphics
- the graphics on which is worked.aGXYContext
- the context of the snapping.- Returns:
true
.
-
anchorPointSFCT
public void anchorPointSFCT(Graphics aGraphics, int aRenderMode, ILcdGXYContext aGXYContext, Point aPointSFCT) throws TLcdNoBoundsException Moves the specified
aPointSFCT
to the anchor point of theILcdArc
object.- Specified by:
anchorPointSFCT
in interfaceILcdGXYPainter
- Overrides:
anchorPointSFCT
in classALcdGXYPainter
- Parameters:
aGraphics
- The graphics to paint the object on.aRenderMode
- The mode to render the object in.aGXYContext
- The context to render the object in.aPointSFCT
- The point that needs to be updated.- Throws:
TLcdNoBoundsException
- if theILcdArc
object doesn't have a valid anchor point, e.g. if it is always invisible in the current projection.- See Also:
-
clone
Description copied from interface:ILcdCloneable
Makes
When for example extending fromObject.clone()
public.java.lang.Object
, it can be implemented like this:public Object clone() { try { return super.clone(); } catch ( CloneNotSupportedException e ) { // Cannot happen: extends from Object and implements Cloneable (see also Object.clone) throw new RuntimeException( e ); } }
- Specified by:
clone
in interfaceILcdCloneable
- Specified by:
clone
in interfaceILcdGXYEditorProvider
- Specified by:
clone
in interfaceILcdGXYPainterProvider
- Overrides:
clone
in classALcdGXYPainter
- Returns:
- a clone of this painter provider.
- See Also:
-