Package com.luciad.imaging.operator
Class TLcdPixelTransformOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdPixelTransformOp
Adjusts the pixel values of an image by applying an affine transform defined by a 2-dimensional matrix and a 1-dimensional
offset vector.
This operation is equivalent to the following pseudo-code:
(Y, Cb and Cr are visualized here as red, green and blue for illustration purposes)
pixelTransform(in_value) {
normalized_in_value = normalize(in_value)
normalized_out_value = matrix * normalized_in_value + offsets
return denormalize(normalized_out_value)
}
The normalization range is defined by the ALcdBandSemantics
of the image.
This operator for example allows scaling pixel values by using a diagonal matrix. For example, increasing the red channel of an RGB image can be done using the matrix {2, 0, 0, 0, 1, 0, 0, 0, 1} and offsets vector {0, 0, 0}. The operator also allows mixing multiple bands together. Converting for example an RGB image to a full-range YCbCr image can be done using the matrix {0.299, 0.587, 0.114, -0.169, -0.331, 0.5, 0.5, -0.419, -0.081} and the offsets vector {0, 0.5, 0.5}.
Example
Convert from RGB to YCbCr:
// Using the static method:
ALcdImage inputImage = ...;
ALcdImage outputImage = TLcdPixelTransformOp.pixelTransform(
inputImage,
new double[]{0.299, 0.587, 0.114, -0.169, -0.331, 0.5, 0.5, -0.419, -0.081},
new double[]{0.0, 0.5, 0.5}
);
// Using a data object:
ALcdImage inputImage = ...;
TLcdPixelTransformOp op = new TLcdPixelTransformOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdPixelTransformOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdPixelTransformOp.MATRIX, new double[]{0.299, 0.587, 0.114, -0.169, -0.331, 0.5, 0.5, -0.419, -0.081});
params.setValue(TLcdPixelTransformOp.OFFSETS, new double[]{0.0, 0.5, 0.5});
ALcdImage outputImage = op.apply(params);
Input
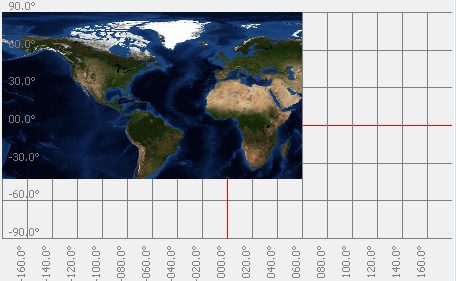
Output
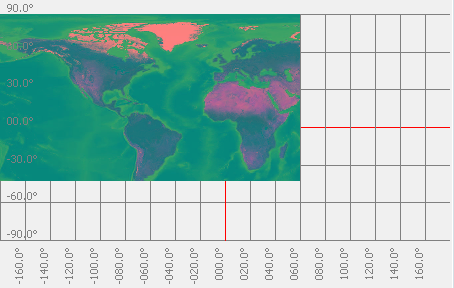
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
The input image.static final TLcdDataProperty
The matrix to apply to the input pixel values.static final String
Name of the operator.static final TLcdDataProperty
The offset to apply to the input values transformed with the matrixstatic final TLcdDataType
Input data type of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdImage
pixelTransform
(ALcdImage aSource, double[] aMatrix, double[] aOffsets) Creates a pixel transform operator for a given input image.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
MATRIX
The matrix to apply to the input pixel values. -
OFFSETS
The offset to apply to the input values transformed with the matrix Note that this offset applies to normalized input values. So for example to add 1 to each pixel of an image with 8-bit unsigned integers you would use an offset of1.0 / 255.0
. -
PIXEL_TRANSFORM_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdPixelTransformOp
public TLcdPixelTransformOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
pixelTransform
Creates a pixel transform operator for a given input image. The operator takes arrays of representing the matrix (in row-major order) and offsets vectors. The length of aOffsets should be equal to the number of bands in the image. The length of aMatrix should be the square of the number of bands in the image.- Parameters:
aSource
- the image to be processedaMatrix
- the matrix to be applied to each of the images pixelsaOffsets
- the offsets to be added- Returns:
- an image with the same number of bands as the input image, where each pixel is the result of the affine transform defined by the matrix and offsets vector.
-