Package com.luciad.imaging.operator
Class TLcdSwipeOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdSwipeOp
Composes two images by taking the left part of the input image and combine it with
the right part of the second image with respect to a swipe line.
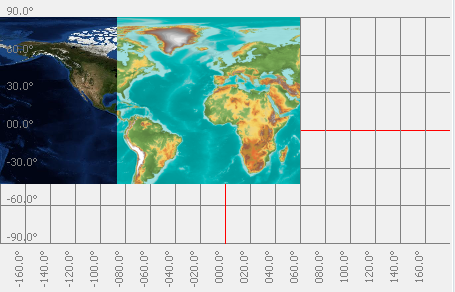
- Swipe operation can be used only with ALcdBasicImage input images
- If one of the input pixels of the input image has a
"no data" value
, the result for the current pixel is also "no data".
Example
// Using the static method:
ALcdBasicImage inputImage = ...;
ALcdBasicImage secondImage = ...;
ALcdBasicImage outputImage = TLcdSwipeOp.swipe(inputImage, secondImage, 350);
// Using a data object:
ALcdBasicImage inputImage = ...;
ALcdBasicImage secondImage = ...;
TLcdSwipeOp op = new TLcdSwipeOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdSwipeOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdSwipeOp.SECOND_IMAGE, secondImage);
params.setValue(TLcdSwipeOp.SWIPE_DISTANCE, 350);
ALcdBasicImage outputImage = op.apply(params);
Input
![]() |
|
![]() |
Output
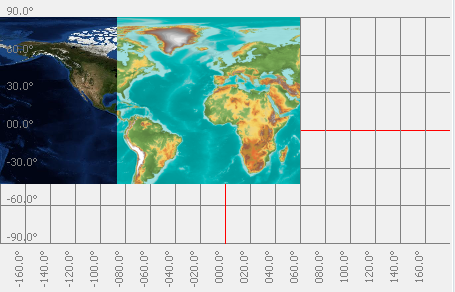
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
The input image.static final String
Name of the operator.static final TLcdDataProperty
The image to overlay on top of the input image.static final TLcdDataProperty
Distance (in pixels) at which to create a swipe line.static final TLcdDataType
Input data type of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdBasicImage
swipe
(ALcdBasicImage aInputImage, ALcdBasicImage aSecondImage, int aSwipeDistance) Creates a swipe operator for a given input imageMethods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
SECOND_IMAGE
The image to overlay on top of the input image. -
SWIPE_DISTANCE
Distance (in pixels) at which to create a swipe line. -
SWIPE_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdSwipeOp
public TLcdSwipeOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
swipe
public static ALcdBasicImage swipe(ALcdBasicImage aInputImage, ALcdBasicImage aSecondImage, int aSwipeDistance) Creates a swipe operator for a given input image- Parameters:
aInputImage
- the first image to be processedaSecondImage
- the second image to be processedaSwipeDistance
- x coordinate of the swipe line- Returns:
- the swipe operator
-