Package com.luciad.imaging.operator
Class TLcdIndexLookupOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdIndexLookupOp
Performs a color lookup using a single-band image.
Example
Input
Output
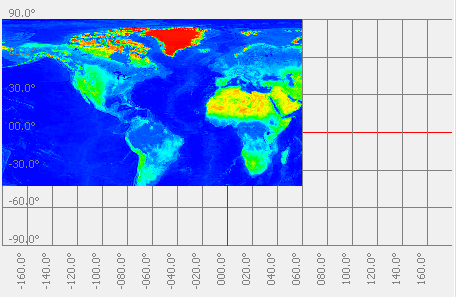
The TLcdLookupTable
specified must satisfy the following constraints:
- 1-dimensional
- 4 components per pixel, red/green/blue/alpha
The range of values that is mapped to the lookup table is determined as follows:
- The range in [
MIN_VALUE
,MAX_VALUE
] if they are specified. - The range in [
ALcdBandSemantics.getMinValue()
,ALcdBandSemantics.getMaxValue()
] if they are specified. - The full normalized range of the band otherwise.
Example 
// Define the table colors
// With interpolation enabled, a smooth gradient is automatically created
byte[] lookupColors = new byte[]{ 0, 0, (byte) 255, (byte) 255, // blue
0, (byte) 255, (byte) 255, (byte) 255,
0, (byte) 255, 0, (byte) 255, // green
(byte) 255, (byte) 255, 0, (byte) 255,
(byte) 255, 0, 0, (byte) 255}; // red
TLcdLookupTable indexLookupTable = TLcdLookupTable.newBuilder()
.dimensions(5)
.componentCount(4)
.data(ByteBuffer.wrap(lookupColors))
.build();
//use a chain. First bandSelect then indexLookUp
ALcdImageOperatorChain chain = ALcdImageOperatorChain.newBuilder()
.bandSelect(new int[]{0})
.indexLookup(indexLookupTable, new double[]{0.0, 0.0, 0.0, 0.0})
.build();
ALcdImage outputImage = chain.apply(inputImage);
Input 
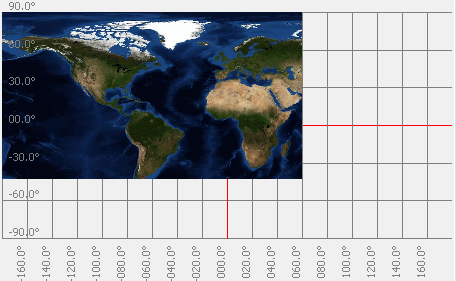
Output 
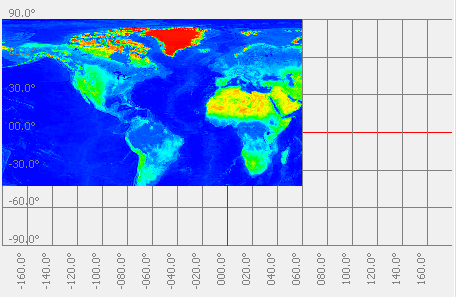
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The input image.static final TLcdDataProperty
The lookup table to use.static final String
Name of the operator.static final TLcdDataProperty
The color to set invalid pixels to.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdImage
indexLookup
(ALcdImage aImage, TLcdLookupTable aLUT) Perform a color lookup operation.static ALcdImage
indexLookup
(ALcdImage aSource, TLcdLookupTable aLUT, double[] aNaNColor) Perform a color lookup operation.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
Constructor Details
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
indexLookup
Perform a color lookup operation.- Parameters:
aImage
- the input image.aLUT
- the lookup table to use.- Returns:
- an image equivalent to the input, with every value replaced by its corresponding color in the lookup table.
-
indexLookup
Perform a color lookup operation.- Parameters:
aSource
- the input image.aLUT
- the lookup table to use.aNaNColor
- color to use for pixels that have an invalid or no value.- Returns:
- an image equivalent to the input, with every value replaced by its corresponding color in the lookup table.
-