Package com.luciad.imaging.operator
Class TLcdPixelReplaceOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdPixelReplaceOp
Replaces every pixel in an image which matches a given value with another value.
A tolerance parameter allows the matching to be made more or less strict. A
typical use case for this operator is to remove borders with a solid color around
the edges of an image, by replacing this color with a fully transparent one.
Example
Input
Output
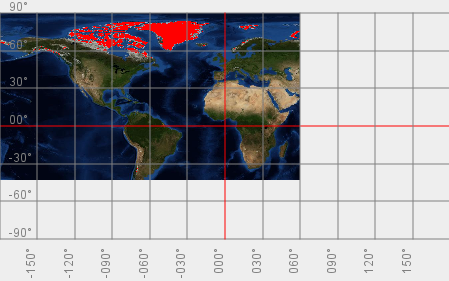
Example 
// Using the static method:
double[] srcColor = ...;
double[] dstColor = ...;
ALcdImage inputImage = ...;
ALcdImage outputImage = TLcdPixelReplaceOp.pixelReplace(inputImage, srcColor, dstColor, 0.01);
// Using a data object:
double[] srcColor = ...;
double[] dstColor = ...;
ALcdImage inputImage = ...;
TLcdPixelReplaceOp op = new TLcdPixelReplaceOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdPixelReplaceOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdPixelReplaceOp.SOURCE_COLOR, srcColor);
params.setValue(TLcdPixelReplaceOp.DESTINATION_COLOR, dstColor);
params.setValue(TLcdPixelReplaceOp.TOLERANCE, 0.01);
ALcdImage outputImage = op.apply(params);
Input 
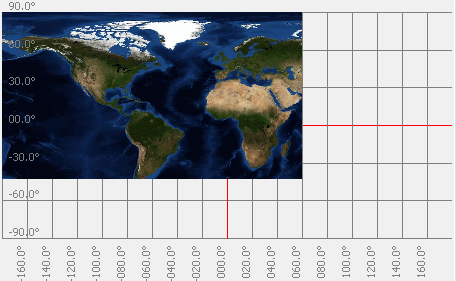
Output 
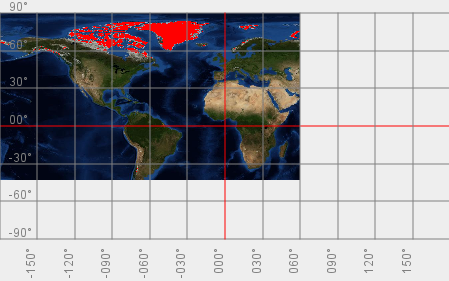
- Since:
- 2024.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
Value by which the source value will be replaced.static final TLcdDataProperty
The input image.static final String
Name of the operator.static final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
Pixel value to be replaced.static final TLcdDataProperty
Tolerance to be used for detecting the source value in the input image.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdImage
pixelReplace
(ALcdImage aSource, double[] aSourceValue, double[] aDestinationValue, double aTolerance) Perform a pixel replace operation on an image.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
SOURCE_VALUE
Pixel value to be replaced. -
DESTINATION_VALUE
Value by which the source value will be replaced. -
TOLERANCE
Tolerance to be used for detecting the source value in the input image. -
PIXEL_REPLACE_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
pixelReplace
public static ALcdImage pixelReplace(ALcdImage aSource, double[] aSourceValue, double[] aDestinationValue, double aTolerance) Perform a pixel replace operation on an image. The lengths of the source and destination value parameters must match the number of bands in the image. A pixel is considered to match the source value if the difference between each of its components and the corresponding source value component is smaller thanaTolerance
(i.e. ifabs(pixelValue[i] - aSourceValue[i]) < aTolerance
for all bands in the image).- Parameters:
aSource
- the input image.aSourceValue
- the pixel value to be replacedaDestinationValue
- the value with which to replace the source valueaTolerance
- the tolerance used for matching the source value in the input image- Returns:
- an image equivalent to the input, with every occurrence of the source value replaced with the destination value
-