You have your own editable format, and you want to show the format-specific tool bar with all the shape creation actions in the side bar of Lucy MapCentric.
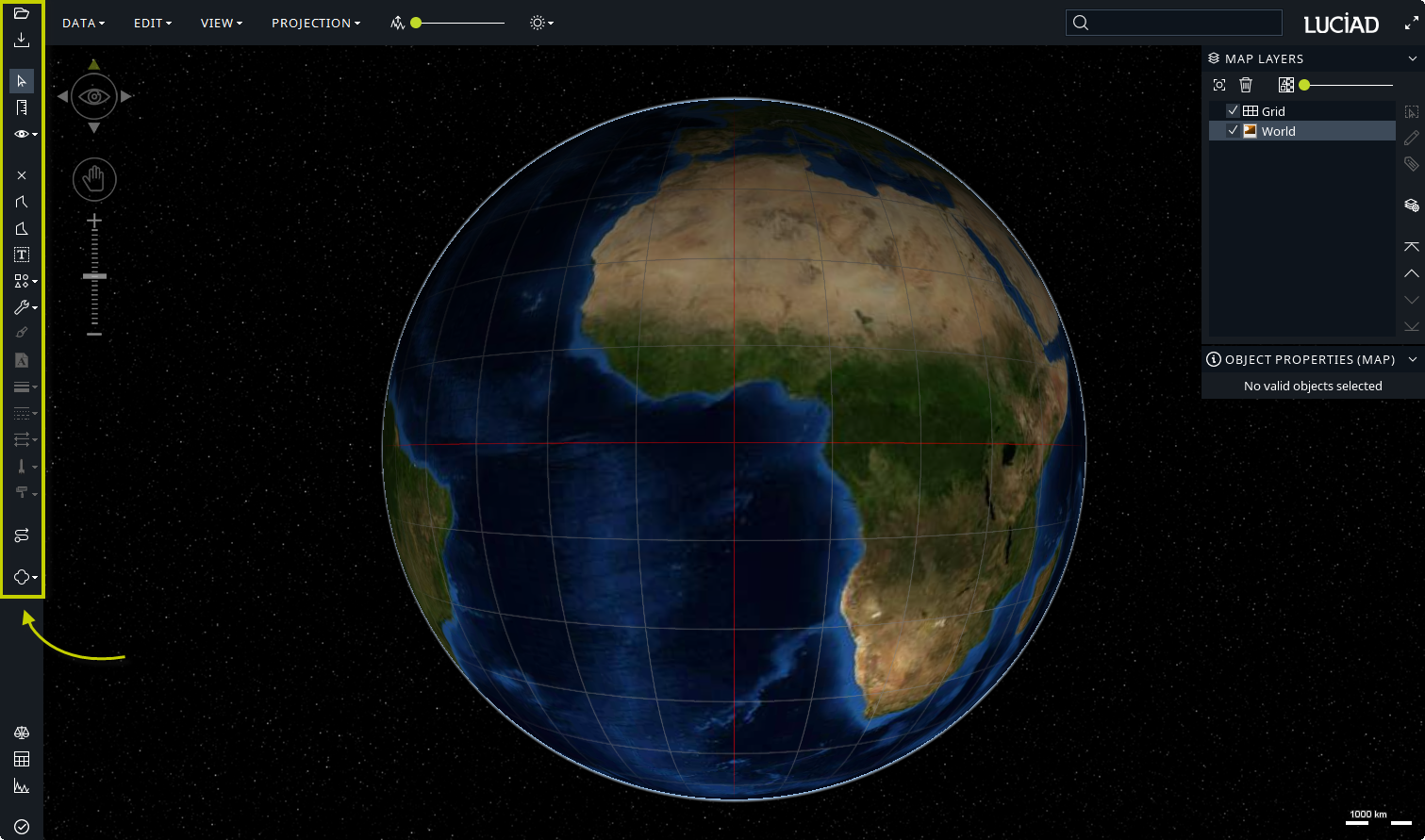
Why do it?
The Lucy MapCentric UI has been designed to place all shape creation actions in the left side bar. You want this side bar to show the actions for your custom format.
How to do it?
-
Register an
ALcyFormatBarFactory
to the Lucy back-end. Typically this is created in theALcyLspFormat.createFormatBarFactory
method. -
Use a
TLcyToolBar
as return value forALcyFormatBar.getComponent
, and make sure theTLcyToolBar
is a configured action bar by callingTLcyActionBarUtil.setupAsConfiguredActionBar
:final class MyCustomFormatBar extends ALcyFormatBar { private final TLcyToolBar fToolBar = new TLcyToolBar(); private final ILcdAction fAction; public MyCustomFormatBar(TLcyLspMapComponent aLspMapComponent, ALcyProperties aProperties, String aShortPrefix, ILcyLucyEnv aLucyEnv) { //Make the tool bar a configured action bar TLcyActionBarManager actionBarManager = aLucyEnv.getUserInterfaceManager().getActionBarManager(); String actionBarID = "myCustomFormatBar"; TLcyActionBarUtil.setupAsConfiguredActionBar(fToolBar, actionBarID, aLspMapComponent, aProperties, aShortPrefix, (JComponent) aLspMapComponent.getComponent(), actionBarManager); //Create your actions and active settables to include in the tool bar fAction = ...; fAction.putValue(TLcyActionBarUtil.ID_KEY, aShortPrefix + "myActionIdentifier"); //Insert the actions and active settables TLcyActionBarUtil.insertInConfiguredActionBar(fAction, aLspMapComponent, aProperties, actionBarManager); } @Override protected void updateForLayer(ILcdLayer aPreviousLayer, ILcdLayer aLayer) { //This method is called when a layer for this format bar is selected //Normally the actions present in this format bar operate on a layer, //and will have a setter for the layer //Use that setter to pass the new layer to the action fAction.setLayer(aLayer); } @Override public boolean canSetLayer(ILcdLayer aLayer) { //check whether the layer is supported by this format bar } @Override public Component getComponent() { return fToolBar.getComponent(); } }
The Supporting custom data in Lucy tutorials contain example format bar implementations.
-
Populate the
TLcyToolBar
with actions and active settables usingTLcyActionBarUtil#insertInConfiguredActionBars
, as illustrated above. -
Modify the configuration file of the
SideFormatBarAddOn
(samples/resources/samples/frontend/mapcentric/formatbar/SideBarFormatBarAddOn.cfg
), and add the name of your configured action bar to the propertySideBarFormatBarAddOn.formatBarNames
. In case of this example, the name is "myCustomFormatBar".