Package com.luciad.imaging.operator
Class TLcdResizeOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdResizeOp
Resizes an image, changing its resolution. The resulting resolution is the rounded result of
the scales multiplied with the input resolution.
The bounds of the image will remain unchanged.
Example
Input
Output
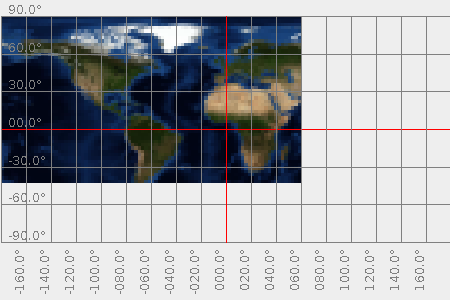
Example 
// Using the static method:
ALcdImage inputImage = ...;
ALcdImage outputImage = TLcdResizeOp.resize(inputImage, 0.1, 0.1);
// Using a data object:
ALcdImage inputImage = ...;
TLcdResizeOp op = new TLcdResizeOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdResizeOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdResizeOp.SCALE_X, 0.1);
params.setValue(TLcdResizeOp.SCALE_Y, 0.1);
ALcdImage outputImage = op.apply(params);
Input 
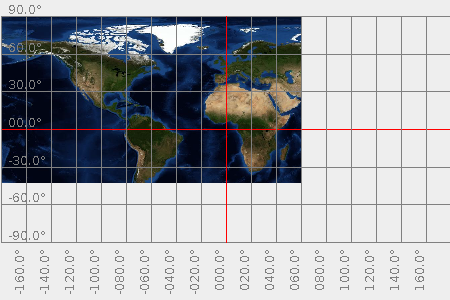
Output 
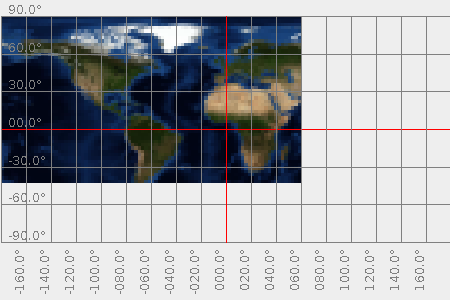
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
The input image.static final String
Name of the operator.static final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The scale factor in the x direction.static final TLcdDataProperty
The scale factor in the y direction.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
Constructor Details
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
resize
Creates a resize operator for a given input image. The operator takes a scale factor for x and y as input.- Parameters:
aSource
- the image to be processedaScaleX
- the scale factor in the x directionaScaleY
- the scale factor in the y direction- Returns:
- the resize operator
-