Package com.luciad.imaging.operator
Class TLcdCompositeOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdCompositeOp
Composes two ALcdBasicImages. This operator results in an image that covers the (spatial) union
of the input images.
The composite contains the pixels of the first image without any change. However if the second image may be
re-sampled if its pixel grid does not align with that of the first image (ex. due to a different pixel size).
Note:
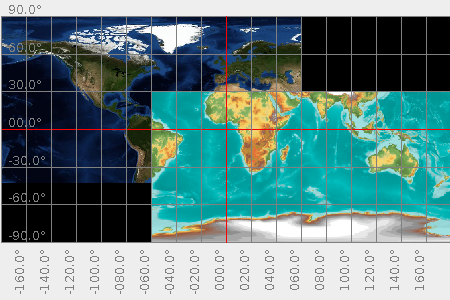
- Compositing can result in an ALcdBasicImage with a different spatial bounds from the input images.
- If one of the input pixels of the input image has a
"no data" value
, the result for the current pixel is also "no data".
Example
// Using the static method:
ALcdBasicImage inputImage = ...;
ALcdBasicImage secondImage = ...;
ALcdBasicImage outputImage = TLcdCompositeOp.composite(inputImage, secondImage);
// Using a data object:
ALcdBasicImage inputImage = ...;
ALcdBasicImage secondImage = ...;
TLcdCompositeOp op = new TLcdCompositeOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdCompositeOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdCompositeOp.SECOND_IMAGE, secondImage);
ALcdBasicImage outputImage = op.apply(params);
Input
![]() | + | ![]() |
Output
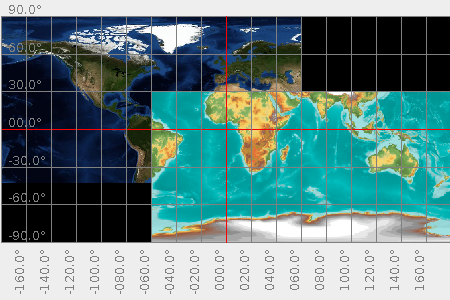
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The input image.static final String
Name of the operator.static final TLcdDataProperty
Image to overlay on top of the input image.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdBasicImage
composite
(ALcdBasicImage aInputImage, ALcdBasicImage aSecondImage) Compose two images.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
SECOND_IMAGE
Image to overlay on top of the input image. -
COMPOSITE_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdCompositeOp
public TLcdCompositeOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
composite
Compose two images.- Parameters:
aInputImage
- the input image.aSecondImage
- image to overlay on top of the input.- Returns:
- the input image with the second image on top of it.
-