You developed a custom panel, and you want to show it as a bottom panel in the map-centric version of Lucy. You also want to add a button for showing and hiding your panel, just like the ones already available for the other bottom panels.
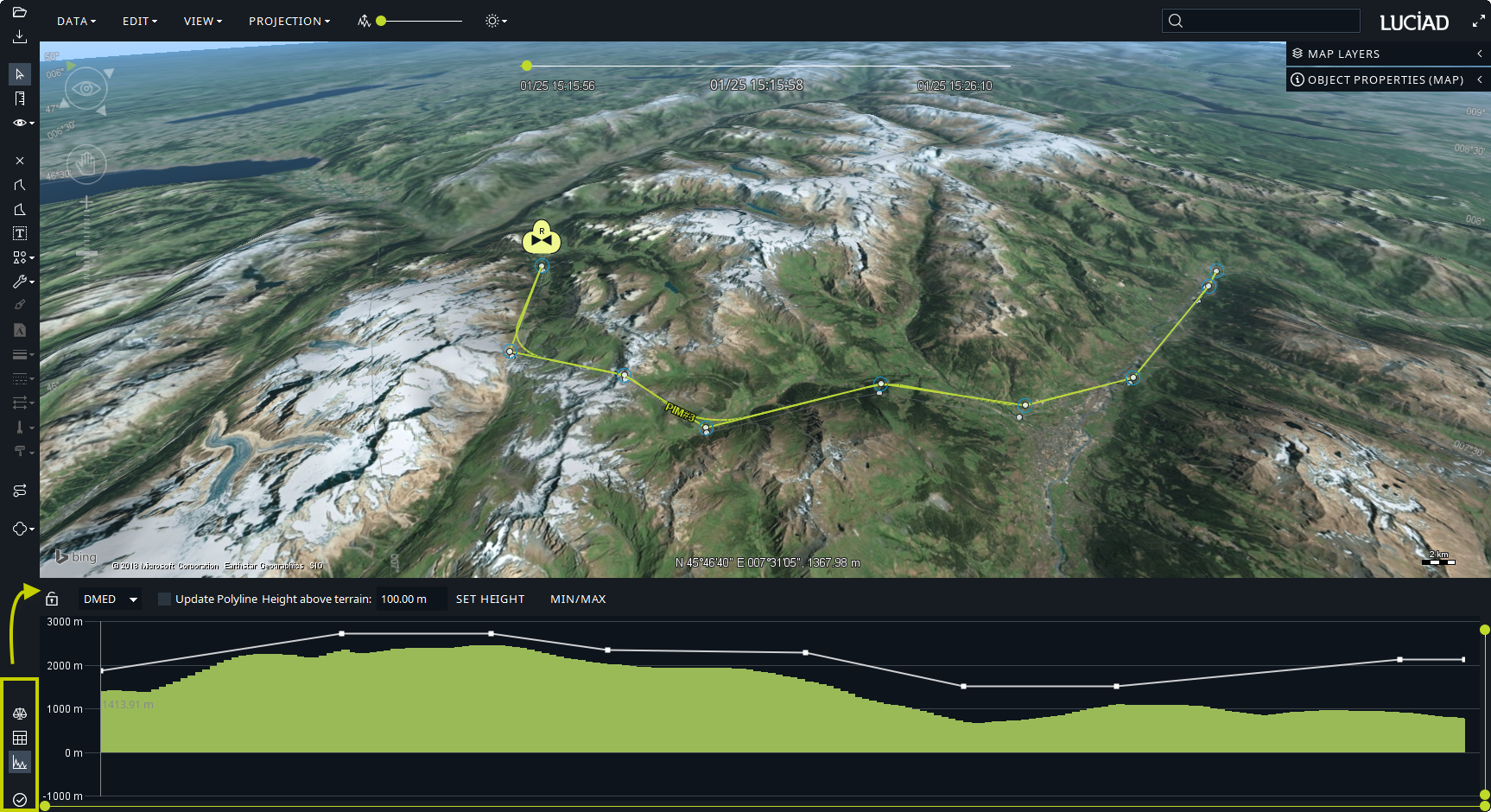
Why do it?
The map-centric Lucy UI has been designed to make all bottom panels available at all times, so it offers buttons on the side to show or hide those panels. You want to add your own panel to the collection of available panels.
How to do it?
-
Use an
ALcyApplicationPaneTool
instance to create yourILcyApplicationPane
, and plug it in from theplugInto
method of yourALcyPreferencesAddOn
. One of the main benefits of using the application pane tool is that you only need to create the contents of the application pane. Everything else, such as the creation of the application pane and workspace support, is taken care of by the tool:public class MyAddOn extends ALcyPreferencesAddOn{ private ALcyApplicationPaneTool fApplicationPaneTool; public MyAddOn() { super(ALcyTool.getLongPrefix(MyAddOn.class), ALcyTool.getShortPrefix(MyAddOn.class)); } @Override public void plugInto(ILcyLucyEnv aLucyEnv) { super.plugInto(aLucyEnv); fApplicationPaneTool = ALcyApplicationPaneTool.create(getPreferences(), getLongPrefix(), getShortPrefix(), this::createMyPanel); fApplicationPaneTool.plugInto(aLucyEnv); } private JComponent createMyPanel(){ return new JLabel("Custom content"); } @Override public void unplugFrom(ILcyLucyEnv aLucyEnv) { super.unplugFrom(aLucyEnv); fApplicationPaneTool.unplugFrom(aLucyEnv); } }
-
The configuration file of your add-on must:
-
Configure the bottom as the location for the application pane.
-
Ensure that the application pane is created when Lucy starts: make the corresponding active settable of the application pane tool active.
-
Configure the icon and the short description for the application pane. The icon will be used for the button in the side bar, and the short description will be the tooltip of the button
-
Remove the active settable from the UI. The map-centric front-end automatically adds a button in the side bar, so there is no need to have the active settable in the UI.
# These are the configuration settings for the ALcyApplicationPaneTool # Consult the class javadoc for an overview of all available configuration options # Activate the panel on start-up, don't show a menu item though MyAddOn.applicationPane.activeSettable.menuBar.item=Show my panel MyAddOn.applicationPane.activeSettable.menuBar.insert=false MyAddOn.applicationPane.activeSettable.menuBar.active=true # Configure name, location and icon # See the constants in ILcyApplicationPaneFactory for the possible values for location # In this case, 1 is the value of the HORIZONTAL_PANE constant MyAddOn.applicationPane.appTitle=My app title MyAddOn.applicationPane.location=1 MyAddOn.applicationPane.shortDescription=Tooltip for the button MyAddOn.applicationPane.smallIcon=icon
-
How to control which panel is visible at start-up?
In most cases, Lucy MapCentric loads a workspace at start-up. The front-end workspace codec saves and restores the available panels, their visibility, and their order.
Therefore, if you want to ensure that a certain panel is visible or hidden at start-up, the best approach is to make the desired changes through the UI and store this state in the workspace that you load on startup.