Package com.luciad.imaging.operator
Class TLcdCropOp
java.lang.Object
com.luciad.imaging.operator.ALcdImageOperator
com.luciad.imaging.operator.TLcdCropOp
Crops an ALcdBasicImage by retaining a sub-rectangle of pixels.
Note: Cropping can affect the
spatial bounds of the ALcdBasicImage and should hence be used with care when working with
ALcdImageMosaic as the bounds of an individual ALcdBasicImage should not be altered. Use
TLcdExpandOp to expand the image back to its original size after cropping.
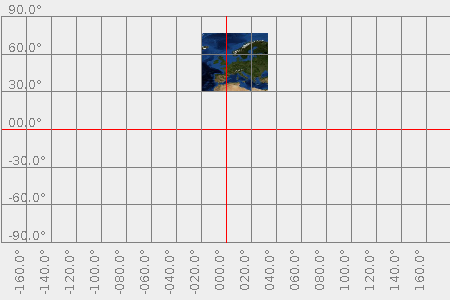
Example
// Using the static method:
ALcdBasicImage inputImage = ...;
ALcdImage outputImage = TLcdCropOp.crop(inputImage, 600, 50, 200, 175);
// Using a data object:
ALcdBasicImage inputImage = ...;
TLcdCropOp op = new TLcdCropOp();
ILcdDataObject params = op.getParameterDataType().newInstance();
params.setValue(TLcdCropOp.INPUT_IMAGE, inputImage);
params.setValue(TLcdCropOp.CROP_X, 600);
params.setValue(TLcdCropOp.CROP_Y, 50);
params.setValue(TLcdCropOp.CROP_WIDTH, 200);
params.setValue(TLcdCropOp.CROP_HEIGHT, 175);
ALcdImage outputImage = op.apply(params);
Input
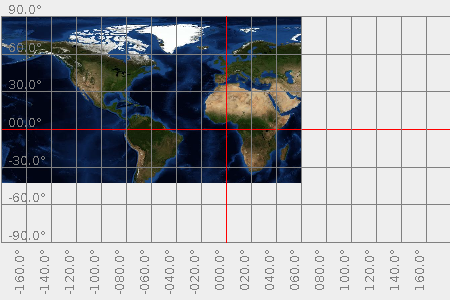
Output
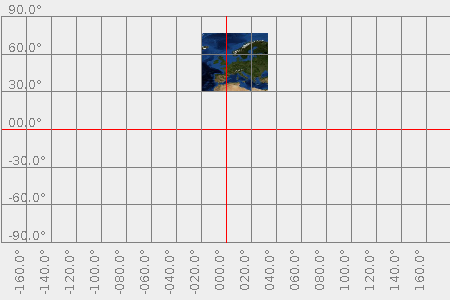
- Since:
- 2014.0
-
Nested Class Summary
Nested classes/interfaces inherited from class com.luciad.imaging.operator.ALcdImageOperator
ALcdImageOperator.ImageOperatorTypeBuilder
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final TLcdDataType
Input data type of the operator.static final TLcdDataProperty
Height of the cropped region.static final TLcdDataProperty
Width of the cropped region.static final TLcdDataProperty
X coordinate of the cropped region.static final TLcdDataProperty
Y coordinate of the cropped region.static final TLcdDataProperty
The input image.static final String
Name of the operator.Fields inherited from class com.luciad.imaging.operator.ALcdImageOperator
INPUT_IMAGE_NAME
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionapply
(ILcdDataObject aParameters) Applies this operator to the given input parameters.static ALcdImage
crop
(ALcdBasicImage aSource, int aCropX, int aCropY, int aCropWidth, int aCropHeight) Creates a crop operator for a given input imageMethods inherited from class com.luciad.imaging.operator.ALcdImageOperator
createTypeBuilder, createTypeBuilder, equals, getParameterDataType, hashCode, toString
-
Field Details
-
NAME
Name of the operator.- See Also:
-
INPUT_IMAGE
The input image. -
CROP_X
X coordinate of the cropped region. -
CROP_Y
Y coordinate of the cropped region. -
CROP_WIDTH
Width of the cropped region. -
CROP_HEIGHT
Height of the cropped region. -
CROP_FILTER_TYPE
Input data type of the operator.
-
-
Constructor Details
-
TLcdCropOp
public TLcdCropOp()Default constructor.
-
-
Method Details
-
apply
Description copied from class:ALcdImageOperator
Applies this operator to the given input parameters. The parameters are stored in a data object which must be of the type given by ALcdImageOperator.getParameterDataType().- Specified by:
apply
in classALcdImageOperator
- Parameters:
aParameters
- the parameters for the operator- Returns:
- the image produced by the operator
-
crop
public static ALcdImage crop(ALcdBasicImage aSource, int aCropX, int aCropY, int aCropWidth, int aCropHeight) Creates a crop operator for a given input image- Parameters:
aSource
- the image to be processedaCropX
- x coordinate of the cropped regionaCropY
- y coordinate of the cropped regionaCropWidth
- width of the cropped regionaCropHeight
- height of the cropped region- Returns:
- the crop operator
-