Package com.luciad.imaging.operator
Class TLcdBinaryOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdBinaryOp
Performs a binary operation on two input images. Supported operations are defined
by TLcdBinaryOp.Operation.
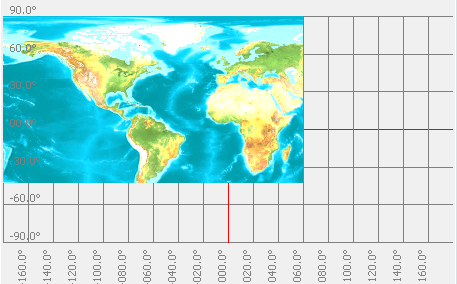
Example
// Using the static method:
ALcdBasicImage inputImage = ...;
ALcdBasicImage secondImage = ...;
TLcdBinaryOp.Operation binaryOperation = TLcdBinaryOp.Operation.ADD;
ALcdBasicImage outputImage = TLcdBinaryOp.binaryOp(inputImage, secondImage, binaryOperation);
// Using a data object:
ALcdBasicImage inputImage = ...;
ALcdBasicImage secondImage = ...;
TLcdBinaryOp op = new TLcdBinaryOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdBinaryOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdBinaryOp.SECOND_IMAGE, secondImage);
params.setValue(TLcdBinaryOp.OPERATION, binaryOperation);
ALcdBasicImage outputImage = op.apply(params);
Convenience shortcut methods are also available for every supported operation:
outputImage = TLcdBinaryOp.add(inputImage,secondImage);
outputImage = TLcdBinaryOp.subtract(inputImage,secondImage);
outputImage = TLcdBinaryOp.multiply(inputImage,secondImage);
outputImage = TLcdBinaryOp.divide(inputImage,secondImage);
outputImage = TLcdBinaryOp.max(inputImage,secondImage);
outputImage = TLcdBinaryOp.min(inputImage,secondImage);
Input
![]() | + | ![]() |
Output
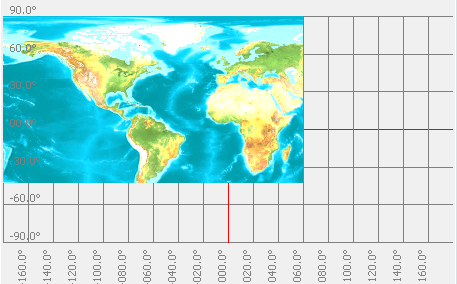
- Since:
- 2014.0
-
Nested Class Summary
Nested ClassesNested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The input image.static final String
Name of the operator.static final TLcdDataProperty
Binary operation to apply.static final TLcdDataProperty
The second image to use in the binary operation.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionstatic ALcdBasicImage
add
(ALcdBasicImage aImage1, ALcdBasicImage aImage2) Add two images together.apply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdBasicImage
binaryOp
(ALcdBasicImage aImage1, ALcdBasicImage aImage2, TLcdBinaryOp.Operation aOperation) Applies a binary operation to two input images.static ALcdBasicImage
divide
(ALcdBasicImage aImage1, ALcdBasicImage aImage2) Divide two images.static ALcdBasicImage
max
(ALcdBasicImage aImage1, ALcdBasicImage aImage2) Take the maximum of two images.static ALcdBasicImage
min
(ALcdBasicImage aImage1, ALcdBasicImage aImage2) Take the minimum of two images.static ALcdBasicImage
multiply
(ALcdBasicImage aImage1, ALcdBasicImage aImage2) Multiply two images.static ALcdBasicImage
subtract
(ALcdBasicImage aImage1, ALcdBasicImage aImage2) Subtract two images from each other.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
SECOND_IMAGE
The second image to use in the binary operation. -
OPERATION
Binary operation to apply. -
BINARY_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdBinaryOp
public TLcdBinaryOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
binaryOp
public static ALcdBasicImage binaryOp(ALcdBasicImage aImage1, ALcdBasicImage aImage2, TLcdBinaryOp.Operation aOperation) Applies a binary operation to two input images.- Parameters:
aImage1
- first input image.aImage2
- second input image.aOperation
- operation to apply to the images.- Returns:
- the image resulting from applying the operation to the source images.
-
add
Add two images together.- Parameters:
aImage1
- first input image.aImage2
- second input image.- Returns:
- an image where every pixel is the sum of the input pixels.
-
subtract
Subtract two images from each other.- Parameters:
aImage1
- first input image.aImage2
- second input image.- Returns:
- an image where every pixel is the difference of the second and first input pixel.
-
multiply
Multiply two images.- Parameters:
aImage1
- first input image.aImage2
- second input image.- Returns:
- an image where every pixel is the product of the input pixels.
-
divide
Divide two images.- Parameters:
aImage1
- first input image.aImage2
- second input image.- Returns:
- an image where every pixel is the quotient of the input pixels.
-
min
Take the minimum of two images.- Parameters:
aImage1
- first input image.aImage2
- second input image.- Returns:
- an image where every pixel is minimum of the input pixels.
-
max
Take the maximum of two images.- Parameters:
aImage1
- first input image.aImage2
- second input image.- Returns:
- an image where every pixel is maximum of the input pixels.
-