Package com.luciad.imaging.operator
Class TLcdExpandOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdExpandOp
Expands an image.
Note: Expanding can affect the
spatial bounds of the ALcdBasicImage and should hence be used with care when working with
ALcdImageMosaic as the bounds of an individual ALcdBasicImage should not be altered.
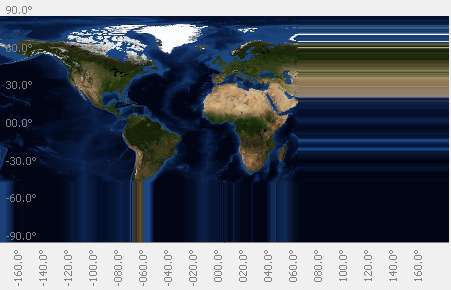
Example
// Using the static method:
ALcdBasicImage inputImage = ...;
ALcdImage outputImage = TLcdExpandOp.expand(inputImage, 0, 0, 1350, 675);
// Using a data object:
ALcdBasicImage inputImage = ...;
TLcdExpandOp op = new TLcdExpandOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdExpandOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdExpandOp.EXPAND_X, 0);
params.setValue(TLcdExpandOp.EXPAND_Y, 0);
params.setValue(TLcdExpandOp.EXPAND_WIDTH, 1350);
params.setValue(TLcdExpandOp.EXPAND_HEIGHT, 675);
ALcdImage outputImage = op.apply(params);
Input
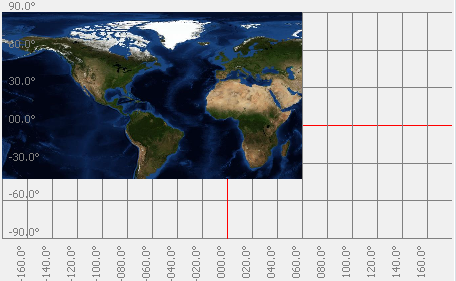
Output
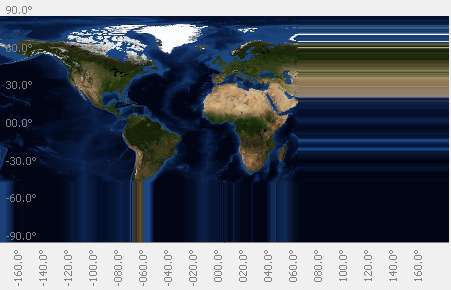
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
Height of the expanded region.static final TLcdDataProperty
Width of the expanded region.static final TLcdDataProperty
X coordinate of the expanded region.static final TLcdDataProperty
Y coordinate of the expanded region.static final TLcdDataProperty
The input image.static final String
Name of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
EXPAND_X
X coordinate of the expanded region. -
EXPAND_Y
Y coordinate of the expanded region. -
EXPAND_WIDTH
Width of the expanded region. -
EXPAND_HEIGHT
Height of the expanded region. -
EXPAND_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdExpandOp
public TLcdExpandOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
expand
public static ALcdImage expand(ALcdImage aInputImage, int aExpandX, int aExpandY, int aExpandWidth, int aExpandHeight) Creates a expand operator for a given input image- Parameters:
aInputImage
- the image to be expandedaExpandX
- x coordinate of the expanded regionaExpandY
- y coordinate of the expanded regionaExpandWidth
- width of the expanded regionaExpandHeight
- height of the expanded region- Returns:
- the expand operator
-