Package com.luciad.imaging.operator
Class TLcdPixelRescaleOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdPixelRescaleOp
Adjusts the pixel values of an image by applying a scale and offset.
Example
Input
Output

This operation is equivalent to the following pseudo-code:
pixelRescale(in_value) {
normalized_in_value = normalize(in_value)
normalized_out_value = normalized_in_value * scale + offset
return denormalize(normalized_out_value)
}
The normalization range is defined by the ALcdBandSemantics
of the image.
When rescaling a pixel with a
"no data" value
, the
result is also "no data".
You can find more details about the image processing model here
.
Example 
// Using the static method:
ALcdImage inputImage = ...;
ALcdImage outputImage = TLcdPixelRescaleOp.pixelRescale(
inputImage,
new double[] { 1.0, 0.8, 0.2 },
new double[] { 0.07, 0.05, 0.0 }
);
// Using a data object:
ALcdImage inputImage = ...;
TLcdPixelRescaleOp op = new TLcdPixelRescaleOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdPixelRescaleOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdPixelRescaleOp.SCALES, new double[] { 1.0, 0.8, 0.2 });
params.setValue(TLcdPixelRescaleOp.OFFSETS, new double[] { 0.07, 0.05, 0.0 });
ALcdImage outputImage = op.apply(params);
Input 
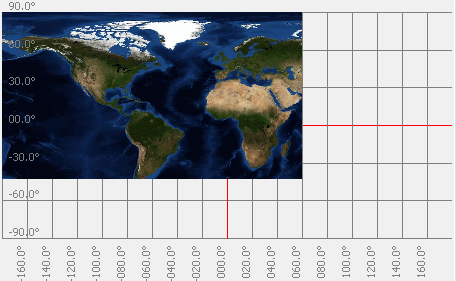
Output 

- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
The input image.static final String
Name of the operator.static final TLcdDataProperty
The offset to apply to the scaled input image values.static final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The scale to apply to the input image values.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdImage
pixelRescale
(ALcdImage aSource, double[] aScales, double[] aOffsets) Creates a pixel rescale operator for a given input image.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
SCALES
The scale to apply to the input image values. -
OFFSETS
The offset to apply to the scaled input image values.Note that this scale applies to normalized input values. So for example to add 1 to each pixel of a color image with 8-bit unsigned integers you would use an offset of
1.0 / 255.0
. -
PIXEL_RESCALE_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
pixelRescale
Creates a pixel rescale operator for a given input image. The operator takes arrays of scale factors and offsets as input, the length of which should be equal to the number of bands in the image.- Parameters:
aSource
- the image to be processedaScales
- the scales to applied to each of the image's color bandsaOffsets
- the offsets to be applied to each of the image's color bands- Returns:
- the pixel rescale operator
-