Package com.luciad.imaging.operator
Class TLcdMedianOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdMedianOp
Applies a median filter to an image.
Example
Input
Output

The median filter computes for each pixel the median in a small area surrounding it. The median is the value that is
'in the middle' when the values would put into a sorted list. For example the median of [3, 5, 2, 1, 4]
is
3
: 2 values are smaller ([1, 2]
) and 2 values are larger ([4, 5]
).
If one of the input pixels has a "no data" value
, the
result is also "no data".
This operator expects kernels with odd dimensions (e.g., 3x3). Otherwise an IllegalArgumentException
will be thrown.
Example 
// Using the static method:
ALcdImage inputImage = ...;
ALcdImage outputImage = TLcdMedianOp.median(inputImage, 3, 3);
// Using a data object:
ALcdImage inputImage = ...;
TLcdMedianOp op = new TLcdMedianOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdMedianOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdMedianOp.MASK_WIDTH, 3);
params.setValue(TLcdMedianOp.MASK_HEIGHT, 3);
outputImage = op.apply(params);
Input 
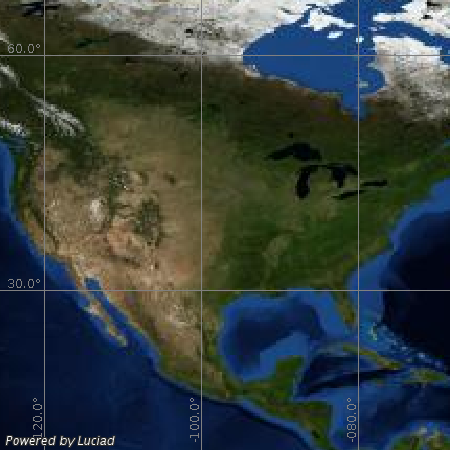
Output 

- Since:
- 2015.1
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
The input image.static final TLcdDataProperty
Height of the median filter.static final TLcdDataProperty
Width of the median filter.static final TLcdDataType
Input data type of the operator.static final String
Name of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
Constructor Details
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
median
Applies a median filter to the given image.- Parameters:
aImage
- the image to be medianaMaskWidth
- the width of the median filteraMaskHeight
- the height of the median filter- Returns:
- the median image
-