Class TLcdConvolveOp
Convolution of an image with a kernel is an operation that computes for each pixel a weighted sum of that pixel and
its surrounding pixels. A convolution filter differs from the straight weighting in such a way that in convolution
the weight for a pixel at offset (dx, dy)
from the center pixel is weighted by the
weight at index (-dx,-dy)
in the kernel. In other words if the kernel is rotated by 180 degrees and placed on
top of the central pixel then each pixel lines up with it kernel weight. Note that the rotation has no effect in
case of a symmetric kernel.
For example the 2D convolution of a pixel with a kernel of 3x3:
double[] kernel = {+1, -2, +3,
-4, +5, -6,
+7, -8, +9};
// Each pixel is computed as follows:
out(column, row) = 0;
for (int dy=-1; dy<=1; dy++) {
for (int dx=-1; dx<=1; dx++) {
int index = 1-dx + (1-dy)*3; // where 3 is the width of the kernel (number of columns)
out(column, row)+=kernel[index]*in(column+dx, row+dy);
}
}
Note the use of -dx and -dy in the index computation, needed to perform the 180 degree rotation.
If one of the input pixels of the convolution kernel has a
"no data" value
, the
result of the kernel for the current pixel is also "no data".
This operator expects kernels with odd dimensions (e.g., 3x3). Otherwise an IllegalArgumentException
will be thrown.
Example 
// Using the static method:
ALcdImage inputImage = ...;
double[] kernel = new double[] {
-1.0f, -1.0f, -1.0f,
-1.0f, 9.0f, -1.0f,
-1.0f, -1.0f, -1.0f
};
ALcdImage outputImage = TLcdConvolveOp.convolve(inputImage, kernel, 3, 3);
// Using a data object:
ALcdImage inputImage = ...;
double[] kernel = new double[] {
-1.0f, -1.0f, -1.0f,
-1.0f, 9.0f, -1.0f,
-1.0f, -1.0f, -1.0f
};
TLcdConvolveOp op = new TLcdConvolveOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdConvolveOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdConvolveOp.KERNEL, kernel);
params.setValue(TLcdConvolveOp.KERNEL_WIDTH, 3);
params.setValue(TLcdConvolveOp.KERNEL_HEIGHT, 3);
outputImage = op.apply(params);
Input 
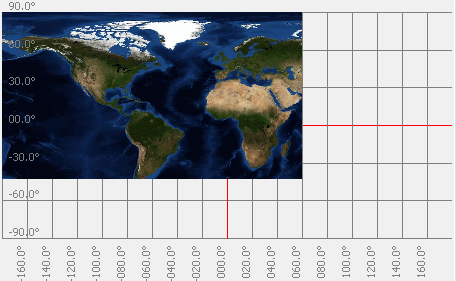
Output 
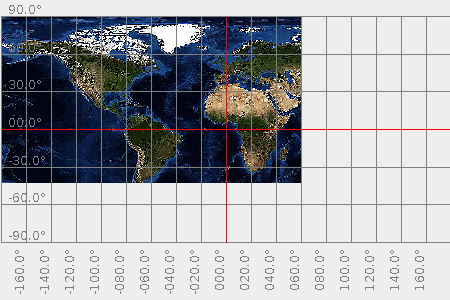
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The input image.static final TLcdDataProperty
The convolution kernel in row-major order.static final TLcdDataProperty
Height of the convolution kernel.static final TLcdDataProperty
Width of the convolution kernel.static final String
Name of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
Constructor Details
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
convolve
public static ALcdImage convolve(ALcdImage aImage, double[] aKernel, int aKernelWidth, int aKernelHeight) Applies a convolution kernel to the given image. The convolution kernel is supplied as an array of doubles in row-major order. The length of the array must equalaKernelWidth
xaKernelHeight
.- Parameters:
aImage
- the image to be convolvedaKernel
- the convolution kernel, in row-major orderaKernelWidth
- the width of the kernelaKernelHeight
- the height of the kernel- Returns:
- the convolved image
-