Package com.luciad.imaging.operator
Class TLcdCurvesOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdCurvesOp
Remaps the values in an image using curves which can be specified
by the user. The curves represent functions which map an input level to an
output level, and can be used to selectively brighten or darken parts of the
spectrum.
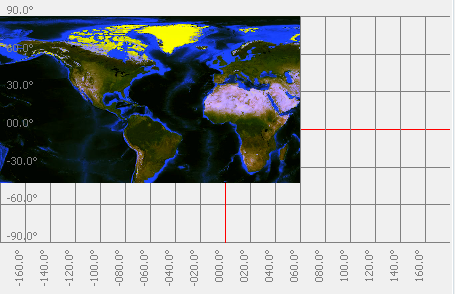
Example
// Using the static method:
ALcdImage inputImage = ...;
TLcdCurvesOp.CurveType curveType = TLcdCurvesOp.CurveType.CATMULL_ROM;
ILcdPoint[][] controlPoints = {{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.5, 0.5), new TLcdXYPoint(1, 1)},
{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.25, 0.25), new TLcdXYPoint(0.5, 0.5), new TLcdXYPoint(1, 1)},
{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.25, 0.1),
new TLcdXYPoint(0.5, 1), new TLcdXYPoint(0.75, 0.1), new TLcdXYPoint(1, 0)},
{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.5, 0.5), new TLcdXYPoint(1, 1)}};
ALcdImage outputImage = TLcdCurvesOp.curves(inputImage,controlPoints,curveType);
// Using a data object:
ALcdImage inputImage = ...;
TLcdCurvesOp op = new TLcdCurvesOp();
ILcdPoint[][] controlPoints = {{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.5, 0.5), new TLcdXYPoint(1, 1)},
{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.25, 0.25), new TLcdXYPoint(0.5, 0.5), new TLcdXYPoint(1, 1)},
{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.25, 0.1),
new TLcdXYPoint(0.5, 1), new TLcdXYPoint(0.75, 0.1), new TLcdXYPoint(1, 0)},
{new TLcdXYPoint(0, 0), new TLcdXYPoint(0.5, 0.5), new TLcdXYPoint(1, 1)}};
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdCurvesOp.INPUT_IMAGE,inputImage);
params.setValue(TLcdCurvesOp.CURVE_TYPE,TLcdCurvesOp.CurveType.CATMULL_ROM);
params.setValue(TLcdCurvesOp.CONTROL_POINTS,controlPoints);
ALcdImage outputImage = op.apply(params);
Input
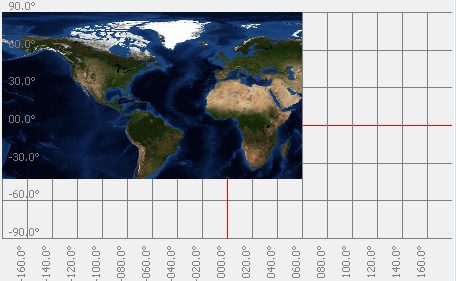
Output
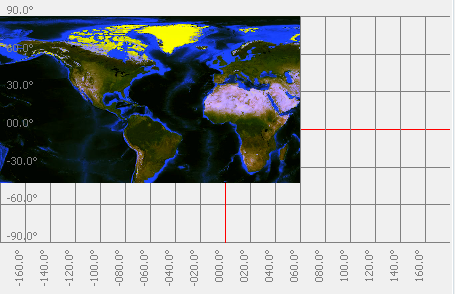
- Since:
- 2014.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
Defines the types of curves that can be used to interpolate between control points.Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataProperty
Control points for the curve.static final TLcdDataProperty
Type of the curve.static final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
The input image.static final String
Name of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdImage
curves
(ALcdImage aInputImage, ILcdPoint[][] aCurves, TLcdCurvesOp.CurveType aCurveType) Creates a curves operator for a given input image.Methods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
CONTROL_POINTS
Control points for the curve. -
CURVE_TYPE
Type of the curve. -
CURVES_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdCurvesOp
public TLcdCurvesOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
curves
public static ALcdImage curves(ALcdImage aInputImage, ILcdPoint[][] aCurves, TLcdCurvesOp.CurveType aCurveType) Creates a curves operator for a given input image. The operator takes a list of lists containing the control points. It is required that either one curve is defined or one curve per channel. If only one curve is defined, this curve will be used for all channels. If there are less curves than channels, the first curve will be used for all channels. The curve type can also be configured.- Parameters:
aInputImage
- the image to be processedaCurves
- the control points for each defined curveaCurveType
- the type of curve used to interpolate between the control points- Returns:
- the curves operator
-