Painting symbology along lines with TLspComplexStrokedLineStyle
TLspComplexStrokedLineStyle
supports complex patterns that can be applied to a line.
This allows you to implement just about any complex line symbology.
The lightspeed.style.strokedline
sample shows how to use this style.
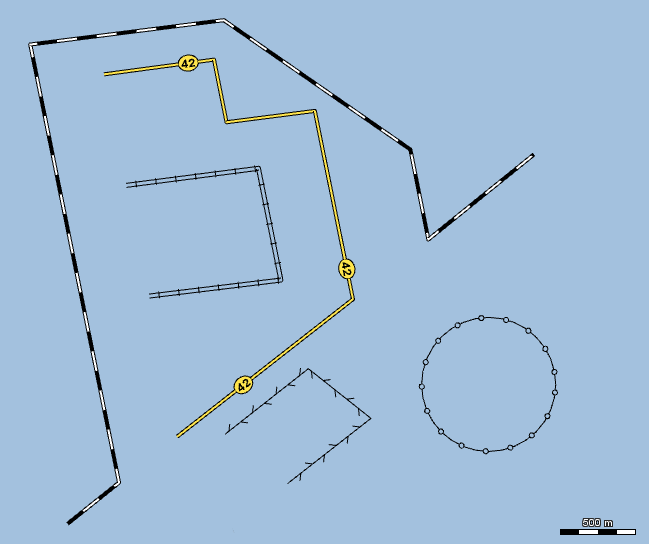
TLspComplexStrokedLineStyle
in sample lightspeed.style.strokedline
A TLspComplexStrokedLineStyle
consists of ALspComplexStroke
instances, along with a few properties that affect the visualization, such as the elevation mode and halo settings.
The following section shows how to create a TLspComplexStrokedLineStyle
.
Creating a TLspComplexStrokedLineStyle
A TLspComplexStrokedLineStyle
defines how a line is painted.
It combines a number of stroke patterns, that are for example repeated along the line, or added as a decoration at the start
or end.
A TLspComplexStrokedLineStyle
can be created using a Builder
.
This builder makes it possible to define decorations and regular repeated strokes patterns.
For example, the complex stroked line in the following figure contains an arrow decoration, a repeated block pattern and a
dash as fallback.
It is generated using the following code:
// Create an arrow stroke pattern ALspComplexStroke arrow = arrow().type(ALspComplexStroke.ArrowBuilder.ArrowType.PLAIN_OUTLINED).size(10).build(); // Create a block stroke pattern ALspComplexStroke block = append(line().length(0).offset0(0).offset1(8).build(), line().length(16).offset0(8).offset1(8).build(), line().length(0).offset0(8).offset1(-8).build(), line().length(16).offset0(-8).offset1(-8).build(), line().length(0).offset0(-8).offset1(0).build()); // Create a dash stroke pattern ALspComplexStroke dash = append(parallelLine().length(4).build(), gap(4)); // Create a stroked line using these stroke patterns TLspComplexStrokedLineStyle strokedLine = TLspComplexStrokedLineStyle.newBuilder() .decoration(1, arrow)// Add the arrow as decoration .regular(atomic(block))// Repeat the block stroke pattern along the line .fallback(dash)// Use the dash pattern as fallback .build();
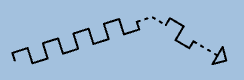
When a decoration or regular stroke pattern is omitted, for example when there is not enough space for it, or when it crosses a sharp corner, the fallback stroke is used.
Creating complex stroke patterns
Decorations, regular repeated patterns and fallback patterns can be created using complex stroke patterns. These patterns can be composed to form more complex strokes patterns. For example, the block pattern from previous example was created by using 5 appended line primitives.
The following figure shows many of the possible complex stroke pattern primitives.
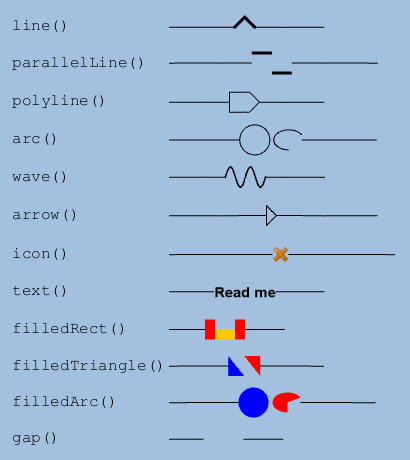
The following figure shows how primitives can be composed:
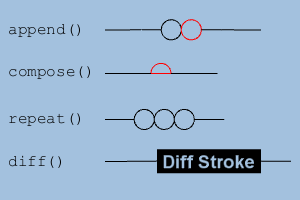